Do you want to know about the PowerShell functions? In this PowerShell tutorial, I will explain what is a function in PowerShell, PowerShell function syntax, how to call a function in PowerShell, and various function examples in PowerShell.
What is a PowerShell Function?
A PowerShell function is a block of code designed to perform a specific task. It is declared using the function
keyword, followed by a name, and includes a script block enclosed in braces.
Functions in PowerShell are fundamental constructs that allow you to encapsulate reusable logic. They are blocks of code designed to perform a particular task and can be called upon whenever needed throughout your script.
Advantages of using PowerShell Functions
PowerShell functions are significant for several reasons:
- Code Reuse: They allow for the code to be reused across multiple scripts or within the same script multiple times without repeating the code.
- Scope Management: Functions have their own scope for variables, which prevents unintended changes to variables in the rest of the script.
- Modularity: They enable script writers to break down a complex task into smaller, more manageable pieces, which can be tested and debuged separately.
- Automation: Functions can be combined into modules, enabling the sharing and distribution of complex scripts across systems and teams, which is indispensable for automating tasks consistently and reliably.
PowerShell function syntax
Now, let us see how to define a function in PowerShell.
You declare a function using the function
keyword, followed by the function name and a pair of curly braces {}
that contain the code that makes up the body of the function. PowerShell function names typically follow the Verb-Noun naming convention, like Get-CurrentTime.
Here is a simple PowerShell function syntax.
function Verb-Noun {
# Code to execute
}
Here is a simple example:
function Get-CurrentTime {
return Get-Date
}
In this example, the function Get-CurrentTime
will return the current date and time whenever it is called.
Functions can accept parameters. Parameters are variables that are used to pass data into a function. Parameters are optional, but they make functions more flexible and reusable.
Parameters are defined within param
blocks, allowing functions to accept input. Here is how a simple parameter is defined:
function Verb-Noun {
param (
[Parameter(Mandatory=$true)]
[string]$ParameterName
)
# Function code here
}
- Mandatory Parameter: Set
Mandatory=$true
to make a parameter required. - Data Type: Denote the expected data type
[string]
to increase reliability and readability.
Return Values
Functions can return values using the return
keyword or outputting objects to the pipeline. Here’s an example of returning a value:
function Verb-Noun {
# Function code here
return $ReturnValue
}
- Implicit Return: If
return
is omitted, PowerShell outputs the last evaluated expression in the function. - Explicit Return: Using
return
clarifies what is being output and can improve code readability.
How to Call a Function in PowerShell
Suppose you define a function in PowerShell like below:
function Get-CurrentTime {
return Get-Date
}
Once you have defined a PowerShell function, you can call it by simply using its name in your script, like so:
Get-CurrentTime
When this line is executed, PowerShell looks for a function with the name Get-CurrentTime
, runs the code inside it, and returns the current date and time.
Here is another example where there is a parameter in the PowerShell function.
function Get-Greeting {
param (
[string]$Name
)
"Hello, $Name!"
}
In this example, Get-Greeting is a function that takes one parameter, $Name, and returns a greeting string.
To call the Get-Greeting
PowerShell function that you’ve defined, you need to use the function name followed by the argument for the $Name
parameter. Here’s how you do it:
Get-Greeting -Name "YourName"
or
Get-Greeting -Name "Alice"
You can see here in the screenshot below, after I executed the PowerShell script using VS code.
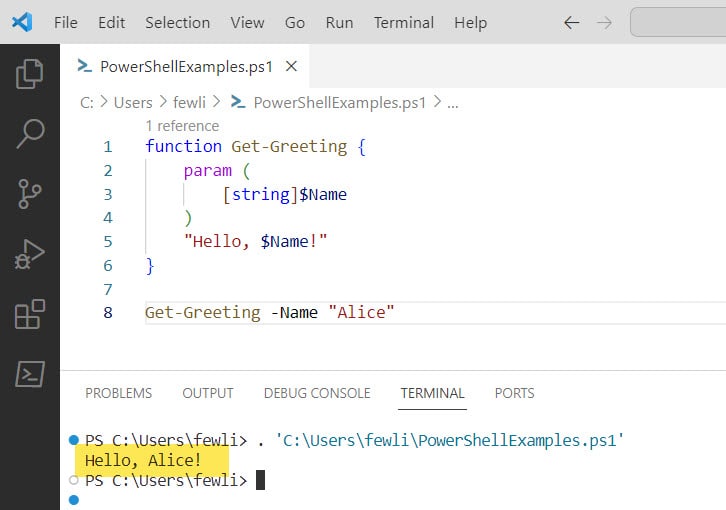
PowerShell Function Scopes and Visibility
In PowerShell, functions have defined scopes that control the visibility and lifetime of variables and commands. These scopes affect how functions interact with the surrounding environment and other functions.
Scope Rules
Global Scope refers to items that are accessible throughout the entire PowerShell session. When a variable or function is defined globally, it can be accessed and modified from any scope.
