PowerShell arrays are a foundational construct in the PowerShell scripting language, used to store collections of items. Much like any other programming or scripting language, arrays in PowerShell hold multiple values, which can be of different data types, and allow the user to iterate through those items for various automation and scripting tasks.
A PowerShell array is a data structure that is designed to store a collection of items, which can be of the same type or different types. Arrays are used to store multiple items in a single variable, allowing you to access, iterate over, and manipulate these items efficiently. In PowerShell, arrays can contain various types of items, including strings, integers, objects, and even other arrays
The structure of a PowerShell array enables it to store a set of items where each item can be accessed using its index. These indices start at 0, meaning the first item in the array is at index 0, the second at index 1, and so on. This allows for precise control over the data stored within an array. PowerShell also provides several ways to create and manipulate arrays, such as adding or removing items, sorting the collection, or finding specific elements based on a condition.
Furthermore, PowerShell arrays can be expanded dynamically, meaning items can be added to an array even if it was not initially declared with a specific size. This flexibility makes it easier for users to work with varying lengths of data sets without determining the size beforehand. PowerShell also provides a range of cmdlets specifically designed for array manipulation, making it a robust tool for administrators who handle complex data structures.
What is a PowerShell Array?
Arrays in PowerShell are essential for handling collections of elements, such as strings, numbers, or objects. They store multiple items that can be accessed and manipulated individually or as a group.
Create an array in PowerShell
In PowerShell, arrays can be created by assigning multiple values to a variable, separated by commas. They can also be generated using the @( )
array subexpression operator, which ensures that the result is always an array.
# Creating an array with comma-separated values
$colors = "red", "green", "blue"
# Ensuring the result is an array using the array subexpression operator
$numbers = @(1, 2, 3)
Access PowerShell Array Elements
Elements in a PowerShell array are accessed using zero-based indexing, meaning the first element is at index 0. One can also use a range of indices or negative indices to access the elements.
# Accessing the first item of the colors array
$firstColor = $colors[0]
# Accessing multiple items using a range
$range = $colors[1..2]
# Accessing the last item using negative indexing
$lastNumber = $numbers[-1]
Modify an Array in PowerShell
Arrays can be modified by directly setting an element at a specific index or by using methods to add and remove elements. However, arrays in PowerShell are of fixed size, and such modifications are internally done by creating new arrays.
# Modifying an existing element
$colors[0] = "yellow"
# Adding an element using the `+=` operator
$colors += "purple"
# Removing an element by value
$numbers = $numbers | Where-Object { $_ -ne 2 }
Working with PowerShell Array Elements
In PowerShell, array elements are managed through various operations such as looping, filtering, and sorting. These operations enable the manipulation of the data stored in arrays, allowing for efficient data handling and transformation.
Looping Through Arrays
To iterate over each element in an array in PowerShell, one uses a loop structure. The foreach
loop is commonly utilized for this purpose:
$array = 1, 2, 3, 4, 5
foreach ($element in $array) {
Write-Host $element
}
This presents each element in the array to the console.
Filtering Arrays
Arrays can be filtered to include only elements that match certain conditions using the Where-Object
cmdlet.
$array = 1..10
$filteredArray = $array | Where-Object { $_ -gt 5 }
The example above filters the array to include only elements greater than 5. The $_
symbol represents each element being processed.
Sorting Arrays
Sorting an array is achieved with the Sort-Object
cmdlet. An array can be sorted in ascending or descending order:
$array = 5, 1, 4, 2, 3
$sortedArrayAsc = $array | Sort-Object
$sortedArrayDesc = $array | Sort-Object -Descending
The first command sorts the array in ascending order, while the second command sorts it in descending order.
Advanced Array Operations in PowerShell
PowerShell provides robust functionality for array manipulation that goes beyond basic operations. The following subsections highlight multi-dimensional arrays, array arithmetic, and the methods for joining and splitting arrays.
Multi-Dimensional Arrays
Multi-dimensional arrays are arrays that contain other arrays as their elements. In PowerShell, one can create a two-dimensional array by initializing an array of arrays:
$multiArray = @( @(1, 2), @(3, 4), @(5, 6) )
Each element can be accessed using two indices: the first for the row, and the second for the column:
$row = 0
$column = 1
$value = $multiArray[$row][$column]
Array Arithmetic
PowerShell allows arithmetic operations to be performed on arrays in a vectorized manner. When arithmetic is applied, it is executed on each element of the array:
$array1 = 1..5
$array2 = 6..10
# Adding arrays
$sumArray = $array1 + $array2
One must note that, unlike mathematical vectors, the +
operator in PowerShell concatenates arrays rather than adding corresponding elements. To perform element-wise addition, one would use a loop or the ForEach-Object
cmdlet:
$addedArrays = $array1 | ForEach-Object { $_ + $array2[$array1.IndexOf($_)] }
Joining and Splitting Arrays
Concatenating arrays in PowerShell is straightforward and uses the +
operator:
$firstArray = 1..3
$secondArray = 4..6
# Joining arrays
$joinedArray = $firstArray + $secondArray
To split an array into smaller arrays, one can use the Select-Object
cmdlet with the -Skip
and -First
parameters:
# Splitting arrays
$firstPart = $joinedArray | Select-Object -First 3
$secondPart = $joinedArray | Select-Object -Skip 3
These advanced operations allow users to process and manipulate array data within their PowerShell scripts.
