In this PowerShell tutorial, we will discuss how to create a log file in PowerShell. We use log files to track information while running a PowerShell script.
If you are a little familiar with PowerShell, then you might realize we use PowerShell for simple to complex and to complete a long time-consuming task. Your PowerShell script might also be running automatically through a scheduler.
So it is very much necessary that, we should tack information while the script is running and that when we use log files. We can track information, error, warning, etc on a log file in PowerShell.
But you should not log sensitive information or personal data like passwords, credit card numbers, email addresses, social security numbers, etc.
So, let us see different ways to create a log file in PowerShell.
PowerShell create log file
We will discuss here different approaches to writing information to a log file as well as how to create PowerShell log file.
I always use PowerShell ISE to write, test, and debug PowerShell scripts. You can use the Visual Studio Code also.
Open PowerShell ISE in administrator mode (Right-click and Run as administrator) and go through the examples step by step.
Example-1: PowerShell create simple log file
This is one of the simplest ways to write a message to a log file in PowerShell.
$logfilepath="D:\Log.txt"
$logmessage="This is a test message for the PowerShell create log file"
$logmessage >> $logfilepath
The above PowerShell script will write the message in the Log.txt file that is presented in D drive.
If the file is presented before then it will keep appending the message like by like. If the Log.txt file is not presented in the D drive, then it will create the log file first and then write the message.
Example-2: Create a simple log file in PowerShell
The below example will check if the log file is presented already, then it will delete the file create a new file, and write the message into it.
$logfilepath="D:\Log.txt"
$logmessage="This is a test message for the PowerShell create log file"
if(Test-Path $logfilepath)
{
Remove-Item $logfilepath
}
$logmessage >> $logfilepath
We can use the Test-Path PowerShell command to check if a file exists or not in the file path.
Example-3:
We can also use the date and time when the message is logged using PowerShell Get-Date.
$logfilepath="D:\Log.txt"
$logmessage="This is a test message for the PowerShell create log file"
if(Test-Path $logfilepath)
{
Remove-Item $logfilepath
}
$logmessage +" - "+ (Get-Date).ToString() >> $logfilepath
The PowerShell script will log the message with the current date and time.
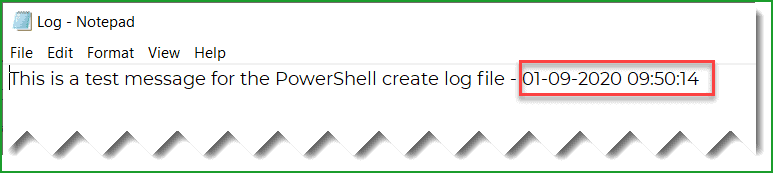
Example-4:
If you want to track multiple log messages, then you can create a PowerShell function like the one below:
$logfilepath="D:\Log.txt"
function WriteToLogFile ($message)
{
$message +" - "+ (Get-Date).ToString() >> $logfilepath
}
if(Test-Path $logfilepath)
{
Remove-Item $logfilepath
}
WriteToLogFile "This is a test message for the PowerShell create log file"
WriteToLogFile "This is another message"
WriteToLogFile "This is another another message"
The above PowerShell script will check if the log file exists in the path, if yes then it will delete the file. Then it will call 3 times the function to write the messages to the PowerShell log file.
Example-5:
Below is a PowerShell script where we have used the Add-content PowerShell cmdlets to write the message to a .log file.
$logfilepath = "D:\Logs.log"
function WriteToLogFile ($message)
{
Add-content $logfilepath -value $message
}
if(Test-Path $logfilepath)
{
Remove-Item $logfilepath
}
WriteToLogFile "This is a test message for the PowerShell create log file"
WriteToLogFile "This is another message"
WriteToLogFile "This is another another message"
Rather than using a .txt file, most of the time, we write the extension as a .log file.
Example-6:
We can also use Start-Transcript PowerShell cmdlets to write to a log file. This not only writes the log message but also tracks other information like username, machine, host application, PSVersion, CLRVersion, etc.
