When working with data in PowerShell, you might be required to get distinct values from the array in PowerShell. In this tutorial, we’ll explore different methods to get unique values from an array in PowerShell with complete real examples.
To get unique values from an array in PowerShell, you can use the Select-Object
cmdlet with the -Unique
switch, which filters out duplicate values without the need to sort the array. For example, $uniqueArray = $array | Select-Object -Unique
will return an array containing only the unique elements from $array
. This method is simple and effective for arrays of both simple data types and objects with properties.
Get distinct values from an array in PowerShell
Now, let us check different methods to check how to get unique values from an array in PowerShell.
1. Using Get-Unique Cmdlet
The Get-Unique
cmdlet in PowerShell is designed to filter out duplicate values from a sorted list. Before you use Get-Unique
, it is important to sort the array because Get-Unique
only compares adjacent elements.
Here’s an example:
# Define an array with duplicate values
$array = 1,2,2,3,4,4,5
# Sort the array and then pipe it to Get-Unique
$uniqueArray = $array | Sort-Object | Get-Unique
# Display the unique array
$uniqueArray
The above PowerShell commands will output the unique values from the original array. Note that Sort-Object
is used to sort the array before piping it to Get-Unique
.
After I executed the code using Visual Studio Code, the output you can see in the screenshot below:
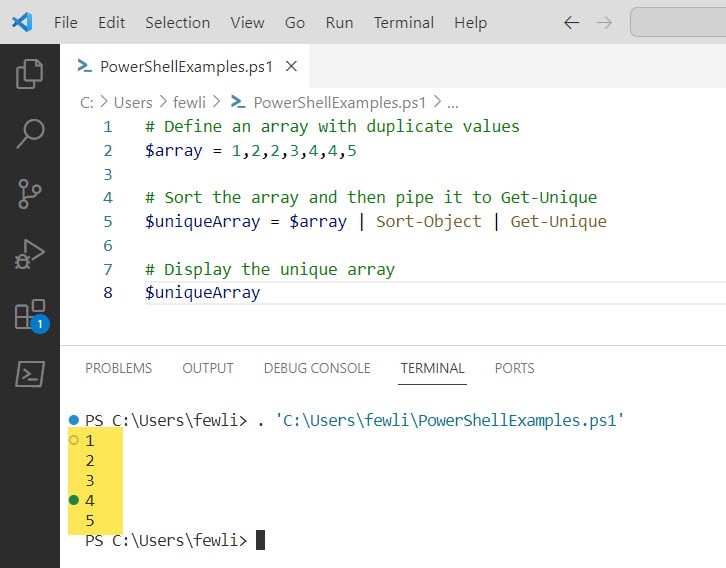
2. Using Select-Object with -Unique
Another method to get unique values from an array in PowerShell is to use Select-Object
with the -Unique
switch. This method is more straightforward because it does not require sorting the array first.
Here’s how you can use it:
# Define an array with duplicate values
$array = 1,2,3,4,5,5,6,7,8,9,0,0
# Use Select-Object with the -Unique switch
$uniqueArray = $array | Select-Object -Unique
# Display the unique array
$uniqueArray
This will immediately give you an array with unique values, as the -Unique switch filters out duplicates. You can see in the screenshot below I ran the code using VS code.
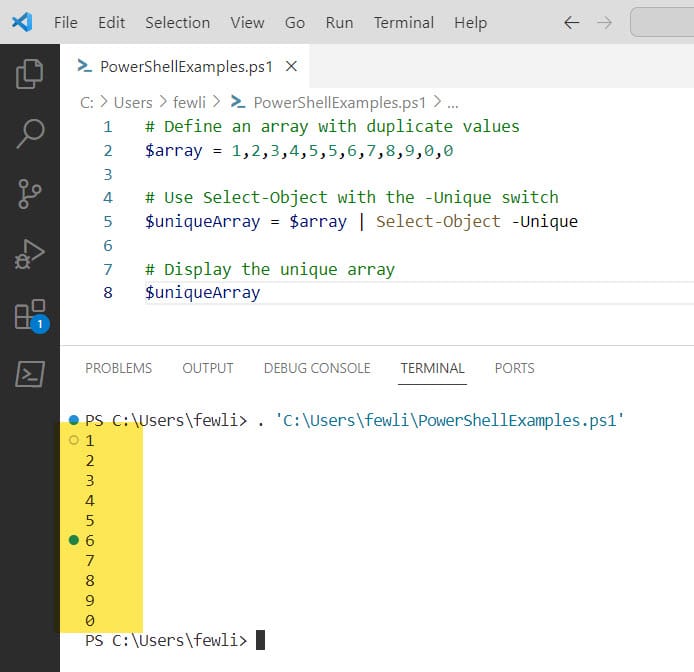
3. Custom Function for Unique Values
You might consider writing a custom function if you want more control over how duplicates are identified and removed. Here’s a simple example of a custom function that returns unique values from an array:
function Get-UniqueValues {
param([array]$InputArray)
# Create an empty array to store unique values
$uniqueValues = @()
foreach ($item in $InputArray) {
# If the item is not already in the unique values array, add it
if ($item -notin $uniqueValues) {
$uniqueValues += $item
}
}
# Return the array of unique values
return $uniqueValues
}
# Define an array with duplicates
$array = 1,2,2,3,4,4,5
# Call the custom function
$uniqueArray = Get-UniqueValues -InputArray $array
# Display the unique array
$uniqueArray
This function iterates through each item in the input array and adds it to a new array if it’s not already present, effectively filtering out duplicates.
