It is always good to add comments in your PowerShell script. In this PowerShell tutorial, I will explain how to add comments in PowerShell.
Comments in PowerShell
Adding comments within PowerShell scripts is crucial for documentation, providing clarity on the purpose and functionality of the code to future readers, which may include the original author or other programmers. Comments in PowerShell follow a simple yet universal syntax that is common to many programming languages, employing the hash symbol (#) to mark the commencement of a single-line comment. This notation signals to the interpreter that the text following the hash until the end of the line should not be executed.
The utility of comments extends beyond mere annotations. They serve to elucidate complex logic, describe the usage of functions and parameters, and guide the user through the flow of the script. PowerShell provides a multi-line comment syntax for more extensive explanations or to temporarily disable blocks of code for debugging purposes. Enclosed by <# and #> symbols, these block comments allow for the inclusion of larger sections of text without the need for a hash at the beginning of each line.
PowerShell’s comment system also supports a built-in help feature known as comment-based help. This allows the script to include comprehensive documentation that details the functionality, parameters, examples, and additional information directly within the script. By adhering to the prescribed format, these blocks of comments become a part of the help system, accessible whenever a user invokes the help commands.
Types of Comments in PowerShell
PowerShell supports two primary types of comments for annotating scripts: single line comments and block comments. Single line comments use the hash symbol, while block comments are ideal for longer annotations spanning multiple lines.
Single Line Comments
A single line comment in PowerShell is created by using the hash symbol (#
). Any text following this symbol on the same line is treated as a comment and is ignored by the PowerShell interpreter. These comments are typically used to explain individual lines of code or to temporarily disable code for testing purposes.
# This is a single line comment
Get-Service # This comments follows a line of code
One can also use blank lines for readability along with line comments to separate code sections.
Block Comments or multiline comments
PowerShell block comments, or multiline comments, start with <#
and end with #>
. These are used for longer descriptions, which explain complex sections of code or provide documentation at the beginning of scripts. Block comments can span multiple lines, allowing for extensive explanations without the need for the #
symbol on each line.
<#
This is a block comment in PowerShell.
It can span multiple lines.
#>
Get-Process
You can see in the screenshot below how I have added comments in PowerShell.
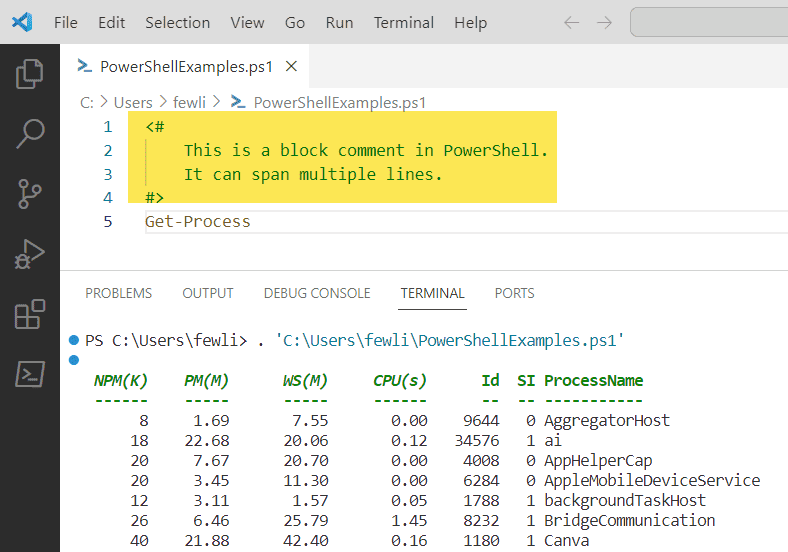
Add Comments in PowerShell Scripts
Adding comments to PowerShell scripts is essential for maintaining their readability and simplifying the debugging process. It enables developers to create self-documenting code that is easier to understand and maintain.
Commenting Code for Debugging
When debugging PowerShell scripts, a programmer often needs to isolate sections of code to pinpoint errors. Single-line comments are marked with the #
symbol, and PowerShell ignores everything to the right of it on the same line. For example:
# This is a single-line comment
Get-Process
To comment out multiple lines or a block of code, PowerShell uses <# #>
syntax:
<#
This is a multi-line comment block.
