Do you want to create a directory if not exists in PowerShell? In this PowerShell tutorial, I will explain to you how to create a folder if not exists in PowerShell. Recently, I got a requirement of creating directories (folders), but only if they don’t already exist; I will guide you through writing a PowerShell script that checks if a directory exists and, if not, creates it.
To create a folder in PowerShell only if it doesn’t already exist, use the Test-Path cmdlet to check for the folder’s presence and New-Item to create it if necessary. Here’s a concise script that accomplishes this: $folderPath = “C:\MyFolder”
if (-not (Test-Path -Path $folderPath)) { New-Item -Path $folderPath -ItemType Directory }
This script will ensure that “C:\MyFolder” is created if it isn’t there already, preventing any errors if the folder exists.
PowerShell create folder if not exists
Now, let us see how to create a folder in PowerShell if it does not exist using the Test-Path Cmdlet and New-Item PowerShell cmdlets.
- Test-Path: This cmdlet checks for the existence of a path (file or directory) and returns True if it exists, False otherwise.
- New-Item: This cmdlet is used to create new items, including directories.
The Test-Path cmdlet in PowerShell is designed to check for the existence of a file or directory. We can use this in conjunction with the New-Item cmdlet to create a folder only if it doesn’t exist.
$folderPath = "C:\MyFolder"
# Check if the folder already exists
if (-not (Test-Path -Path $folderPath)) {
# The folder does not exist, so create it
New-Item -Path $folderPath -ItemType Directory
Write-Host "Folder created: $folderPath"
} else {
Write-Host "Folder already exists: $folderPath"
}
Once I executed the PowerShell script using Windows PowerShell ISE, you can see the output in the screenshot below.
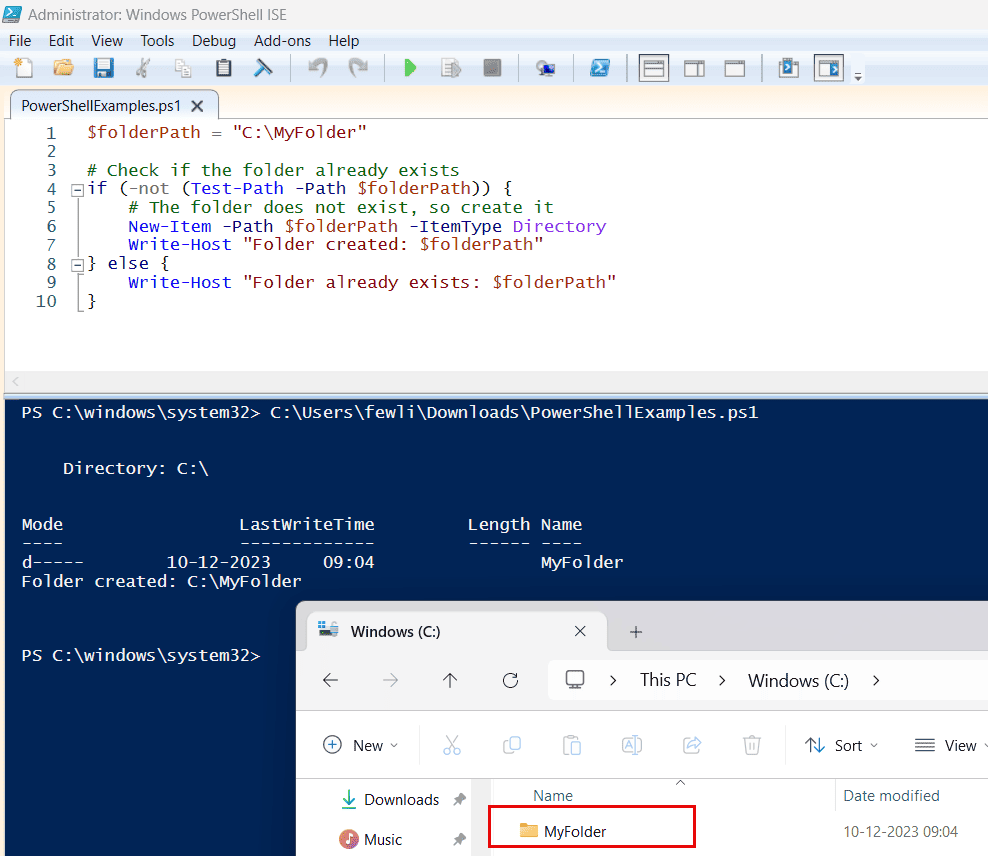
In this script, we first define the path of the folder we want to create. We then use Test-Path to check if the folder exists. If it doesn’t, we proceed to create it with New-Item.
You can also create a function to reuse; here is the complete PowerShell script:
function Ensure-FolderExists {
param (
[string]$Path
)
if (-not (Test-Path -Path $Path)) {
New-Item -Path $Path -ItemType Directory
Write-Host "Folder created: $Path"
} else {
Write-Host "Folder already exists: $Path"
}
}
# Usage
Ensure-FolderExists -Path "C:\MyFolder"
You can also use the try-catch if you want to handle potential errors.
