When working with PowerShell, it’s common to encounter situations where you must clean up an array by removing empty lines. This can happen when you’re processing text files, command output, or any data that may contain superfluous whitespace. In this PowerShell tutorial, I will explain you several methods to remove empty lines from an array in PowerShell.
To remove empty lines from an array in PowerShell, you can use the Where-Object
cmdlet with a condition that filters out lines that are empty or contain only whitespace. For example, $cleanArray = $arrayWithEmptyLines | Where-Object { $_.Trim() -ne "" }
will return an array without any empty lines.
PowerShell: remove blank lines from an array
An array in PowerShell is a data structure that holds a collection of items. These items can be of any type, including strings, integers, or even other arrays. In PowerShell, you can create an array by assigning multiple values to a variable:
$array = "line1", "line2", "line3"
Sometimes, PowerShell arrays might contain empty strings or lines, essentially strings with no characters or only whitespace characters.
Here are different methods to do it; let us understand with practical examples.
Method 1: Using the Where-Object
Cmdlet
One of the simplest ways to remove empty lines from an array in PowerShell is by using the Where-Object
cmdlet. This cmdlet allows you to filter out elements based on a particular condition. To remove empty lines from a PowerShell array, you can use it like this:
# Create an array with empty lines
$arrayWithEmptyLines = "line1", "", "line2", " ", "line3"
# Remove empty lines
$cleanArray = $arrayWithEmptyLines | Where-Object { $_.Trim() -ne "" }
# Display the clean array
$cleanArray
In this example, $_
represents each element in the array, and the Trim()
method removes any leading or trailing whitespace. The -ne
operator stands for “not equal.” So, the condition $_.Trim() -ne ""
checks if the trimmed element is not an empty string. If it’s not empty, it’s included in the $cleanArray
.
I ran the above PowerShell script using VS code, and you can see the output in the screenshot below:
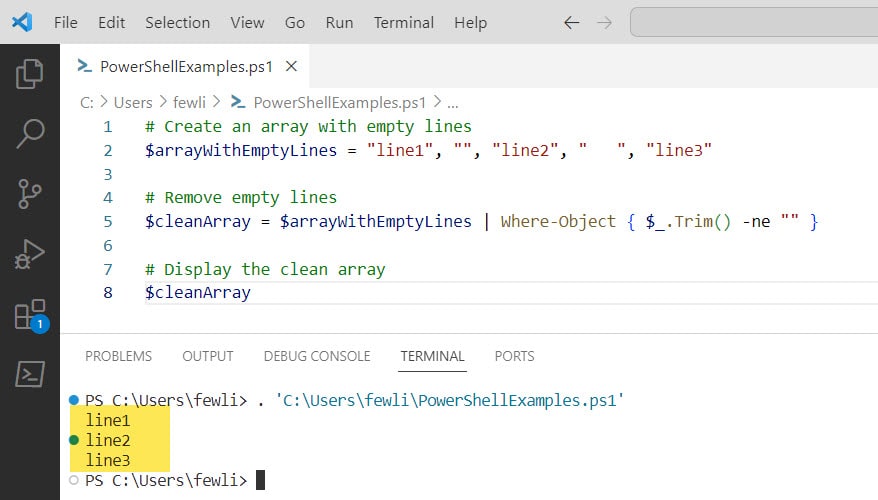
Method 2: Using Comparison Operators
Alternatively, you can use comparison operators to filter out empty strings directly in PowerShell. Here’s an example:
# Create an array with empty lines
$arrayWithEmptyLines = "line1", "", "line2", " ", "line3"
# Remove empty lines
$cleanArray = $arrayWithEmptyLines | Where-Object { $_ -ne "" -and $_ -ne " " }
# Display the clean array
$cleanArray
This method checks for empty strings and strings that consist only of spaces in PowerShell. However, if the array contains tabs or other whitespace characters, you might still need to use Trim()
.
