In PowerShell, you can loop through an array using several methods, including, foreach
, for
, and ForEach-Object
. I will show you how to loop through a PowerShell array using various methods in this PowerShell tutorial.
PowerShell loop through array
There are various methods to loop through an array in PowerShell.
1. Using the foreach Loop
The foreach loop is one of the most common ways to iterate through an array in PowerShell. It allows you to execute a block of code for each item in the array.
Here’s a basic example of how to enumerate an array in PowerShell.
$stringArray = @("New York", "Chicago", "Dallas")
foreach ($item in $stringArray) {
Write-Host "City name: $item"
}
In this script, $stringArray is an array of strings. The foreach loop goes through each string in the array and prints it out with Write-Host.
Output:
City name: New York
City name: Chicago
City name: Dallas
Once you run the PowerShell script, you can see the output in the below screenshot.
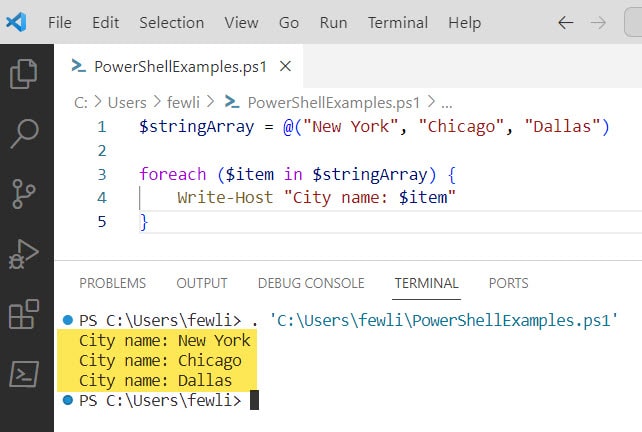
2. Using the for Loop
The for loop provides a more traditional looping mechanism, which is similar to what you’d find in languages like C# or Java.
Here is an example of how to loop through an array of elements in PowerShell using a for loop.
$stringArray = @("New York", "Chicago", "Dallas")
for ($i = 0; $i -lt $stringArray.Length; $i++) {
Write-Host "City: $($stringArray[$i])"
}
This script uses a for loop to iterate through each index of the array and access the string at that index.
Once you run the PowerShell script, you can see the output in the below screenshot.
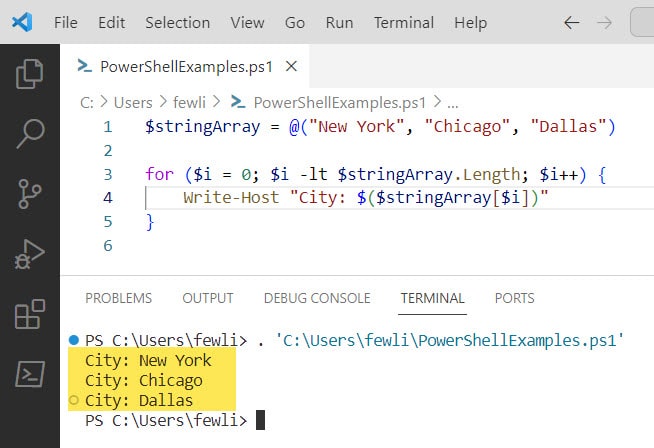
3. Using ForEach-Object
The ForEach-Object cmdlet provides a way to iterate an array of strings in PowerShell. Here is an example of PowerShell foreach array of strings.
$stringArray = @("New York", "Chicago", "Dallas")
$stringArray | ForEach-Object {
Write-Host "City: $_"
}
In this example, $_ represents the current object in the pipeline, which, in this case, is each string in the array in PowerShell. Check out the screenshot below for the output.
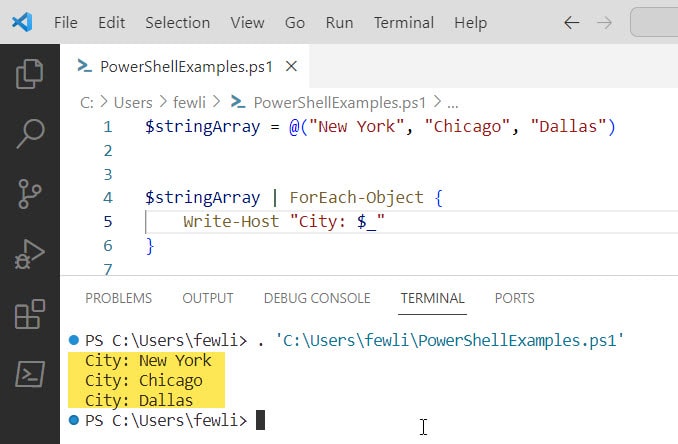
PowerShell Foreach Array of Strings
When dealing with an array of strings in PowerShell, the foreach
loop is particularly straightforward and readable. Here’s a more detailed example:
$names = @("Alice", "Bob", "Charlie")
foreach ($name in $names) {
Write-Host "Hello, $name!"
}
This script will output a personalized greeting for each name in the array.
PowerShell Loop Through Array of Strings
You can combine the loop with other PowerShell commands to loop through an array of strings and perform more complex operations. Here is a complete example.
Example:
$files = @("document.docx", "presentation.pptx", "spreadsheet.xlsx")
foreach ($file in $files) {
if ($file -like "*.docx") {
Write-Host "Word document: $file"
} elseif ($file -like "*.pptx") {
Write-Host "PowerPoint presentation: $file"
} elseif ($file -like "*.xlsx") {
Write-Host "Excel spreadsheet: $file"
}
}
This is how to loop through an array of strings in PowerShell.
Conclusion
Looping through arrays in PowerShell can be done using various methods, each suitable for different scenarios. The foreach
loop is simple and effective for most use cases, especially when dealing with arrays of strings. The for
loop provides more control over the iteration process and ForEach-Object
is useful when working with pipelines.
I have explained how to Loop Through a PowerShell Array using various methods in this PowerShell tutorial.
You may also like:
- PowerShell ArrayList
- PowerShell Array Tutorial
- Powershell Global Variable
- How to Replace a String in an Array Using PowerShell?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com