Multiple lines of code can be commented out for debugging.
#>
Get-Service
The block comment is particularly helpful when large sections of a script require temporary removal from execution during troubleshooting phases.
Commenting for Code Clarity
For code clarity, adding descriptive comments helps to explain the purpose and logic behind certain lines of code or scripts as a whole. Comments are a form of self-documenting code, making it easier for others to understand and ensuring that the developer’s intent is clear. A self-explanatory comment might look like this:
# Check if the user is an administrator
$user = "AdminUser"
PowerShell scripts often include complex logic, where it becomes crucial to explain the reasoning behind a particular line of code. Use concise and informative comments to clarify the purpose of code segments without overwhelming the reader with information. For instance:
# Calculate disk space and report if less than threshold
$diskSpace = Get-PSDrive C
if ($diskSpace.Free -lt 1024MB) {
Write-Warning "Low disk space on C drive"
}
Through judicious commenting, developers ensure their code is accessible and maintainable by others in the team or by future maintainers.
PowerShell Comment Based Help
In PowerShell, comment-based help provides a structured way to document functions and cmdlets directly in the script, allowing users to retrieve information using the Get-Help
cmdlet.
Writing Comment Based Help
To write comment-based help in PowerShell, one begins with a series of annotated comments that precede a function or a cmdlet definition. Two distinct syntax options are available:
- Using the hash symbol: A hash (
#
) precedes each line of the help content. - Using comment blocks: One can encapsulate the help content within
<#
and#>
to create a comment block.
Example of hash symbol usage:
# .SYNOPSIS
# Brief description of the function/cmdlet.
Example of comment block usage:
<#
.SYNOPSIS
Brief description of the function/cmdlet.
#>
Keywords for Comment Based Help
Comment-based help may include certain predefined keywords that categorize the help content. PowerShell processes these keywords to organize and display help information effectively when the Get-Help
cmdlet is called.
Here is a list of some common help keywords and their purposes:
- .SYNOPSIS: A brief description of the function or cmdlet.
- .DESCRIPTION: A more detailed explanation.
- .PARAMETER name: Details about a particular parameter.
- .EXAMPLE: An example of how to use the function or cmdlet.
- .NOTES: Additional information relevant to the user’s understanding.
- .LINK: References to online documentation or related commands.
Syntax for using keywords:
Each keyword is preceded by a period (.) followed by the keyword name, and the related content comes on the following lines.
<#
.SYNOPSIS
Adds a file name extension to a supplied name.
.DESCRIPTION
This function takes a file name and appends the specified file extension.
.PARAMETER Name
The name of the file to which the extension will be added.
.PARAMETER Extension
The type of file extension to add, defaults to 'txt'.
.EXAMPLE
PS> Add-Extension -Name "Report" -Extension "docx"
#>
Using comment-based help is a best practice that enhances the maintainability and usability of scripts by providing in-line documentation for troubleshooting and understanding script behavior without diving into the code itself.
Create External Help Files in PowerShell
In PowerShell, creating external help files separates in-depth documentation from script code, offering users a more accessible help system that aligns with larger, complex modules.
XML-based Help
An external help file in PowerShell is typically an XML-based file adhering to the Microsoft Assistance Markup Language (MAML) schema. It facilitates the creation of structured help content, which PowerShell can interpret and display via the Get-Help
cmdlet.
To connect a script to an XML-based help file, one must include the .ExternalHelp
directive in the script’s comment-based help section. For example:
<# .ExternalHelp myscripts\Add-Extension.xml #>
XML-based help files must have a corresponding filename indicated in the external help keyword, ensuring Get-Help
can retrieve and display the help content when prompted.
About External Help Files
When a user creates an external help file, it serves as a centralized and detailed source of help for one or multiple scripts or functions. Within these files, sections such as detailed descriptions, parameter explanations, and examples are formatted in XML to provide users with a comprehensive help experience.
