Do you want to know how to create a file if not exist in PowerShell? In this PowerShell, I will explain to you how to create a file if not exists in PowerShell. I will show you different methods of “PowerShell create file if not exists”.
To create a file in PowerShell if it doesn’t already exist, you can use the New-Item cmdlet in combination with Test-Path. For example: $filePath = “C:\Dummy\file.txt”; if (-not (Test-Path $filePath)) { New-Item -Path $filePath -ItemType File } This script checks if the file exists using Test-Path and creates it with New-Item if it does not.
Now. let us check various methods to create a file if not exists in PowerShell.
Note: Remember, always ensure you have the correct permissions to create files in the specified directory and handle any potential errors that might arise during file creation.
PowerShell create file if not exists using New-Item Cmdlet
The New-Item cmdlet is a straightforward way to create a new file in PowerShell. This method checks for the file’s existence before creating it. Here is the complete PowerShell script to create a text file if not exist.
$filePath = "C:\Bijay\Dummy\file.txt"
if (-not (Test-Path $filePath)) {
New-Item -Path $filePath -ItemType File
} else {
Write-Host "File already exists."
}
In this script, Test-Path checks if the file exists. If it does not (-not (Test-Path $filePath)), New-Item creates the file. Once you run the PowerShell script, you can see the output in the screenshot below.
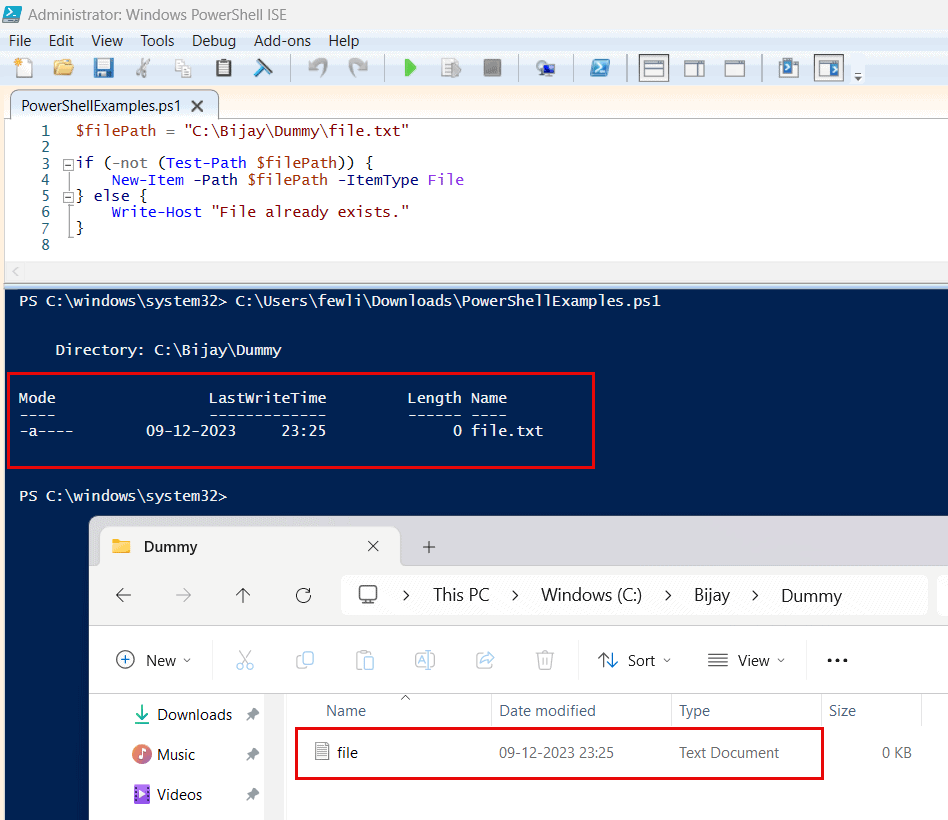
PowerShell create file if not exist using Out-File
The Out-File cmdlet is typically used for output redirection but can also be used for file creation in PowerShell. Here is a complete PowerShell script to create a file if not exist using out-file in PowerShell.
$filePath = "C:\Bijay\Dummy\NewFile.txt"
if (-not (Test-Path $filePath)) {
"" | Out-File $filePath
} else {
Write-Host "File already exists."
}
This method creates an empty file by redirecting an empty string (“”) to the file. If the file exists, the script does not alter it. Here is the output after I executed the PowerShell script using PowerShell ISE.powershell create text file if not exist
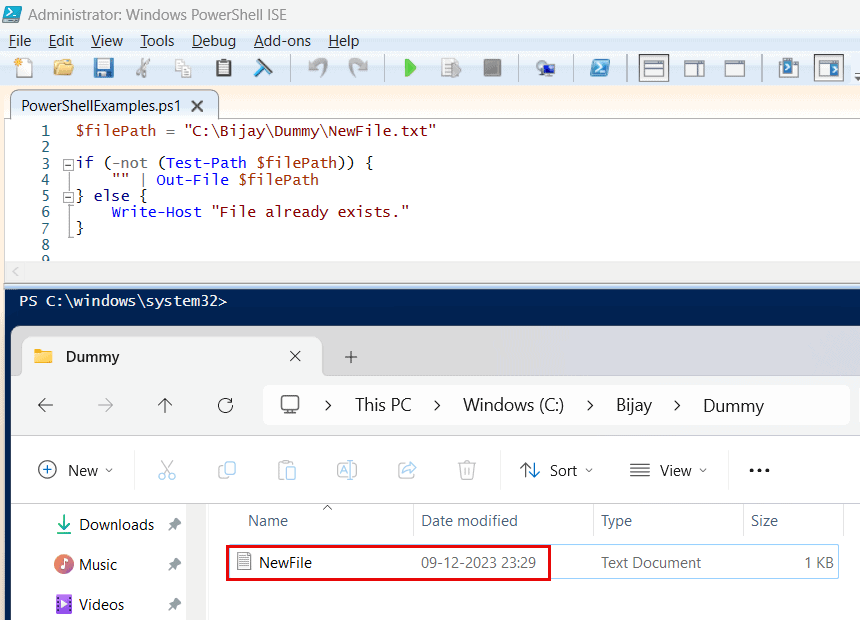
PowerShell create text file if not exist using Add-Content
Add-Content PowerShell cmdlet is another way to create a file, especially if you want to add some content to it if it doesn’t exist. This method adds specified content to the new file. If the file already exists, it leaves the file unchanged.
