Functions in PowerShell can simplify the code, make it reusable, and allow for easy sharing among scripts. A critical aspect of functions is their parameters, which allow you to pass different types of data into your functions. Let’s dive deep into PowerShell functions with parameters.
PowerShell Function with Parameters
A PowerShell function can be defined using the function
keyword followed by a name, and within curly braces {}
, you can place your script block. Parameters are defined within a param
block. Here’s a simple example:
function Greet-User {
param (
[string]$Name
)
Write-Host "Hello, $Name!"
}
To call this function, you would simply pass a name like so:
Greet-User -Name "Alice"
This would output: Hello, Alice!
You can see the screenshot below for the output after I run the PowerShell script using VS code.
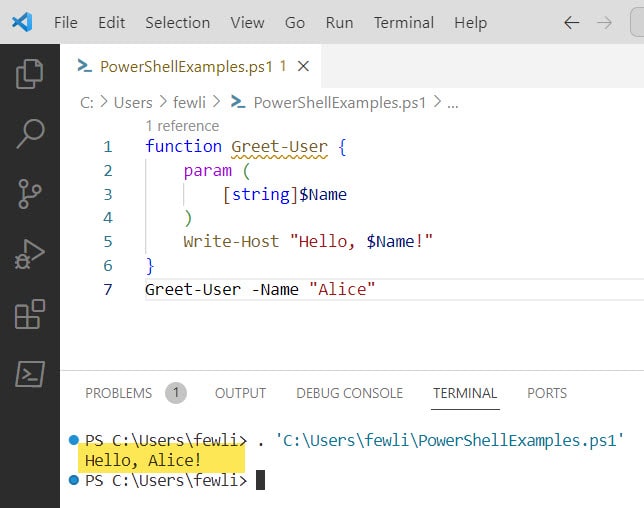
PowerShell Function with Multiple Parameters
You can define multiple parameters when you want to pass more than one piece of data to your PowerShell function. Here’s how you can modify the above function to accept a second parameter:
function Greet-User {
param (
[string]$Name,
[string]$Greeting
)
Write-Host "$Greeting, $Name!"
}
To call function with multiple parameters in PowerShell, you need to pass two arguments like below:
Greet-User -Name "Alice" -Greeting "Good morning"
This would output: Good morning, Alice!
Check out the screenshot for the output below:
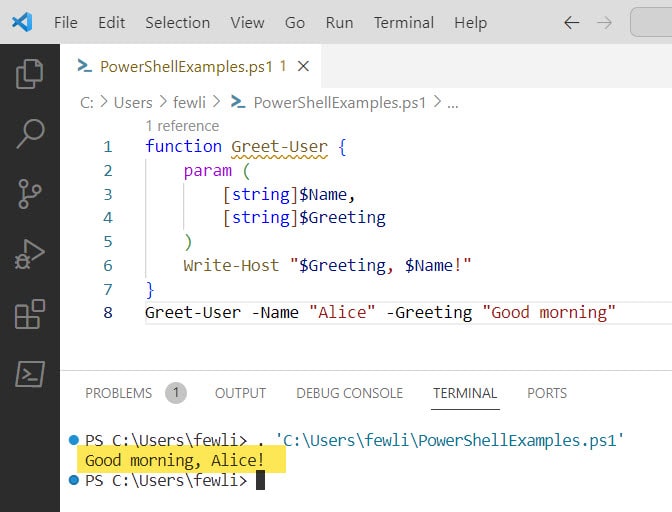
PowerShell function parameter default value
It’s often useful to have default values for some parameters to make them optional. You can assign a default value to a parameter by using the assignment operator =
within the param
block. Here is a complete PowerShell script.
function Greet-User {
param (
[string]$Name = "User",
[string]$Greeting = "Hello"
)
Write-Host "$Greeting, $Name!"
}
Now if you call Greet-User
without any arguments, it will output: Hello, User!
because it uses the default values.
Here you can see in the below screenshot, that I called the function without passing any argument.
