Do you want to know about PowerShell Arraylist? In this PowerShell tutorial, I will explain what is an arraylist in PowerShell? How to create and use an ArrayList in PowerShell. Also, we will see how to sort an ArrayList, remove items from an ArrayList, how to sort an ArrayList, and how to create an ArrayList from an array in PowerShell.
An ArrayList in PowerShell is a dynamic object that comes from the .NET Framework. It allows you to add, remove, and sort items in a collection. The key feature of an ArrayList is its ability to automatically resize, which is particularly useful when you’re dealing with an unknown or variable number of elements.
What is PowerShell ArrayList?
An ArrayList in PowerShell is a dynamic array, which means it can grow and shrink in size as needed. Unlike a standard array in PowerShell, which has a fixed size once it is created, an ArrayList can be modified by adding or removing items without creating a new one. This flexibility makes it a popular choice for scenarios where the number of elements in a collection is unknown or changes over time.
One difference between an array and an ArrayList is that an array is strongly types, which means an array can store only specific type elements; on the other hand, an ArrayList can store all the datatype values in PowerShell.
Create ArrayList in PowerShell
Now, we will see how to create an ArrayList in PowerShell.
To create a PowerShell ArrayList
, you can use the New-Object
cmdlet with the System.Collections.ArrayList
type. Here’s a simple example of how to create an empty ArrayList
:
$arrayList = New-Object System.Collections.ArrayList
or
You can create a PowerShell array list like the one below:
$demoarrayList = New-Object -TypeName 'System.Collections.ArrayList';
or
$demoarrayList = [System.Collections.ArrayList]::new()
This way, we can create an ArrayList of object types.
You can see this by calling the GetType() method.
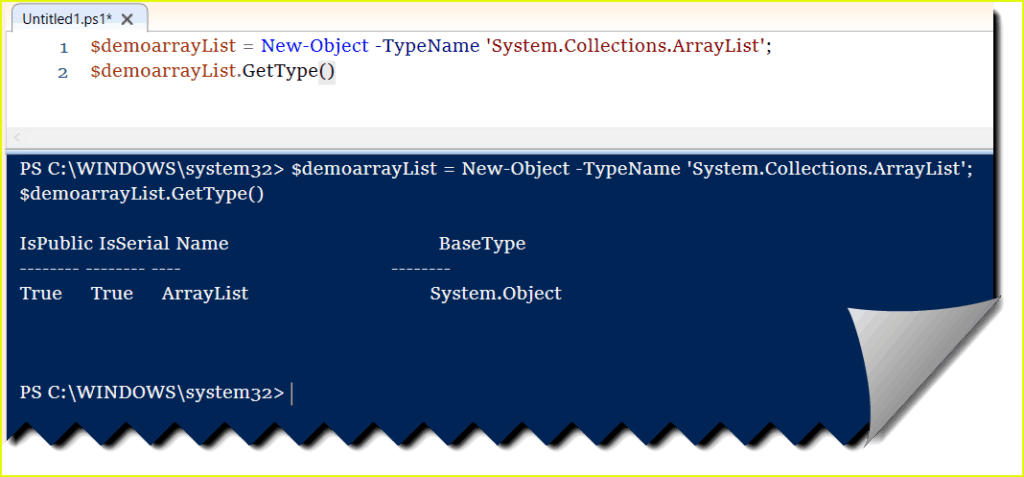
PowerShell Create an empty ArrayList
To create an empty ArrayList in PowerShell, you can write like below:
[System.Collections.ArrayList]$demoArrayList= @()
This will create an empty PowerShell ArrayList.
Creating an empty ArrayList in PowerShell is straightforward. You can use the New-Object
cmdlet to instantiate a new ArrayList object from the System.Collections
namespace. Here’s how you do it:
# Create an empty ArrayList
$arrayList = New-Object System.Collections.ArrayList
This command creates a new ArrayList that is empty. You can confirm that the ArrayList is empty and ready to use by checking its Count
property, which should return 0:
# Display the count of items in the ArrayList
$arrayList.Count
Once you run the PowerShell script using Visual Studio code, you can see the output like the below screenshot.
