In PowerShell, we can create functions that accept parameters, including arrays. This tutorial will show us how to create PowerShell functions with array parameters.
An array is a data structure that stores a collection of items, which can be of the same type or different types. In PowerShell, arrays are used to store multiple values in a single variable, making it easier to manipulate and pass around sets of data.
Declare PowerShell Function with an Array Parameter
To declare a function in PowerShell that takes an array as a parameter, you simply define the parameter type as an array using square brackets. Here’s a basic example of a PowerShell function with a parameter as an array.
function Get-ArraySum {
param (
[int[]]$Numbers
)
$sum = 0
foreach ($number in $Numbers) {
$sum += $number
}
return $sum
}
In this example, the Get-ArraySum
function accepts a single parameter $Numbers
, which is expected to be an array of integers. The function calculates the sum of all the numbers in the array.
Passing an Array to a Function in PowerShell
You can pass an array to a PowerShell function in several ways. Here’s an example of how to pass an array directly when calling the function:
$myNumbers = 1, 2, 3, 4, 5
$sum = Get-ArraySum -Numbers $myNumbers
Write-Host "The sum is: $sum"
Alternatively, you can pass the array elements as a comma-separated list directly to the function:
$sum = Get-ArraySum -Numbers 1, 2, 3, 4, 5
Write-Host "The sum is: $sum"
Here is a complete PowerShell script:
function Get-ArraySum {
param (
[int[]]$Numbers
)
$sum = 0
foreach ($number in $Numbers) {
$sum += $number
}
return $sum
}
$myNumbers = 1, 2, 3, 4, 5
$sum = Get-ArraySum -Numbers $myNumbers
Write-Host "The sum is: $sum"
Once you run the PowerShell script using Visual Studio Code, you can see the output in the screenshot below.
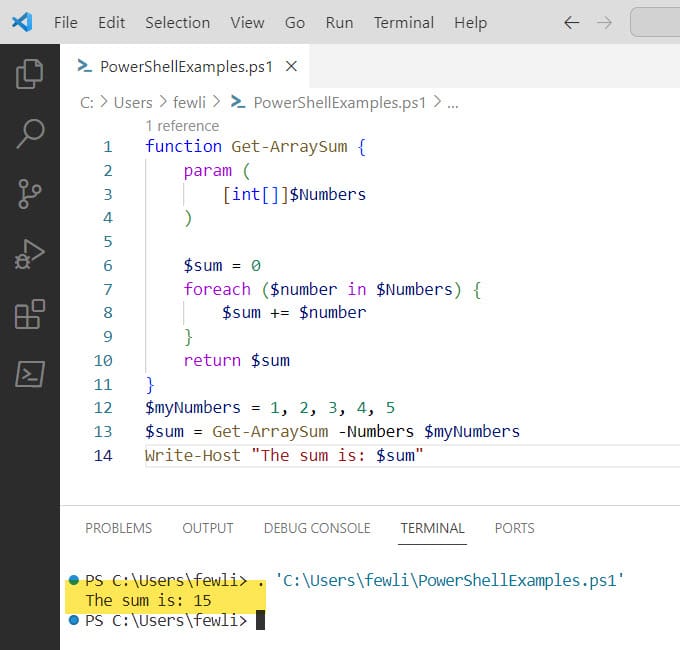
Return an Array from a PowerShell Function
Like passing a parameter as an array to a PowerShell function, we can also return an array from a PowerShell function.
Functions in PowerShell can also return arrays. This is useful when you want to process data within a function and then pass the results back to the caller. Here’s an example:
function Get-MultiplesOfTwo {
param (
[int[]]$Numbers
)
$multiplesOfTwo = @()
foreach ($number in $Numbers) {
if ($number % 2 -eq 0) {
$multiplesOfTwo += $number
}
}
return $multiplesOfTwo
}
$myNumbers = 1..10
$multiples = Get-MultiplesOfTwo -Numbers $myNumbers
Write-Host "Multiples of two: $multiples"
In this example, the Get-MultiplesOfTwo
function returns an array containing only the elements of the $Numbers
array that are multiples of two.
You can see in the screenshot below, the output after I ran the code using VS code.
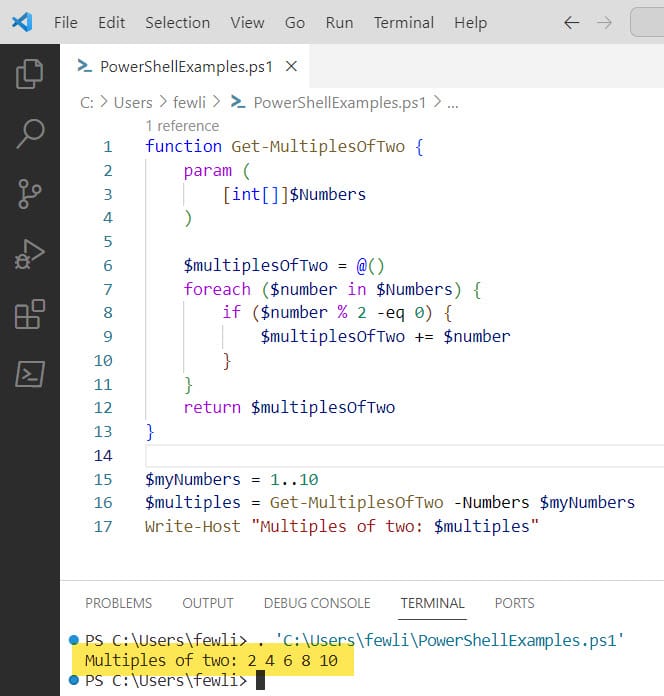
PowerShell function parameter array of objects
In PowerShell, functions can accept parameters of various data types, including arrays of objects. An array is a data structure that can hold multiple values, and when you have an array of objects, each element in the array is an instance of an object with its own properties and methods.
When you define a function in PowerShell, you can specify a parameter that will accept an array of objects by defining the parameter type as an array. For example:
function Process-Objects {
param(
[Parameter(Mandatory=$true)]
[PSObject[]]$ObjectArray
)
foreach ($obj in $ObjectArray) {
# Process each object here
}
}
In this Process-Objects
function, $ObjectArray
is expected to be an array of PSObject
instances, which is a base .NET class that PowerShell uses to encapsulate objects, allowing for flexibility in the types of objects the array can contain.
To pass an array of objects to this PowerShell function, you would first create the array and then call the function with the array as an argument:
# Create an array of custom objects
$myObjects = @(
New-Object PSObject -Property @{Name='Object1'; Value=10},
New-Object PSObject -Property @{Name='Object2'; Value=20}
)
# Pass the array to the function
Process-Objects -ObjectArray $myObjects
The function will then iterate over each object in the array with a foreach
loop and perform actions on them.
Tips for Working with Array Parameters in PowerShell
- When defining array parameters, you don’t have to specify the size of the array. PowerShell arrays are dynamic, meaning they can grow and shrink in size as needed.
- If you pass a single value to an array parameter, PowerShell will automatically wrap this value in an array, so the function still works as expected.
- To ensure type consistency and avoid errors, you can explicitly define the type of elements your array should contain, as seen in the examples above with
[int[]]
.
Conclusion
In this PowerShell tutorial, I will explain everything about PowerShell function array parameters. I have explained how to declare a PowerShell function with an array as a parameter. Also, I will show you how to return an array from a PowerShell function with examples.
You may also like:
- PowerShell try catch with examples
- PowerShell Function With Parameters
- PowerShell get-childitem sort by date
- How To Check If Array Is Empty In PowerShell?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com