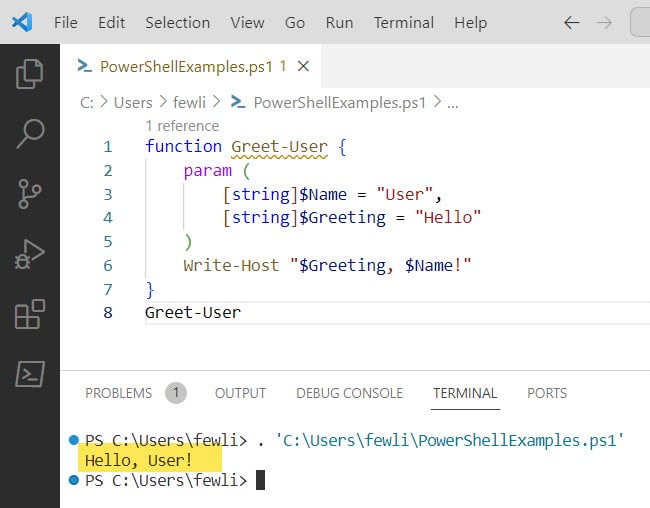
PowerShell function default parameters
In PowerShell, you can also define default parameter values for all functions within a script or a PowerShell session. This is done by using $PSDefaultParameterValues
automatic variable. Here’s an example:
$PSDefaultParameterValues = @{"Greet-User:Greeting"="Welcome"}
After setting this, if you call Greet-User -Name "Bob"
without specifying the Greeting
parameter, it will automatically use “Welcome” as the greeting.
To call the function after setting the default parameter:
Greet-User -Name "Bob"
Optional Parameters in PowerShell Functions
To make a parameter optional, you can either provide a default value, as shown above, or you can use a different approach where you check if the parameter has been provided inside the function body. Here is a complete example of a “PowerShell function optional parameter“.
function Greet-User {
param (
[string]$Name,
[string]$Greeting
)
if (-not $PSBoundParameters.ContainsKey("Greeting")) {
$Greeting = "Hey there"
}
Write-Host "$Greeting, $Name!"
}
In this function, $PSBoundParameters
is a built-in variable that contains all the parameters that were passed to the function. If Greeting
is not in there, the function sets a default.
You can see the output in the screenshot below after I executed the PowerShell script using VS code.
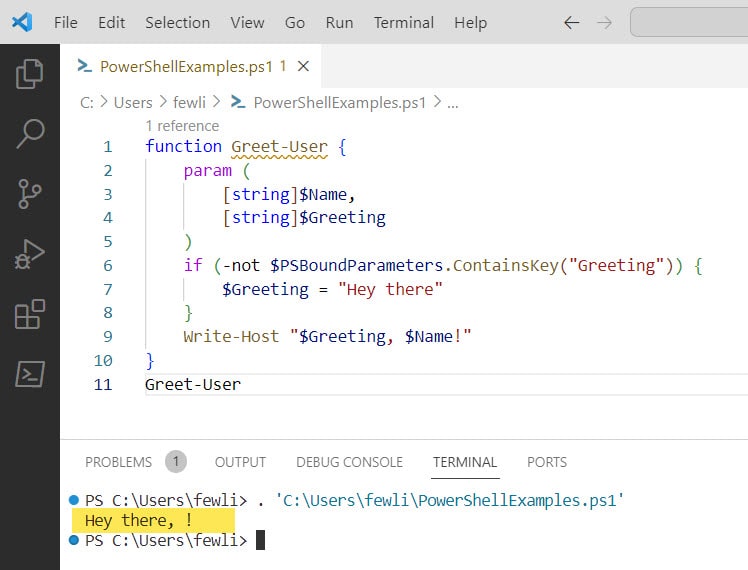
Conclusion
PowerShell functions with parameters are a powerful feature that can greatly enhance your scripting and automation capabilities. In this PowerShell tutorial, I have explained to you the below examples.
- PowerShell function parameters example
- PowerShell function multiple parameters
- PowerShell function param default value
- PowerShell function default parameter
- PowerShell function optional parameter
You may also like:
- PowerShell try catch with examples
- PowerShell Get-date Format Milliseconds
- How to Get-Date Without Time in PowerShell?
- How to Get Current Function Name in PowerShell?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com