- Local Scope is created anytime a new function is invoked. Variables and functions defined within this scope are only accessible within the function itself, unless explicitly defined otherwise. For example:
function Set-LocalVariable {
$localVar = 'I am only accessible within this function'
}
- Script Scope pertains to items defined within a script file. They are accessible to anything within that script file but not to the global session or to other script files.
- Private Scope when used, ensures that a variable or function is only visible within the scope it is defined in. Use the
private
keyword to declare a private variable.
A PowerShell function can also access higher scopes using the $global:
or $script:
scope modifiers. However, modifying variables outside of the local scope can lead to less predictable behavior and should be used with caution.
Access Modifiers
Public and Private are the two primary access modifier keywords that control the visibility of a function within a module.
- Public functions are available to users or other modules when the module is imported. They are essentially the exposed surface of a module for users to interact with.
- Private functions are hidden from the user after module import. They are intended to be used internally within the module.
For instance:
function Get-PublicData {
# This function is available outside the module
}
function private:Get-PrivateData {
# This function is hidden from module users
}
No explicit keyword is needed to make a function public; not specifying private:
defaults a function to public scope.
Access modifiers ensure you can orchestrate which parts of a module users can interact with, improving module design and user experience.
Error Handling in Functions in PowerShell
Good error handling is crucial for creating robust PowerShell functions. It ensures a function can gracefully handle unexpected situations, making the code more reliable and user-friendly.
Try-Catch-Finally
PowerShell’s try-catch-finally
block is a structured approach to handling exceptions that occur during the execution of code within the try
block. When an error is thrown, control is passed to the catch
block, where custom error handling can be implemented. Regardless of whether an error occurred, the finally
block executes, allowing for any necessary cleanup.
- Try: Contains the code that might cause an exception.
- Catch: Specifies actions to take when an exception occurs.
- Finally: It contains cleanup code that should run regardless of the outcome.
Example:
function Example-Function {
try {
# Code that may throw an error
}
catch {
# Error handling code goes here
}
finally {
# Cleanup code goes here
}
}
ErrorAction Preference
The $ErrorActionPreference
variable in PowerShell determines how the system responds to non-terminating errors. Settings for this preference can be specified at the script or function level, influencing error-handling behavior without the need for a try-catch-finally
block.
- Continue (Default): Prints an error message and continues execution.
- Stop: Halts the function upon encountering an error.
- SilentlyContinue: Suppresses error messages and continues execution.
- Inquire: Asks the user how to proceed.
Setting $ErrorActionPreference
:
function Example-Function {
[CmdletBinding()]
param()
# Set the preference at the function level
$ErrorActionPreference = 'Stop'
# Code that may generate non-terminating errors
}
By manipulating $ErrorActionPreference
, developers can influence the behavior of their functions when dealing with non-terminating errors, ensuring they follow the desired execution path.
PowerShell Function Naming Conventions
In PowerShell, it’s important to follow a consistent naming convention for functions to ensure your code is easy to read, maintain, and understand. The recommended naming convention for PowerShell functions is the Verb-Noun format, where the verb indicates the function’s action and the noun specifies the entity on which the action is performed.
Verb-Noun Format
A PowerShell function name should start with a verb, followed by a dash, and then a noun. The verb should be selected from a list of approved verbs that PowerShell recognizes. This standardization helps understand what a function does just by looking at its name. For example:
function Get-Inventory {
# Code to retrieve inventory details
}
In this case, Get
is the verb and Inventory
is the noun. This function presumably retrieves inventory details.
Using Approved Verbs
PowerShell has a set of approved verbs that you should use when naming your functions. Using standard verbs can also ensure compatibility with other PowerShell commands and make your functions easily discoverable. To get a list of approved verbs, you can use the Get-Verb
cmdlet in PowerShell.
Pascal Casing
When naming your functions in PowerShell, it’s recommended to use Pascal case, which means capitalizing the first letter of each word in the function’s name. For example:
function Convert-ToCsv {
# Code to convert to CSV
}
Singular Nouns
It’s also advised to use singular nouns rather than plural. This helps keep function names concise and consistent with the naming of other PowerShell cmdlets.
Example of PowerShell Function Naming
Here’s an example of a properly named PowerShell function following the naming convention:
function Set-UserProfile {
param(
[string]$UserName,
[string]$ProfilePath
)
# Code to set the user profile
}
In this example, Set
is the verb, indicating that the function will change or assign a value, and UserProfile
is the noun, indicating that the user profile is what’s being modified.
Conclusion
Functions in PowerShell are a key feature that can make your scripts more modular, reusable, and readable. I hope now you understand:
- What is a function in PowerShell?
- PowerShell function syntax and how to Create PowerShell function
- how to call a function in PowerShell
- PowerShell function examples
- PowerShell function naming conventions
You may also like:
- PowerShell Function Array Parameters
- How to Call Function in If Statement in PowerShell?
- How to Run PowerShell Script in Visual Studio Code
- PowerShell Naming Conventions
- PowerShell Function With Parameters
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com