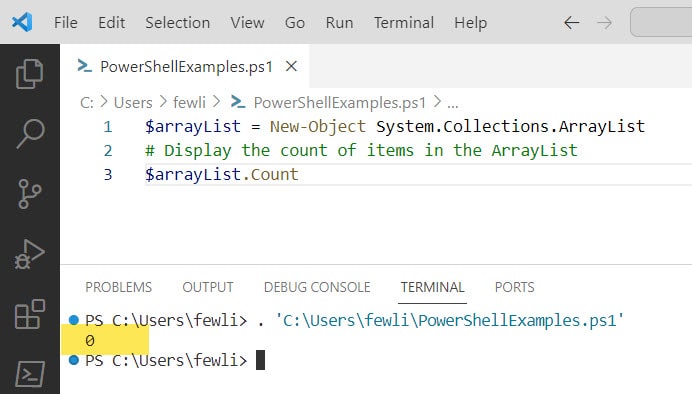
After creating an empty ArrayList, you can add items to it using the Add
method. Here’s an example of adding a few items:
# Add items to the ArrayList
$arrayList.Add('PowerShell')
$arrayList.Add(123)
$arrayList.Add($true)
Each time you add an item, the Add
method returns the index at which the item was added. If you don’t need this information, you can suppress the output by piping it to Out-Null
or casting it to [void]
:
# Add an item and suppress the return value
$arrayList.Add('New Item') | Out-Null
# or
[void]$arrayList.Add('Another New Item')
Add Items to PowerShell ArrayList
Let us see how to add items to a PowerShell arraylist.
Once you have an ArrayList
in PowerShell, you can add items to it using the Add
method. For example:
$arrayList.Add('Item1')
$arrayList.Add('Item2')
$arrayList.Add('Item3')
Each Add
method call returns the index at which the item was added, which can be useful in certain scenarios but is often ignored.
How to Get PowerShell ArrayList Item Count
We can count the items from the ArrayList by using the Count property.
$arrayList = New-Object System.Collections.ArrayList;
# Add items to the ArrayList
$arrayList.Add('PowerShell')
$arrayList.Add(123)
$arrayList.Add($true)
$arrayList.Count
Sort ArrayList Items
We can easily sort arraylist using sort-object in PowerShell.
$arrayList = New-Object System.Collections.ArrayList;
# Add items to the ArrayList
$arrayList.Add('PowerShell')
$arrayList.Add(123)
$arrayList.Add($true)
$arrayList | sort-object
You can see now the Arraylist is displaying in sorted order like below:
This is how to sort an ArrayList in PowerShell.
PowerShell arraylist foreach
Now, we will see how to iterate ArrayList by using foreach.
Syntax:
foreach (item in itemCollection){ item }
We can use ArrayList foreach like below:
$arrayList = New-Object System.Collections.ArrayList;
# Add items to the ArrayList
$arrayList.Add('PowerShell')
$arrayList.Add(123)
$arrayList.Add($true)
foreach ($item in $arrayList){ $item }
Once you run the PowerShell script, you can see the output below:
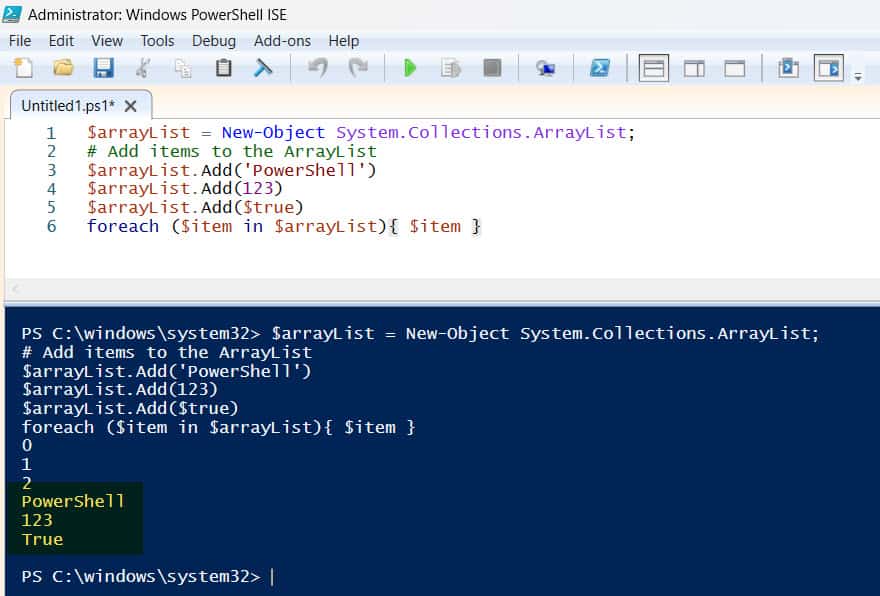
How to create an ArrayList from an Array in PowerShell?
Now, we will see how to create an ArrayList from an array. Below are two ways we can create an ArrayList from an array in PowerShell.