Arrays and PowerShell Cmdlets
PowerShell cmdlets frequently interact with arrays, allowing for efficient data manipulation and storage. This section will discuss how arrays are integrated with PowerShell cmdlets, focusing on conversion techniques and the use of arrays within the pipeline.
Converting To and From Arrays
To convert data to an array in PowerShell, one can use the @()
array subexpression operator or cast a collection to an array type [array]
. For example, they can take command output and explicitly cast it:
[array]$myArray = Get-Process
Alternatively, simply enclosing command output in @()
ensures that it’s stored as an array:
$myArray = @(Get-Process)
For converting an array back to individual elements, one might use a loop construct such as foreach
, or they could leverage cmdlets like ForEach-Object
. Also, if they need a single string from an array, they can utilize the -join
operator.
Combining Arrays with Pipelines
PowerShell’s pipeline is designed to pass objects, not just text. Arrays are seamlessly pushed through the pipeline, one element at a time. Each cmdlet in the pipeline operates on a single object before passing it along.
To illustrate, given two arrays, $array1
and $array2
, they can be combined and sent through the pipeline with:
$array1 + $array2 | Sort-Object
Furthermore, using ForEach-Object
within the pipeline allows for operations on each element in the array:
$array1 | ForEach-Object { $_ * 2 }
This would double every number in $array1
if it contains numerical values. The pipeline, thus is a powerful tool when working with arrays in PowerShell.
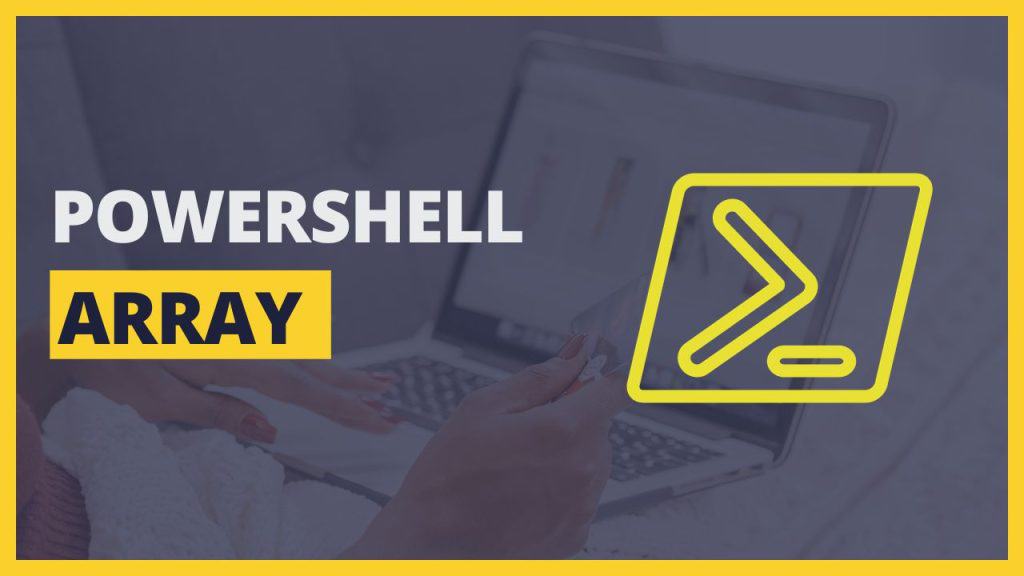
Conclusion
PowerShell arrays are integral to managing and manipulating collections of data efficiently. They allow users to easily store multiple items in a single variable and perform actions on each element. The use of arrays is a foundational skill for any PowerShell user.
Flexibility and Scalability:
- Arrays in PowerShell are dynamic, meaning the size of an array is not fixed and can be adjusted as needed.
- They can hold elements of various types, allowing complex data structures to be easily manipulated.
Commands and Operations:
- Users have a range of commands at their disposal, such as
ForEach-Object
, which can iterate through each item of an array. - Sorting and filtering are simplified through native PowerShell cmdlets like
Sort-Object
andWhere-Object
.
Custom Objects and Hashtable Integration:
- Arrays can hold custom objects, enabling the storage of structured data.
- The combination of hashtables and arrays can produce more sophisticated data representations.
Performance Considerations:
- PowerShell arrays have performance implications; large arrays may affect script execution times.
- Alternative data structures should be considered for performance-critical applications.
PowerShell arrays are a powerful feature for data manipulation. I hope you know how to work with the PowerShell array.
You may also like:
- How to create an array in PowerShell from CSV file
- PowerShell Copy Item examples
- PowerShell Create Log File
- How to Replace a String in an Array Using PowerShell?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com