Here’s an example for a function that adds a file name extension:
<?xml version="1.0" encoding="utf-8"?>
<helpItems xmlns="http://msh" schema="maml">
<command:command xmlns:command="http://schemas.microsoft.com/maml/dev/command/2004/10">
<command:details>
<command:name>Add-Extension</command:name>
...
</command:details>
<command:description>
<maml:para>Adds a file name extension to a supplied name.</maml:para>
</command:description>
...
</command:command>
</helpItems>
The file name for the help file is typically FunctionName.xml
, for instance, Add-Extension.xml
for the Add-Extension
function. Users should ensure that the help file is located in a path accessible by PowerShell and specified correctly with the .ExternalHelp
keyword inside their script.
Annotations in PowerShell
In PowerShell, annotations are essential for documenting scripts. They provide clarity on the script’s purpose, usage, parameters, and expected outcomes, which is vital for both the creator and other users who may interact with the script.
Leveraging Annotations
Annotations in PowerShell are utilized to describe how a script functions, outline the expected inputs and outputs, and provide examples of the script in use. These annotations are not only critical for code maintenance and readability but also support the built-in help system that PowerShell provides.
- .Synopsis: Gives a brief summary of the script’s functionality.
- .Description: Elaborates on the details of what the script does.
- .Example: Demonstrates how the script is called and used.
- .Notes: Offers additional information about the script, such as the author’s name, any known issues, or changelog.
- .Inputs: Describes what inputs, if any, the script can accept.
- .Outputs: Explains what the script will output after execution.
Proper use of these annotations ensures that anyone can understand the script’s purpose and how to use it effectively without delving deep into the code.
Annotation Syntax
Properly formatted annotations are crucial for the Get-Help
cmdlet in PowerShell to parse and display help content to users. The syntax of annotations in PowerShell is characterized by comment blocks that precede the script’s executable code.
- Single-Line Comments: Start with a
#
symbol, and everything to the right is considered part of the comment.
# This is a single-line comment
Multi-Line Comments: Enclosed within <#
and #>
symbols, typically used for longer descriptions and help content.
<#
.SYNOPSIS
This script performs a network check.
.DESCRIPTION
The script checks the connectivity of a specified IP address.
.EXAMPLE
PS C:\> .\Check-Network.ps1 192.168.1.1
#>
Each annotation keyword in a comment block aligns with the entities that provide structured information. The comment-based help system recognizes specific keywords with a period prefix, such as .Synopsis
, .Description
, and .Example
, to structure the help information for Get-Help
.
Correct annotation syntax ensures that when other users use Get-Help
to query a script, they receive a comprehensive overview with all the pertinent sections, including a name, synopsis, description, examples, notes, input, and output descriptions.
Working with PowerShell Cmdlets and Comments
In PowerShell, combining cmdlets with comments enhances script clarity and maintainability. Commenting involves placing a ‘#’ symbol at the start of a line or appending it after a cmdlet. This marks the text as a comment, which PowerShell ignores during execution.
One can document the functionality of Get-Service
, which retrieves a service’s status, by placing a comment above the cmdlet:
# Retrieve the current status of the Print Spooler service
Get-Service -Name "Spooler"
Similarly, for setting an item’s value with Set-Item
, it is good practice to explain the action:
# Change the value of a registry key
Set-Item -Path "HKCU:\Software\MyCompany\Preferences" -Value "DarkMode"
Stopping a service using Stop-Service
benefits from a preceding comment indicating the purpose or the reason for stopping said service:
# Stop the unnecessary background service to free resources
Stop-Service -Name "BackgroundTransferService"
When using Get-Content -Path
, authors may place a comment to clarify the intention behind reading a file:
# Read content from a configuration file
Get-Content -Path "C:\config\settings.ini"
Table of Best Practices for Comment use with Cmdlets
Cmdlet | Purpose | Example Comment |
---|---|---|
Get-Service | Retrieve service status | # Get status of the Print Spooler service |
Set-Item | Modify a registry key value | # Update user preferences for application UI |
Stop-Service | Terminate a running service | # Discontinue an unnecessary service |
Get-Content | Read data from a file | # Load the application configuration settings |
Using comments alongside cmdlets significantly aids in the comprehension of script actions, providing insight into what each piece of the script is intended to achieve. This is especially beneficial when scripts are revisited after time or shared with other users.
