An empty array can lead to unexpected results or errors in your PowerShell scripts. In this PowerShell tutorial, I will explain various methods to check if an array is empty in PowerShell. I will also show you how to check if an array contains null or empty in PowerShell.
To check if an array is empty in PowerShell, you can examine its Count
or Length
property. If either of these properties is equal to 0, the array is empty. For example, using $myArray.Count -eq 0
or $myArray.Length -eq 0
in an if statement will allow you to determine if $myArray
contains no elements. Alternatively, you can use $myArray -eq $null
or simply if (-not $myArray)
to check for an empty array as well.
Check If Array Is Empty In PowerShell
In PowerShell, an array is a data structure that stores a collection of items. These items can be of any type, including integers, strings, and objects. Sometimes, you need to verify whether an array is empty—meaning it has no elements. Here are a few methods to check if an array is empty in PowerShell:
Method 1: Using the Count Property
One of the simplest ways to check if an array is empty is by using the Count
property in PowerShell. This property returns the number of elements in the PowerShell array.
# Create an empty array
$myArray = @()
# Check if the array is empty
if ($myArray.Count -eq 0) {
Write-Host "The array is empty."
} else {
Write-Host "The array is not empty."
}
You can see the output in the screenshot below after I executed the above PowerShell script using Visual Studio Code.
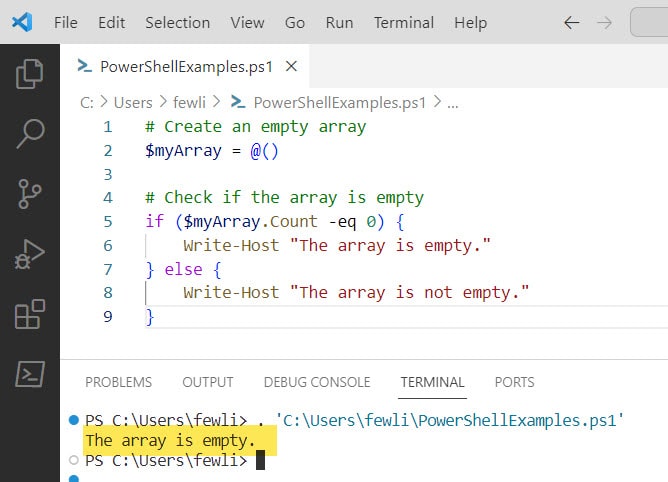
Method 2: Using the Length Property
Similar to the Count
property, you can use the Length
property to determine the number of elements in a PowerShell array.
Here is a complete PowerShell script to check if a PowerShell array is empty by using the length property.
# Create an empty array
$myArray = @()
# Check if the array is empty
if ($myArray.Length -eq 0) {
Write-Host "The array is empty."
} else {
Write-Host "The array is not empty."
}
You can see the output in the screenshot below:
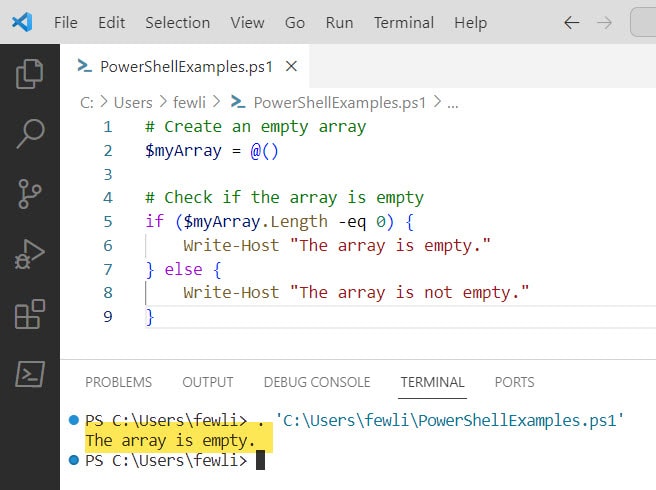
Method 3: Using Conditional Statements Directly
PowerShell allows you to use the array variable in a conditional statement directly. If the array is empty, it will be evaluated as $false
. Here is the PowerShell script to determine if array is empty.