$X=2,4,6,8,9,20,5
[System.Collections.ArrayList]$X
$X=2,4,6,8,9,20,5
$y=[System.Collections.ArrayList]$X
You can see the output like below:
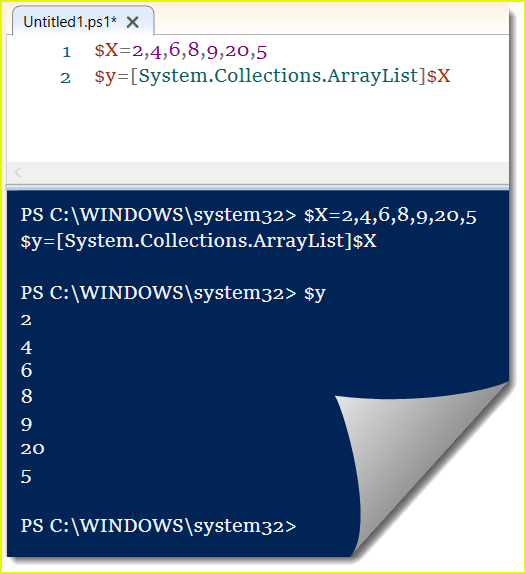
Below is also another way we can create an ArrayList from an array by using the below script:
$X=2,4,6,8,9,20,5
$y= New-Object System.Collections.ArrayList(,$X)
Remove item from ArrayList PowerShell
Now, we will see how to remove items from ArrayList in PowerShell.
We can use the RemoveAt() method to remove an item from the ArrayList.
$X=2,4,6,8,9,20,5
$y=[System.Collections.ArrayList]$X
$y.RemoveAt(2)
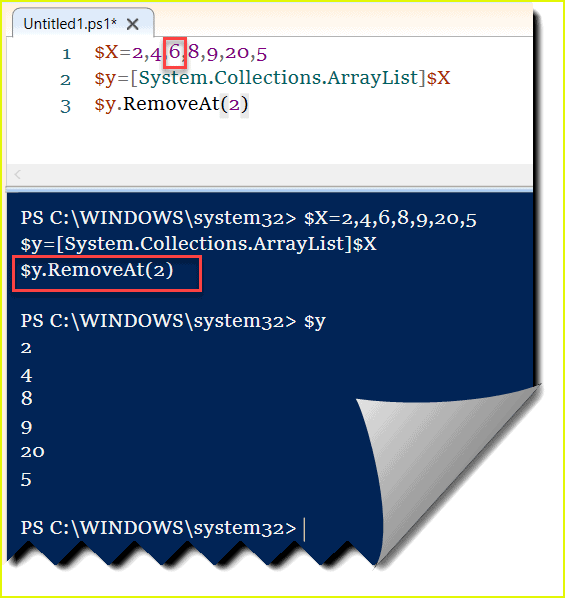
Remove multiple items from ArrayList
Now, we will see how to remove multiple items from PowerShell ArrayList by using the RemoveRange() method.
$X=2,4,6,8,9,20,5
$y=[System.Collections.ArrayList]$X
$y.RemoveRange(1,2)
RemoveRange takes two parameters: the first parameter is the Index, and the second parameter is the Count.
The above code removes 2 items starting from index 1. So it will remove 4,6 from the arraylist.
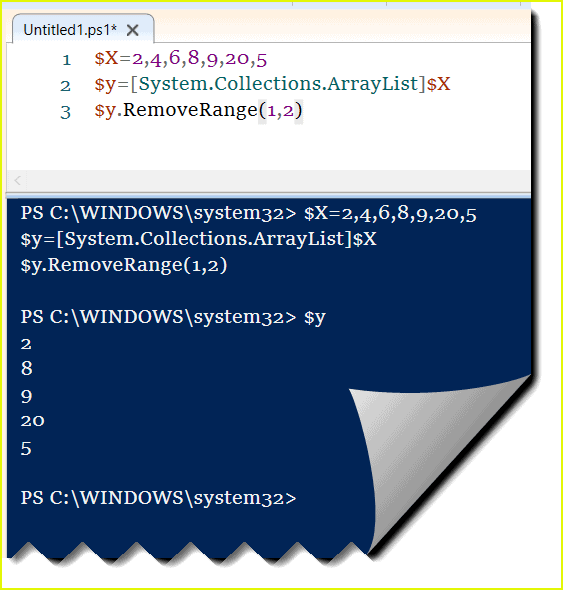
This is how to remove multiple items from an array list.
Conclusion
In this PowerShell tutorial, I will explain what is an ArrayList in PowerShell. And then I have explained how to create an empty array in PowerShell.
You may also like:
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com