Working with PowerShell ISE and Comments
When working with Windows PowerShell ISE, an integrated scripting environment, users have various tools to streamline coding and comment management. Efficiently leveraging features like Visual Studio Code integration and keyboard shortcuts can greatly enhance a developer’s productivity.
Visual Studio Code Integration
Visual Studio Code (VS Code) is a versatile editor that supports PowerShell development through its powerful extension, PowerShell Integrated Scripting Environment (ISE) mode, which provides an experience similar to Windows PowerShell ISE.
This mode allows users access an enriched environment with IntelliSense, code navigation, and integrated version control. To integrate PowerShell ISE with VS Code, one must ensure the PowerShell extension is installed, after which ISE mode can be enabled for an experience that supports PowerShell commenting and scripting.
- Steps to Enable ISE Mode in VS Code:
- Open Command Palette (
Ctrl+Shift+P
) - Type “Enable ISE Mode”
- Select
PowerShell: Enable ISE Mode
- Open Command Palette (
Using Keyboard Shortcuts
Keyboard shortcuts in PowerShell ISE simplify the process of adding or removing comments. A single-line comment in PowerShell starts with the #
symbol. Multi-line comment blocks can be toggled by enclosing text within <# #>
.
PowerShell ISE users can utilize pre-made or custom functions that can be added to the $Profile
file to automate the commenting process with shortcuts.
- Default Keyboard Shortcuts:
- Comment a line:
Ctrl+K Ctrl+C
- Uncomment a line:
Ctrl+K Ctrl+U
- Comment a line:
Developers can customize these shortcuts or use a function to toggle comments by editing their PowerShell $Profile
. By placing a predefined function in the profile, comments can be managed with custom key bindings, enhancing the efficiency of working within scripts. Users need to know the specifics of creating and editing the $Profile for these customizations to take effect.
PowerShell Comment Shortcut
In PowerShell Integrated Scripting Environment (ISE), users have the capability to comment or uncomment lines of code efficiently using shortcuts.
Single Line Commenting: To comment out a single line, the user can place the cursor on the line they wish to comment on and use the following shortcut:
- Ctrl + J or Ctrl + K, Ctrl + C
This adds a #
at the beginning of the line, turning it into a comment.
Block Commenting: For multiple lines, block commenting can be applied with the following approach:
- Select the lines to be commented.
- Apply the shortcut Ctrl + K, Ctrl + C to comment the block.
Uncommenting Code: To revert commented code back into executable script lines, the following shortcut is used:
- For either a single line or selected block, Ctrl + K, Ctrl + U will remove the preceding
#
symbol or<#...#>
block, uncommenting the code.
It is important to note that these shortcuts assist in creating and removing comments quickly, enhancing the scripting workflow. Moreover, when sharing scripts, comments are invaluable for explaining the purpose and functionality of the code—helping both the author and other users understand the intent and mechanism of the script properly.
Remember, these shortcuts are specific to PowerShell ISE and may vary in other editors or environments. It’s always beneficial to refer to the specific documentation or help resources of the environment one is using for accurate shortcuts and procedures.
Advanced Comment Utilization
When scripting in PowerShell, leveraging advanced commenting techniques is essential for creating comprehensive documentation and managing output effectively. Two aspects critical to mastery in PowerShell are writing verbose help and intelligently commenting outputs.
Writing Verbose Help
Verbose help in PowerShell is a detailed way of documenting functions and scripts that goes beyond simple line comments. A user can create verbose help using comment-based help syntax that allows for multiple help topics such as descriptions, parameter explanations, and usage examples.
<#
.SYNOPSIS
A brief description of the function or script.
.DESCRIPTION
A more detailed explanation of the function or script.
.PARAMETER Name
Description of the parameter's purpose.
.EXAMPLE
An example of how to use the function or script.
.NOTES
Additional information about the script.