4. Advanced Filtering for Unique Objects
Sometimes, you might be dealing with arrays of objects in PowerShell where you need to filter based on unique property values. In such cases, you can still use Select-Object
with the -Unique
switch, but you’ll need to specify the property.
For example, if you have an array of custom objects with a Name
property, you could get unique objects like this:
# Define an array of custom objects
$people = @(
[PSCustomObject]@{Name='John'; Age=25},
[PSCustomObject]@{Name='John'; Age=30},
[PSCustomObject]@{Name='Jane'; Age=25}
)
# Get unique objects by the Name property
$uniquePeople = $people | Select-Object -Unique -Property Name
# Display the unique people
$uniquePeople
This will return a list of people with unique names, ignoring duplicates. You can see the output in the screenshot below:
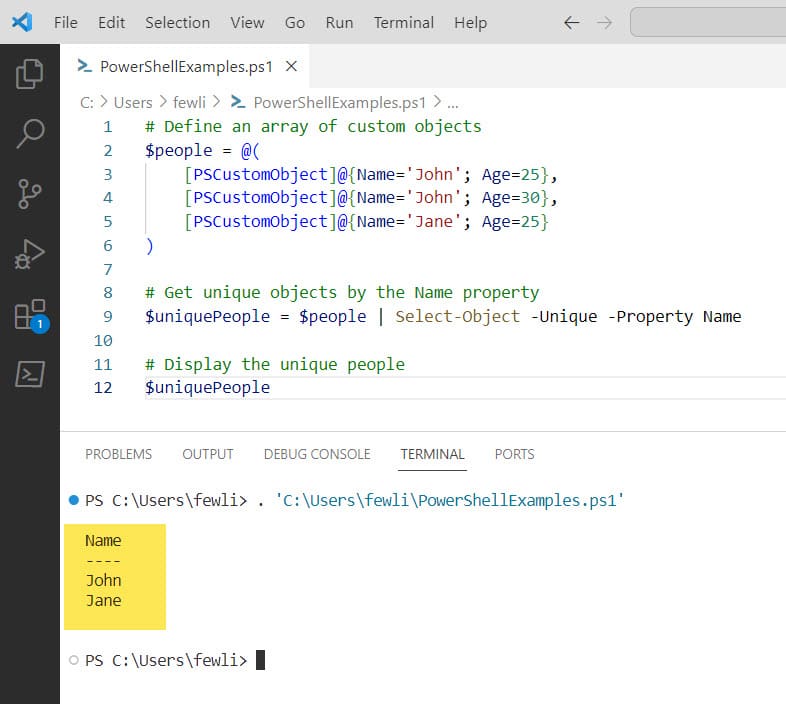
Conclusion
PowerShell offers several ways to get unique values from an array, whether you’re dealing with simple values or complex objects. The Get-Unique
cmdlet is useful for sorted lists, while Select-Object
with the -Unique
switch is a more direct approach that doesn’t require sorting. For more control, you can write a custom function or use advanced filtering techniques for objects.
I hope you understand these various methods to find unique values from an array in PowerShell or how to get distinct values from an array in PowerShell.
You may also like:
- PowerShell Function Array Parameters
- How to Replace a String in an Array Using PowerShell?
- How to Remove Empty Lines from an Array in PowerShell?
- How to Get the Highest Number in an Array in PowerShell?
- How To Sort Array Of Objects In PowerShell?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com