Here is the output you can see in the screenshot below:
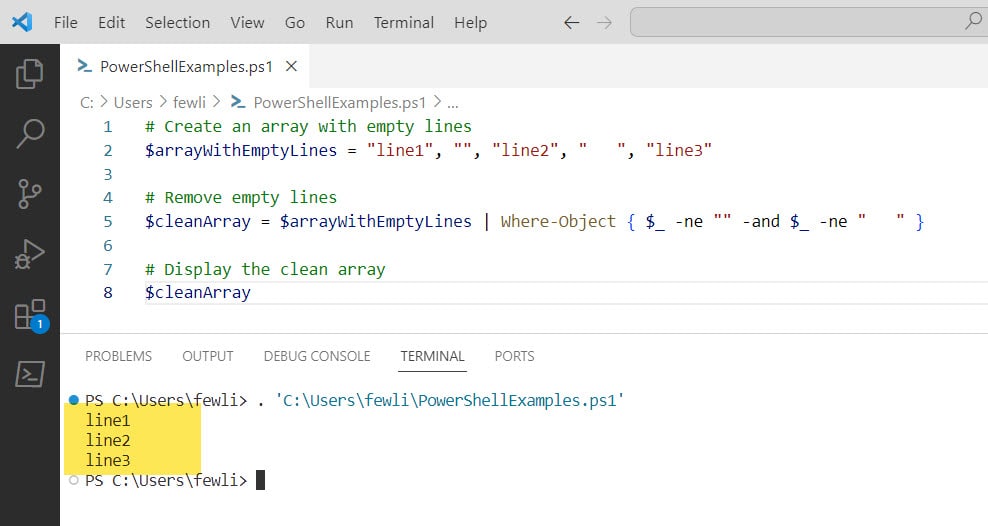
Method 3: Using a Foreach Loop
We can also use a foreach
loop to remove empty lines from a PowerShell array. Here is a complete script:
# Create an array with empty lines
$arrayWithEmptyLines = "line1", "", "line2", " ", "line3"
# Initialize an empty array to hold the clean elements
$cleanArray = @()
# Loop through each element in the original array
foreach ($line in $arrayWithEmptyLines) {
# Check if the line is not empty after trimming
if ($line.Trim() -ne "") {
# Add non-empty lines to the clean array
$cleanArray += $line
}
}
# Display the clean array
$cleanArray
In this loop, each element of the $arrayWithEmptyLines
is processed one by one. If the Trim()
method determines that a line is not empty, it’s added to the $cleanArray
.
Check out the screenshot below for the output; I ran the code using Visual Studio code.
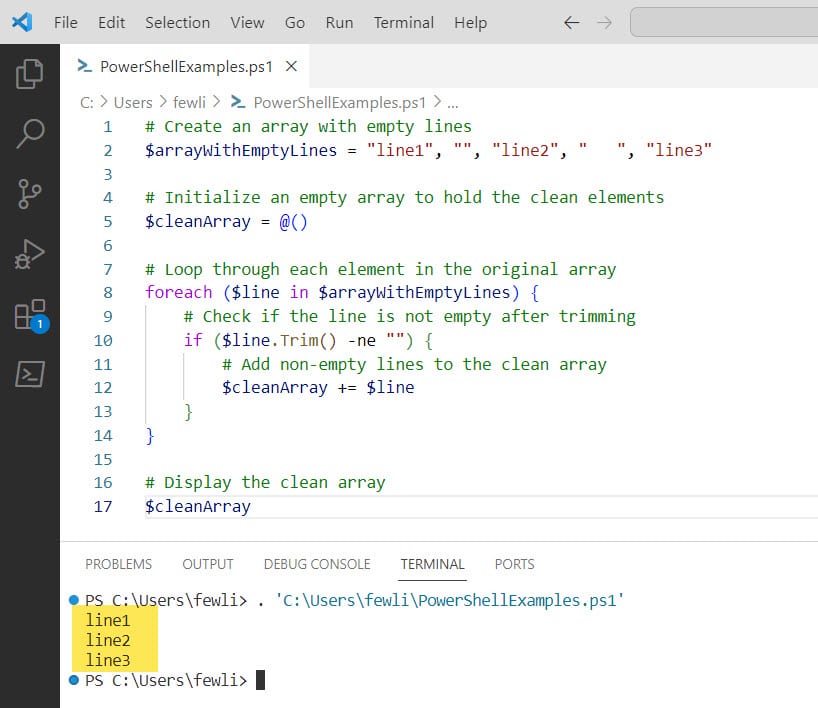
Method 4: Using Regular Expressions
PowerShell supports regular expressions (regex), which are patterns used to match character combinations in strings. You can use regex to remove empty lines from an array in PowerShell. You can check out the below example:
# Create an array with empty lines
$arrayWithEmptyLines = "line1", "", "line2", " ", "line3"
# Use regex to remove empty lines
$cleanArray = $arrayWithEmptyLines -notmatch '^\s*$'
# Display the clean array
$cleanArray
The regex pattern ^\s*$
matches any line that consists only of whitespace characters (or is completely empty). The -notmatch
operator is used to filter out any lines that match this pattern.
Conclusion
Removing empty lines from an array in PowerShell can be done in multiple ways. Whether you prefer the simplicity of the Where-Object
cmdlet, the precision of comparison operators, the control of a foreach
loop, or the power of regular expressions, PowerShell provides you with the flexibility to clean up your arrays effectively.
For beginners, it’s recommended to start with the Where-Object
cmdlet as it’s straightforward to understand. I hope you have an idea now of how to remove empty or blank lines from an array in PowerShell.
You may also like:
- PowerShell Function Array Parameters
- How to Replace a String in an Array Using PowerShell?
- How to Loop Through a PowerShell Array?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com