Here is the complete PowerShell script to create a text file if it does not exist.
$filePath = "C:\Bijay\Dummy\NewFile.txt"
$content = "Initial content of the file."
if (-not (Test-Path $filePath)) {
Add-Content -Path $filePath -Value $content
} else {
Write-Host "File already exists."
}
Once you execute the PowerShell script, it will create a text file with default content; check the screenshot below:
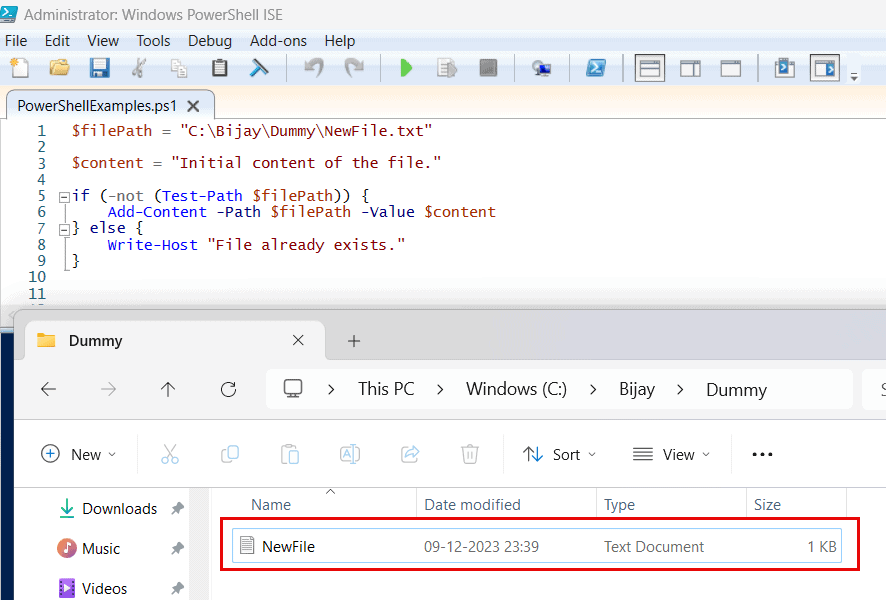
Create a file if not exists in PowerShell using .Net Class
Now, let us see how to use the .Net class to create a file if it does not exists in PowerShell.
If you prefer to use .NET classes in PowerShell, then it provides the System.IO.File class, which you can use to create a file if not exists. Here is a complete script. Here, System.IO.File::Create is used to create the file. The Dispose method is called to release the file handle immediately.
$filePath = "C:\Bijay\Dummy\NewFile.txt"
if (-not (Test-Path $filePath)) {
[System.IO.File]::Create($filePath).Dispose()
} else {
Write-Host "File already exists."
}
Once you execute the PowerShell script using Windows PowerShell ISE, you can see the output like the below;
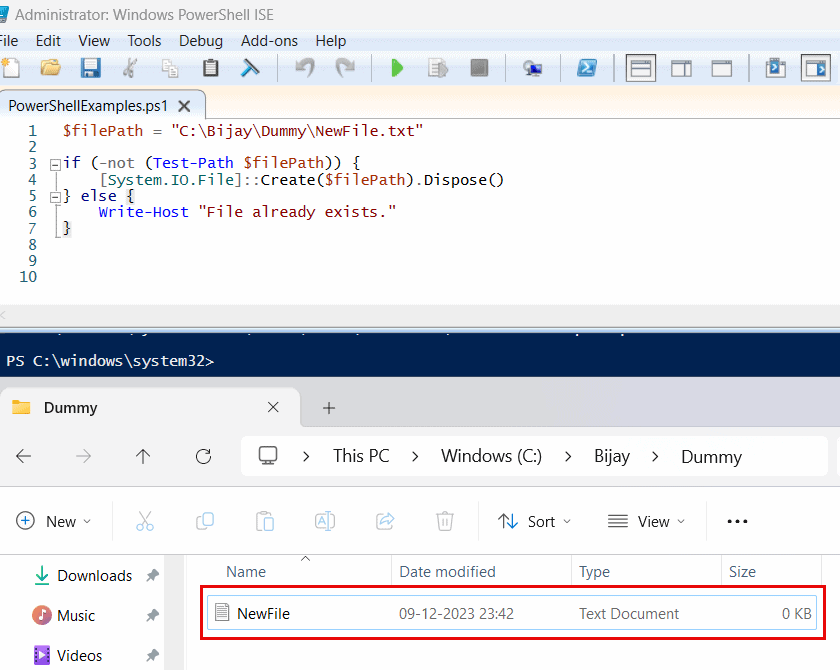
Conclusion
Each method can be used to create a file in PowerShell only if it does not already exist. The choice of method depends on the specific requirements of your script, such as whether you need to add content to the new file or simply create an empty file. In this tutorial, I have explained different methods: “PowerShell create file if not exists.“
You may also like:
- PowerShell create folder if not exists
- PowerShell Copy Item examples
- PowerShell Create Log File
- How to loop through a PowerShell array
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com