#>
This structured approach enables one to generate help files that are accessible using the Get-Help
cmdlet, providing a robust in-console help experience.
Commenting Outputs
Intelligent commenting on outputs involves documenting the purpose and potential results of output commands within a script. PowerShell offers different cmdlets to handle outputs, which can be narrated for clarity:
Write-Host: This cmdlet is useful for printing outputs directly to the console. It should be documented to explain what information is being displayed and why.
# Write the number of files to the console
Write-Host "There are $fileCount files in the directory."
Out-File: When outputs are being redirected to a file, comments should describe the destination path, file format, and reason for exporting data.
# Save the list of user profiles to a text file
$userProfiles | Out-File -FilePath "C:\Output\UserProfiles.txt"
It is important to remember that while Write-Host
is fine for quick displays of information, its outputs are not capturable in variables or through piping. One should consider using Write-Output
for a return value that can be further processed. Comments should clearly state if direct display is the intention to avoid confusion.
Managing Help Content
Proper management of help content in PowerShell involves leveraging built-in features to enhance the understanding of cmdlets. Users can utilize the Get-Help
cmdlet with parameters to access comprehensive documentation.
Using -Full and -Online
When a user requires detailed information about a cmdlet, they can append the -Full
parameter to the Get-Help
cmdlet. This action retrieves a complete help file that includes the syntax, parameters, examples, and additional notes.
Example:
Get-Help Get-ChildItem -Full
Alternatively, if they prefer the most updated documentation available online, they can use the -Online
parameter. This parameter opens the default web browser to the Microsoft Docs page for the respective cmdlet.
Example:
Get-Help Get-ChildItem -Online
Help Content for Specific Cmdlets
Specific cmdlets’ help content can be enhanced by including .LINK
in the comment-based help section of the script or function. .LINK
directs to additional resources or related documentation, providing a pathway for further reading for the user.
<#
.SYNOPSIS
Short description of the function or script.
.DESCRIPTION
A longer description.
.LINK
URL to more resources or related commands
#>
In this way, users may append the .LINK
keyword followed by a URL or a reference to another help topic within their PowerShell script to offer external information or context.
Add Comments for Data Files in PowerShell
In PowerShell, comments within data files like CSV or log files provide context and enhance understanding for future users. These annotations play a crucial role in maintaining data files’ clarity and integrity.
Commenting CSV-based Files
To add comments to a CSV-based input or output file in PowerShell, one could utilize a #
symbol at the beginning of the line. Since CSV files are plain text, this will ensure the line is treated as a comment and not as a data entry. For example:
# This is a comment in a CSV file
"Name","Age","Location"
"John Doe","28","New York"
When processing CSV data, PowerShell’s Import-Csv
cmdlet can be used to read the file, and Export-Csv
to create an output file. By using the -OutVariable
or common parameters like -ErrorAction
, -ErrorVariable
, -WarningAction
, -WarningVariable
, and -OutBuffer
, users can manage the execution and output of their data handling scripts effectively.
Date and Time Stamps in Logs
Logs typically include date and time stamps to provide a temporal context to each entry, allowing users to track events and changes over time. In PowerShell, when appending data to a text-based log file, one can prepend the date and time to a comment or entry using the Get-Date
cmdlet:
# Add a date and time stamp to a log entry
"$(Get-Date) - The script started successfully" | Out-File -FilePath "script.log" -Append
Using the Out-File
cmdlet with the -Append
parameter, the script appends the comment directly to the .txt
log file, ensuring that the temporal order of events is maintained without overwriting the existing contents.
Add Comments in PowerShell Functions and Parameters
In PowerShell, adding comments to functions and parameters is crucial for creating self-documented scripts that are easy to understand and maintain. Proper commenting assists in explaining the purpose, usage, and mechanics of the code.
Documenting Functions
When documenting functions in PowerShell, one should utilize a comment block above the function declaration. This is enclosed in <# #>
. The function’s description, including its purpose, and usage details, should be detailed within this block. Below is an illustrative example:
<#
.SYNOPSIS
Brief description of the function.
.DESCRIPTION
A more detailed explanation of what the function does and its usage.