# Create an empty array
$myArray = @()
# Check if the array is empty
if ($myArray) {
Write-Host "The array is not empty."
} else {
Write-Host "The array is empty."
}
This method is succinct and works because PowerShell treats an empty array as $false
when evaluated in a boolean context.
Method 4: Combining Where-Object and Measure-Object
You can also use Where-Object
to filter the array and then pipe it to Measure-Object
to count the elements. This method is useful if you want to filter out specific values (like $null
or empty strings) before counting.
# Create an array with a mix of null, empty, and non-empty strings
$myArray = $null, "", "PowerShell", $null
# Check if the array is empty after filtering out null and empty strings
if ($myArray | Where-Object { $_ -ne $null -and $_ -ne "" } | Measure-Object).Count -eq 0 {
Write-Host "The array is empty."
} else {
Write-Host "The array is not empty."
}
Here’s a complete PowerShell script that demonstrates each method to check if an array in empty in PowerShell.
# Define an array
$myArray = @()
# Method 1: Using Count
if ($myArray.Count -eq 0) {
Write-Host "Method 1: The array is empty."
}
# Method 2: Using Length
if ($myArray.Length -eq 0) {
Write-Host "Method 2: The array is empty."
}
# Method 3: Using conditional statement directly
if (-not $myArray) {
Write-Host "Method 3: The array is empty."
}
# Method 4: Using Where-Object and Measure-Object
if ($myArray | Where-Object { $_ } | Measure-Object).Count -eq 0 {
Write-Host "Method 4: The array is empty."
}
When you run this script with an empty array, it will output that the array is empty for each method.
PowerShell check if an array contains null or empty
In PowerShell, you may sometimes need to check if an array contains any null or empty elements, which is slightly different from checking if the entire array is empty. Now, let us check different methods to check if an array contains null or empty in PowerShell.
Using Where-Object
The Where-Object
cmdlet is useful for filtering arrays based on certain conditions. You can use it to check if any elements are $null
or an empty string (""
) in PowerShell.
# Assume $myArray is your array
$nullOrEmpty = $myArray | Where-Object { -not $_ -or $_ -eq "" }
if ($nullOrEmpty) {
Write-Host "Array contains null or empty elements."
} else {
Write-Host "Array does not contain null or empty elements."
}
In this example, -not $_
checks for $null
values and $_ -eq ""
checks for empty strings. If $nullOrEmpty
has any items after the Where-Object
filter, it means the original array contained null or empty elements.
Using foreach
Loop
You can also iterate through each element in the PowerShell array and check for null or empty values using a foreach
loop.
# Assume $myArray is your array
$containsNullOrEmpty = $false
foreach ($item in $myArray) {
if (-not $item -or $item -eq "") {
$containsNullOrEmpty = $true
break
}
}
if ($containsNullOrEmpty) {
Write-Host "Array contains null or empty elements."
} else {
Write-Host "Array does not contain null or empty elements."
}
The loop breaks as soon as it finds a null or empty element, setting $containsNullOrEmpty
to $true
.
Using Any
Method
If you’re working with PowerShell 3.0 or later, you can use the Any
method from LINQ, which can be more concise.
# Assume $myArray is your array
$containsNullOrEmpty = [System.Linq.Enumerable]::Any([System.Collections.Generic.List[object]]$myArray, {[string]::IsNullOrEmpty($_)})
if ($containsNullOrEmpty) {
Write-Host "Array contains null or empty elements."
} else {
Write-Host "Array does not contain null or empty elements."
}
This method uses type casting to treat $myArray
as a generic list and then applies the Any
method with a condition that checks for null or empty strings.
Conclusion
In this tutorial, you’ve learned several methods to check if an array is empty in PowerShell. I have explained here the below 4 methods to check if an array is empty in PowerShell.
- Using the Count Property
- Using the Length Property
- Using Conditional Statements Directly
- Combining Where-Object and Measure-Object
I have also explained a few methods to check if a PowerShell array contains null or empty elements.
You may also like:
- How to Loop Through a PowerShell Array?
- How to Replace a String in an Array Using PowerShell?
- How To Access First Item In an Array In PowerShell?
- How to Replace Strings Containing Backslashes in PowerShell?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com