You might get various requirements to import csv into an array in PowerShell. This is going to be a complete tutorial on how to read CSV into an array in PowerShell.
Read CSV into an Array in PowerShell
PowerShell provides Import-Csv cmdlet to read CSV files and import the data into PowerShell as an array of custom objects. Each row in the CSV file becomes an object, and the columns become the properties of those objects.
Here’s the basic syntax:
$data = Import-Csv -Path "C:\yourfile.csv"
The above command reads the CSV file located at “C:\yourfile.csv” and stores the data in the $data
variable as an array of objects.
Suppose we have a CSV file named users.csv
with the following content:
Name,Email,Department
John Doe,johndoe@example.com,IT
Jane Smith,janesmith@example.com,HR
You can see the CSV file also.
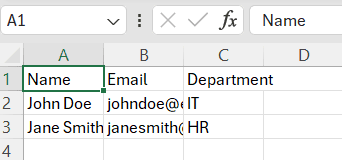
To read this CSV file into an array using PowerShell, we use the following command:
$users = Import-Csv -Path "C:\MyFolder\users.csv"
Now, $users
is an array where each element is an object representing a row in the CSV file. You can access the properties like this:
$users = Import-Csv -Path "C:\MyFolder\users.csv"
foreach ($user in $users) {
Write-Host "Name: $($user.Name), Email: $($user.Email), Department: $($user.Department)"
}
This loop will output like below in the screenshot, after I executed the PowerShell script using VS code.
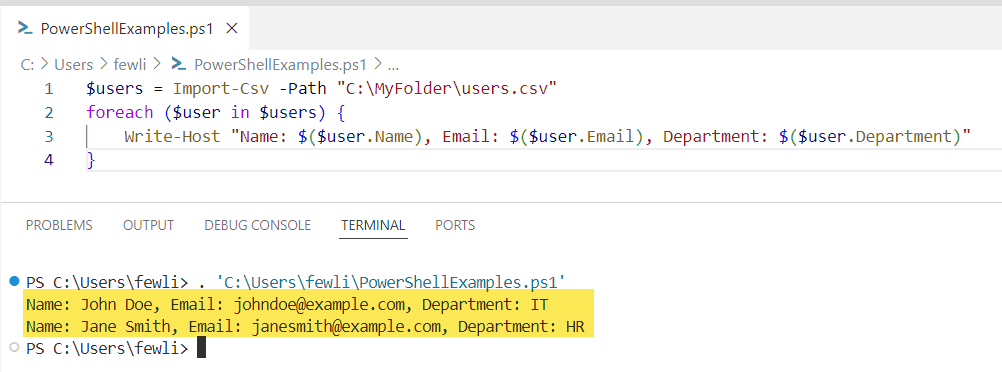
Accessing CSV Data as Arrays
Sometimes, you may want to work with the data from a specific column as a simple array. For example, if you want to get a list of all email addresses, you can use the Select-Object
cmdlet in combination with Import-Csv
:
$emailAddresses = Import-Csv -Path "C:\MyFolder\users.csv" | Select-Object -ExpandProperty Email
Now, $emailAddresses
is a standard array of strings containing just the email addresses from the CSV file.
Modify CSV Data
You can also modify the data in the array and then export it back to a CSV file using the Export-Csv
cmdlet. For instance, if we want to add a new user to our array and then save it back to the CSV file, we would do the following:
$newUser = [PSCustomObject]@{
Name = "Alice Johnson"
Email = "alicejohnson@example.com"
Department = "Marketing"
}
$users += $newUser
$users | Export-Csv -Path "C:\MyFolder\users.csv" -NoTypeInformation
This will add the new user to the users.csv
file without including the type of information that PowerShell usually adds at the top of the CSV file.
Conclusion
I hope now you have an idea of how to import csv to an array in PowerShell using the Import-Csv cmdlet. We saw here how to read a csv file into an array in PowerShell with this example.
You may also like the following PowerShell tutorials:
- How to Add Quotes in PowerShell
- Get Microsoft 365 Group Members Using PowerShell
- How to Use PowerShell Foreach With Examples
- How to Add Comments in PowerShell
- How to Convert String to Integer in PowerShell?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com
Hello, Bijay.
Would you please help provide a sample powershell script “how to create Hashtable from CSV file”. Looking forward to hearing from you. Thanks.
PS I have learned a lot from your blog “how to create an array from CSV file”.
you left out the most useful usecase! How do you find the value for a given varialbe. Using your example how do you display the salary for padmini