.EXAMPLE
An example of how to use the function.
.NOTES
Additional information about the function.
#>
function Get-ResourceStatus {
# Function code goes here
}
Parameter Descriptions
Each parameter within a PowerShell function can be documented using comment-based help. To effectively describe a parameter, one should provide details directly above the parameter declaration or within the function’s comment block under a .PARAMETER
tag. Descriptions should be clear and concise. Here, they enumerate the function’s parameters and describe what each expects:
<#
.PARAMETER ResourceId
The unique identifier for the resource that this function checks.
.PARAMETER TimeoutSeconds
The time, in seconds, to wait for a response before considering the check to have failed.
#>
function Get-ResourceStatus {
param(
[string]$ResourceId,
[int]$TimeoutSeconds = 20
)
# Function code goes here
}
Comments directly above the parameter declarations can also be used for individual inline documentation. This ensures that anyone reviewing the code has immediate visibility of the purpose for each parameter, enhancing the script’s readability.
PowerShell Comment Multiple Lines
When writing scripts in PowerShell, a user may need to incorporate comments that span multiple lines. PowerShell supports block comments, which are ideal for this purpose. Block comments are enclosed within <#
and #>
tags.
To begin a block comment, the user starts with the <#
tag, followed by the comment text on one or more lines, and concludes with the #>
tag.
Example of a Multi-Line Comment:
<#
This is an example of a
multi-line comment in PowerShell
#>
Multi-line comments are particularly useful for:
- Documenting Code: Describing what a complex script or function does.
- Commenting Out Code: Temporarily disabling code blocks during testing or debugging.
Here are the steps a user should follow to create multi-line comments:
- Type
<#
to start the comment block. - Press Enter to move to a new line.
- Add the descriptive text across as many lines as necessary.
- After the final line of commentary, close the block with
#>
.
Users should note that anything inside the multi-line comment block will not be executed by PowerShell. This feature allows for flexible documentation right within the code without affecting its functionality.
PowerShell Comment Example
PowerShell scripts often require comments to explain or clarify the purpose of certain lines of code or to temporarily disable code execution. One can insert comments in PowerShell using the hash symbol (#
) for single-line comments and <# #>
for block (multi-line) comments.
Single-Line Comments
The single-line comment in PowerShell is initiated by the #
symbol. Any text following #
on that line will not be executed.
# This is a single-line comment in PowerShell
Get-Process -Name notepad # This comment is after a line of code
The second example shows how to place a comment at the end of the executable code on the same line, which PowerShell will ignore during execution.
Multi-Line Comments
For comments that span multiple lines, PowerShell utilizes block comments, which start with <#
and end with #>
.
<#
This is an example of a block comment that can be extended
over multiple lines. It's particularly useful for larger descriptions
or when commenting out blocks of code during testing and debugging.
#>
Get-Service -Name "bits"
In this block comment example, multiple lines of text are encapsulated between <#
and #>
, and they are all ignored by PowerShell.
Note on Comment Usage
The developer should use comments thoughtfully to enhance the script’s readability without over-commenting, which can clutter the code. Clear and concise comments can significantly assist in understanding and maintaining the PowerShell script.
Conclusion
Comments in PowerShell scripts are critical for maintaining code clarity and manageability. They facilitate understanding and collaboration, allowing individuals to discern the purpose and functionality of the script at a glance. A well-commented script saves time for anyone who needs to modify, debug, or use the script in the future.
PowerShell supports two types of comments:
- Single-line comments: Initiated with the
#
symbol and continue until the end of the line. For example:
# This is a single-line comment
Get-ChildItem
- Multi-line comments: Enclosed within
<#
and#>
symbols and can span across multiple lines, useful for more extensive descriptions or block-commenting code sections. For instance:
<#
This is a multi-line comment
It can span multiple lines
#>
Get-Service
In this PowerShell tutorial, I have explained everything about how to add comments in PowerShell with various examples.
You may also like:
- Convert String to SecureString in PowerShell
- Replace String in XML File using PowerShell
- Get Unique Values from an Array in PowerShell
- Replace String Containing Double Quotes in PowerShell
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com