$logfilepath="D:\Log.log"
Start-Transcript -Path $logfilepath
Write-Host "This is a message"
Stop-Transcript
The file looks like below:
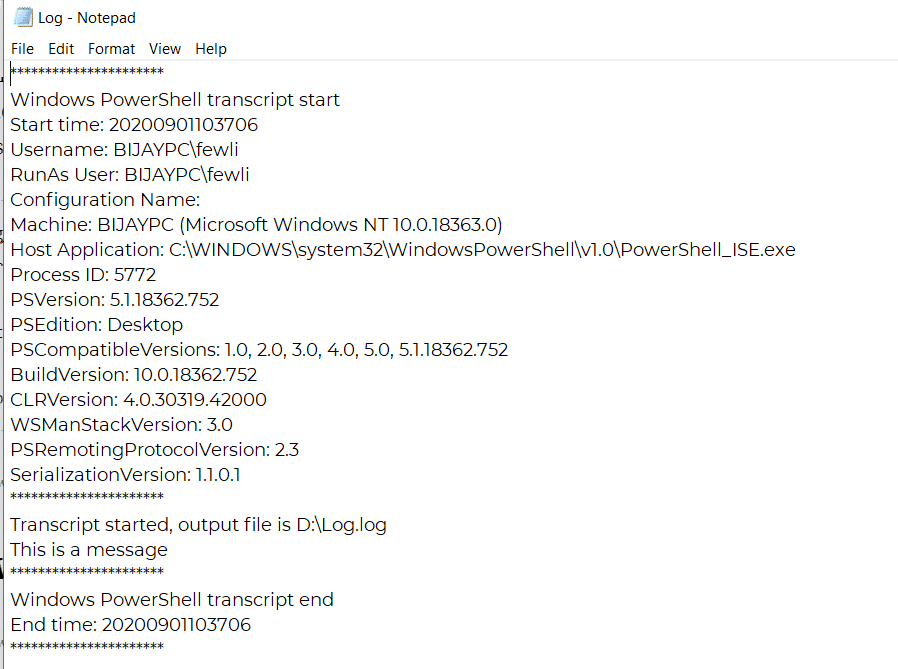
PowerShell Log Levels
In the Write- PowerShell cmdlets, PowerShell provides various types of log levels like:
- Write-Verbose
- Write-Debug
- Write-Information
- Write-Warning
- Write-Error
PowerShell create log file with date
Now, let us see how to create log file with date in PowerShell. Basically, it will create the file with the file name as of today’s date.
The script will check if any file exists with name as today’s date, if the file exists, then it will delete will first delete the file, else it will create the file and write the log message into it.
$todaysdate=Get-Date -Format "MM-dd-yyyy"
$logfilepath = "D:\"+$todaysdate+".log"
function WriteToLogFile ($message)
{
Add-content $logfilepath -value $message
}
if(Test-Path $logfilepath)
{
Remove-Item $logfilepath
}
WriteToLogFile "PowerShell log file message"
WriteToLogFile "This is another message"
WriteToLogFile "This is another another message"
This is how we can create log file with date in PowerShell.
This is how we can create a daily log file in PowerShell that will be created on today’s date.
PowerShell create log file with datetime
Now, we can see how to create a log file with datetime using PowerShell.
We need to format the Get-Date PowerShell cmdlet to get the current date and time like below:
$todaysdate=Get-Date -Format "MM-dd-yyyy-hh-mm"
$logfilepath = "D:\"+$todaysdate+".log"
function WriteToLogFile ($message)
{
Add-content $logfilepath -value $message
}
if(Test-Path $logfilepath)
{
Remove-Item $logfilepath
}
WriteToLogFile "PowerShell log file message"
In the above example, the PowerShell log file will be created on the name as today’s date and time.
PowerShell create log file if not exists
Now, let us see how to create a log file if not exists in PowerShell.
We can use the Test-Path PowerShell cmdlet to check if the file exists in the path or not.
And we can use New-Item PowerShell cmdlets to create a file in the folder path.