$directoryPath = "C:\Example\NewFolder"
try {
if (-not (Test-Path -Path $directoryPath)) {
New-Item -Path $directoryPath -ItemType Directory -ErrorAction Stop
Write-Host "Directory created: $directoryPath"
} else {
Write-Host "Directory already exists: $directoryPath"
}
} catch {
Write-Host "Error creating directory: $_"
}
PowerShell create directory if not exists using mkdir
PowerShell has an alias md (short for ‘make directory’) which is equivalent to mkdir in Unix-like systems. This is a quicker way to create folders but does not have a built-in check for the existence of the folder. However, we can still combine it with Test-Path.
Here is the full PowerShell script to make a directory if not exists in PowerShell.
$folderPath = "C:\MyFolder"
# Check if the folder already exists
if (-not (Test-Path -Path $folderPath)) {
# The folder does not exist, so create it
md $folderPath
Write-Host "Folder created: $folderPath"
} else {
Write-Host "Folder already exists: $folderPath"
}
Here is the output you can see in the screenshot below:
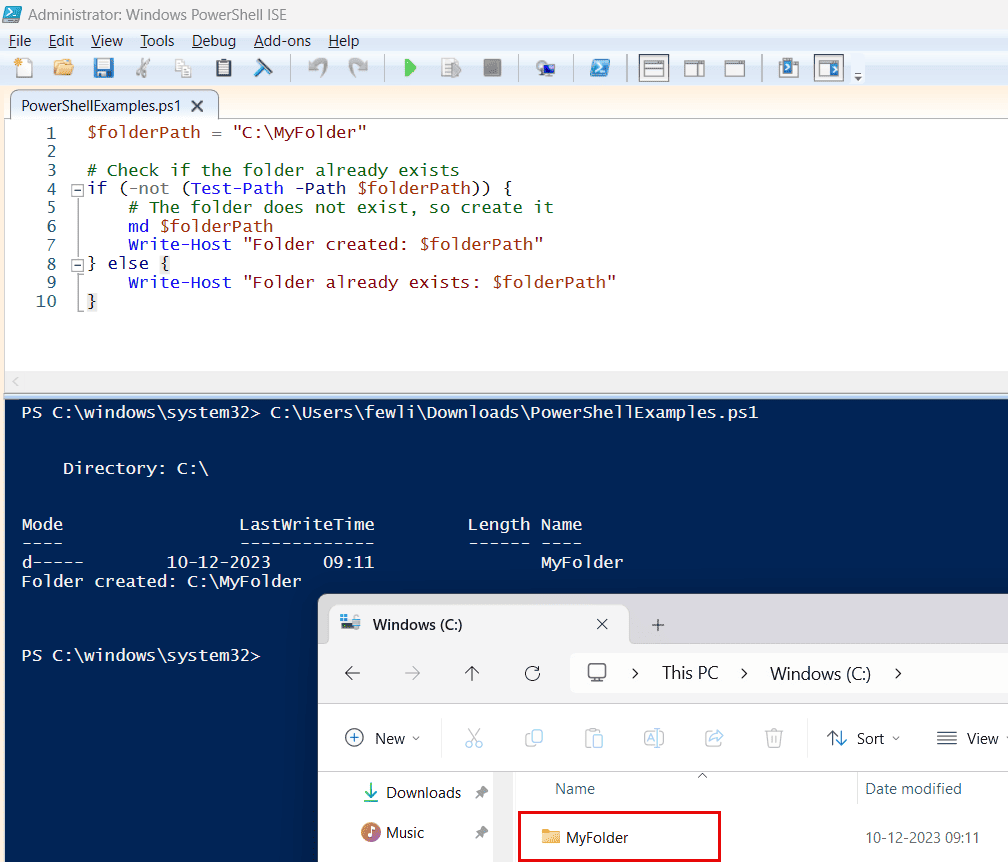
Create a folder if not exist in PowerShell using New-Item with the -Force Parameter
The New-Item cmdlet has a -Force parameter that can be used to create a directory even if it already exists in PowerShell, without throwing an error. While this doesn’t prevent the directory from being created if it exists, it ensures that the script continues without interruption.
Here is the PowerShell script:
$folderPath = "C:\MyFolder"
# Use the -Force parameter to create the folder even if it already exists
New-Item -Path $folderPath -ItemType Directory -Force
Write-Host "Folder is ensured to exist: $folderPath"
This method is less about checking for the folder’s existence and more about making sure the folder is there without worrying about whether it was created in this instance or was already present.
PowerShell create a directory if not exist using .NET classes
This method uses .NET classes directly and is useful if you prefer or need .NET functionality. It provides System.IO.Directory that you can use to create a directory if not exist in PowerShell.
$directoryPath = "C:\NewFolder"
if (-not ([System.IO.Directory]::Exists($directoryPath))) {
[System.IO.Directory]::CreateDirectory($directoryPath)
Write-Host "Directory created: $directoryPath"
} else {
Write-Host "Directory already exists: $directoryPath"
}
Conclusion
In this PowerShell tutorial, I have explained various methods to create a folder if it does not exist in PowerShell. If you have any questions related to “PowerShell create directory if not exists“, do let me know.
You may also like:
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com