Recently, I got a requirement to get the highest number in an array in PowerShell. In this PowerShell tutorial, we’ll explore different methods to retrieve the highest number from an array in PowerShell with complete and real examples.
To find the highest number in an array in PowerShell, you can use the Measure-Object
cmdlet with the -Maximum
parameter. For example, $highestNumber = ($numbers | Measure-Object -Maximum).Maximum
will give you the highest value. Alternatively, you can sort the array and get the last element using $numbers | Sort-Object | Select-Object -Last 1
, or iterate through the array with a loop to compare each value.
PowerShell get highest number in array
An array is a collection of items that are accessed by a numerical index. In PowerShell, arrays can hold items of any type, including numbers, strings, and objects.
Here’s an example of how to create an array in PowerShell:
$numbers = 8, 23, 15, 42, 7, 34
Now. let’s look at different ways to find the highest number from an array in PowerShell.
Method 1: Using the Measure-Object
Cmdlet
The most straightforward method to find the highest number in an array is by using the Measure-Object
cmdlet in PowerShell. This cmdlet is designed to perform calculations on the properties of objects, and it can easily return the maximum value in a PowerShell array.
Here’s how to use it:
$numbers = 8, 23, 15, 42, 7, 34
$highestNumber = ($numbers | Measure-Object -Maximum).Maximum
Write-Host "The highest number in the array is: $highestNumber"
In this example, we pipe our $numbers
array into Measure-Object
, specifying the -Maximum
parameter. The .Maximum
property of the result object will hold the highest number.
You can see the output in the screenshot below after I executed the code using Visual Studio Code.
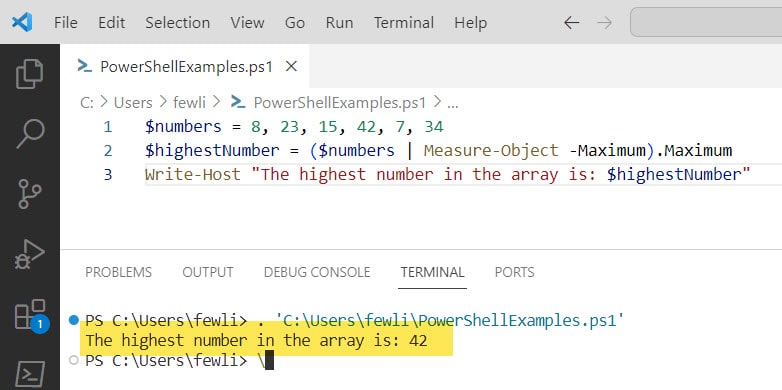
Method 2: Sorting the Array
Another way to find the highest number from a PowerShell array is to sort the array and then select the last item, which will be the largest number since the default sorting order is ascending.
Here’s an example:
$numbers = 8, 23, 15, 42, 7, 34
$sortedNumbers = $numbers | Sort-Object
$highestNumber = $sortedNumbers[-1]
Write-Host "The highest number in the array is: $highestNumber"
By using Sort-Object
, we sort the $numbers
array. We then access the last element using [-1]
, which is a shorthand for getting the last item of an array in PowerShell.
You can see the output after I executed the code using VS code.
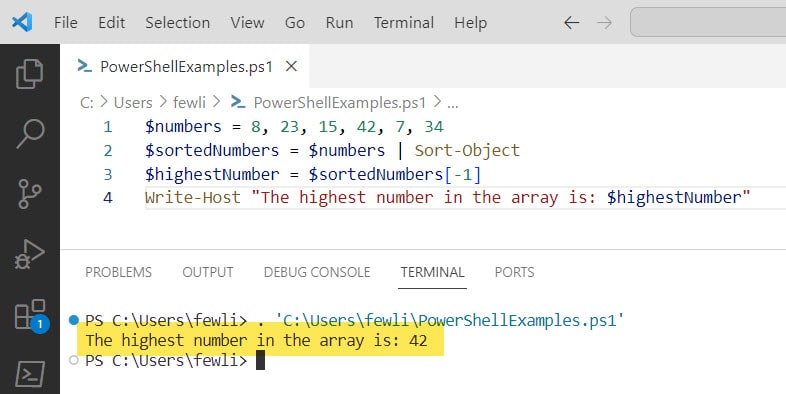
Method 3: Using a For Loop
You can use a for loop to iterate through each number and determine the highest one from the PowerShell array.
Here’s what that looks like:
$numbers = 8, 23, 15, 42, 7, 34
$highestNumber = $numbers[0]
foreach ($number in $numbers) {
if ($number -gt $highestNumber) {
$highestNumber = $number
}
}
Write-Host "The highest number in the array is: $highestNumber"
In this script, we assume the first number in the array is the highest. We then loop through each number, and if we find a number greater than our current highest, we update $highestNumber
.
You can see the screenshot below for the output, I executed the code using VS code.
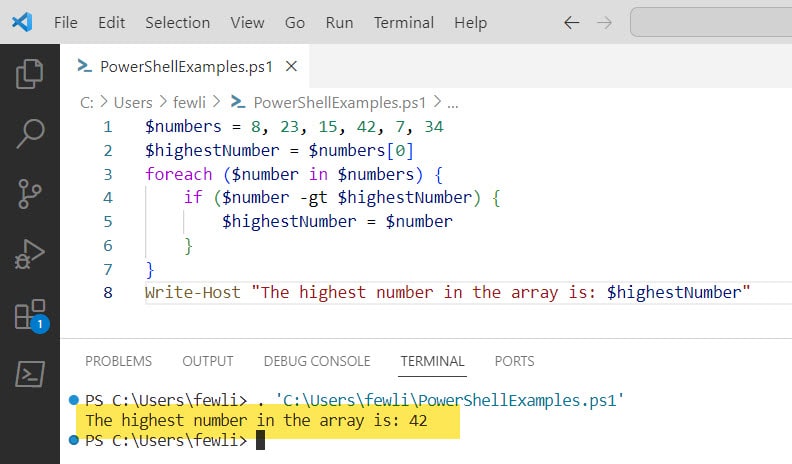
Method 4: Using LINQ in PowerShell
For those comfortable with .NET, PowerShell can interoperate with .NET’s Language Integrated Query (LINQ), which provides a more advanced method for querying collections.
Here’s an example using LINQ to get the highest number from an array in PowerShell.
Add-Type -AssemblyName System.Core
$highestNumber = [System.Linq.Enumerable]::Max($numbers)
Write-Host "The highest number in the array is: $highestNumber"
In this script, we add the System.Core
assembly to access LINQ methods and then call the Max
method on our array.
Conclusion
In this PowerShell tutorial, I have explained how to get the highest number in the array in PowerShell. Here are the 4 methods to check the highest number in a PowerShell array.
- Using the Measure-Object Cmdlet
- Sorting the Array
- Using a For Loop
- Using LINQ in PowerShell
You may also like:
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com