$todaysdate=Get-Date -Format "MM-dd-yyyy"
$folderpath="D:\"
$filename = $todaysdate+".log"
if (!(Test-Path $logfilepath))
{
New-Item -itemType File -Path $folderpath -Name $filename
}
You can see the output below:
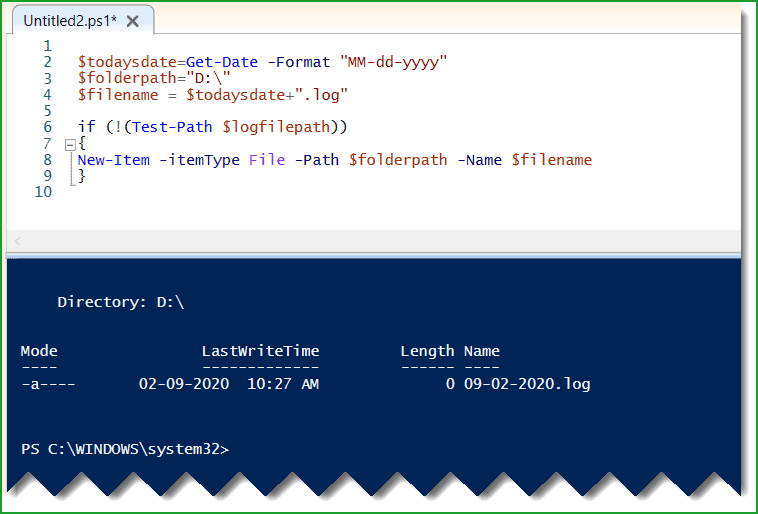
This is how we can create a log file if not exists in PowerShell.
PowerShell create log file with headers
Now, let us see how to create a log file with headers in PowerShell. Here we will create a .csv file and also will add headers to the CSV file.
$filename = "Log-"+ (Get-Date -Format "MM-dd-yyyy")
$outfile = "D:\"+$filename+".csv"
$newcsv = {} | Select "DateAndTime","Message" | Export-Csv $outfile
$csvfile = Import-Csv $outfile
$csvfile.DateAndTime = Get-Date -Format "MM-dd-yyyy-hh-mm"
$csvfile.Message = "This is a test message"
$csvfile | Export-CSV $outfile
The above PowerShell script will create a .csv file and then it will add two headers (DateAndTime and Message). We will add a test log message also.
PowerShell create error log file
Now, we will see how to create an error log file in PowerShell. Basically, we will use the try-catch statement, and in the catch statement, we will log the error message in a log file in PowerShell.
$filename = "Log-"+ (Get-Date -Format "MM-dd-yyyy")
$logfile = "D:\"+$filename+".log"
try
{
1/0
}
catch
{
$_.Exception.Message | Out-File $logfile
}
In the above PowerShell script, whenever an error will appear in the try statement, then the message will be logged in the catch statement in the PowerShell error log file.
PowerShell create csv log file
In the same way we are creating .txt or .log file, we can also create .csv file in PowerShell to log information.
Now, let us see how to create a .csv log file in PowerShell.
To create a .csv file, we just need to change the file extension to .csv.
$todaysdate=Get-Date -Format "MM-dd-yyyy"
$logfilepath = "D:\"+$todaysdate+".csv"
function WriteToLogFile ($message)
{
Add-content $logfilepath -value $message
}
if(Test-Path $logfilepath)
{
Remove-Item $logfilepath
}
WriteToLogFile "PowerShell log file message"
This is how we can create csv log file in PowerShell.
Use PowerShell Framework (PSFramework) to Log Information
You can also use PSFramework or PowerShell Framework to log information.
To use PSFramework, the first thing you need to install it by running the following command.
Install-Module PSFramework
By using the PSFramework, we can write to the log file by using the Write-PSFMessage script.
PowerShell create simple log file
Now, let us see how to create a simple log file in PowerShell. The PowerShell script will create a very simple log file and will log a text message. The file will be created on today’s date.
$filename = "Log- "+ (Get-Date -Format "MM-dd-yyyy")
$logfilepath = "C:\"+$filename+".log"
New-Item -Path $logfilepath -ItemType file
Add-Content -Path $logfilepath -Value "This is a text file message"
In this PowerShell tutorial, we learned how to create a log file in PowerShell and the following things:
- PowerShell create log file
- PowerShell create log file with date
- PowerShell log file with date and time
You may also like:
- PowerShell Copy Item examples
- PowerShell get file size
- PowerShell create folder if not exists
- How to Loop Through a PowerShell Array?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com