One of the powerful features of PowerShell is the ability to handle secure strings. Secure strings are essential to scripting when you need to handle sensitive data like passwords. This post will explore how to convert a string to a SecureString in PowerShell using the ConvertTo-SecureString cmdlet, ensuring your scripts handle sensitive information securely.
To convert a string to a SecureString in PowerShell, use the ConvertTo-SecureString
cmdlet. For a plain text string, pass the string and use the -AsPlainText
and -Force
parameters. For an encrypted string, pipe the encrypted data directly to ConvertTo-SecureString
. Here’s a quick example:
$plainTextPassword = "P@ssw0rd"
$securePassword = $plainTextPassword | ConvertTo-SecureString -AsPlainText -Force
SecureString in PowerShell
A SecureString in PowerShell is an instance of the System.Security.SecureString
class, which represents text that should be kept confidential. The text is encrypted in memory and can only be decrypted by the process that created it. This makes SecureString a more secure option for handling sensitive data compared to plain text strings.
ConvertTo-SecureString Cmdlet
The primary way to convert a string to a SecureString in PowerShell is by using the ConvertTo-SecureString
cmdlet. This cmdlet can convert plain text or encrypted standard strings into a SecureString object.
1. From Plain Text
To convert a plain text password to a SecureString, you can use the AsPlainText
and Force
parameters. Here is an example:
$plainTextPassword = "P@ssw0rd"
$securePassword = $plainTextPassword | ConvertTo-SecureString -AsPlainText -Force
This method is straightforward but not recommended for PowerShell scripts that will be shared or stored, as the plain text password can be easily exposed.
2. From Encrypted Standard String
A more secure method is to convert an already encrypted standard string into a SecureString in PowerShell. If you have a string that’s been encrypted with PowerShell’s ConvertFrom-SecureString
, you can convert it back to a SecureString like this:
$encryptedPassword = "01000000d08c9ddf0115d1118c7a00c04fc297eb01000000..."
$securePassword = $encryptedPassword | ConvertTo-SecureString
This method is safer because the encrypted password can be stored and shared without revealing the actual sensitive data.
Handling SecureString in PowerShell Scripts
When working with SecureStrings in scripts, you may need to pass them to cmdlets that require a PSCredential object. You can create a PSCredential object using a SecureString as follows:
$username = "User01"
$securePassword = ConvertTo-SecureString "P@ssw0rd" -AsPlainText -Force
$credential = New-Object System.Management.Automation.PSCredential ($username, $securePassword)
This PSCredential object can then be used with cmdlets that require authentication, such as Invoke-Command
or Get-WmiObject
.
Storing and Retrieving SecureStrings in PowerShell
You might want to store the encrypted password in a file or a script for later use. Here’s how you can export and import a SecureString in PowerShell:
Exporting SecureString to File
Below is the PowerShell script to export SecureString to a file.
$securePassword = ConvertTo-SecureString "P@ssw0rd" -AsPlainText -Force
$securePassword | ConvertFrom-SecureString | Out-File "C:\Bijay\securePassword.txt"
You can see the output in the screenshot below after I executed the PowerShell script using VS code:
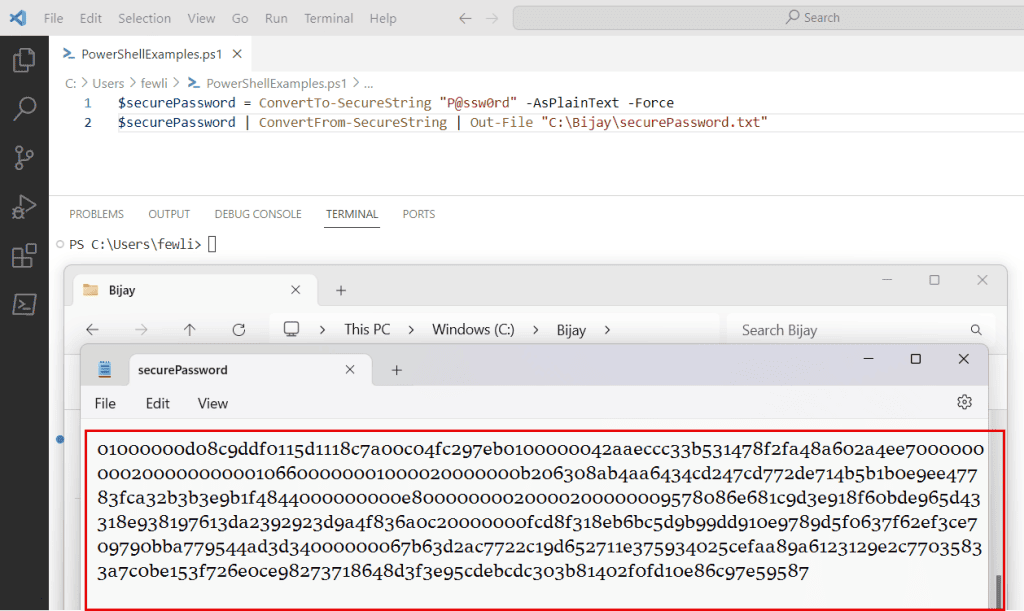
Importing SecureString from File
Below is the PowerShell script to import SecureString from a file.
$secureString = Get-Content "C:\securePassword.txt" | ConvertTo-SecureString
This process allows you to securely store the encrypted password and convert it back to a SecureString when needed.
Best Practices for Using SecureString in PowerShell
You can follow the below best practices while using SecureString in PowerShell:
- Avoid using plain text whenever possible. If you must use plain text, do so only in secure environments and never store the plain text password in scripts or files.
- Store encrypted passwords securely. If you need to save a password for later use, always store it in an encrypted form using
ConvertFrom-SecureString
. - Limit exposure of SecureStrings. Only decrypt SecureStrings when necessary and dispose of them as soon as you’re done, using the
Clear
method to remove the data from memory.
Reference – ConvertTo-SecureString
Conclusion
Converting strings to SecureString in PowerShell is crucial in writing secure scripts that handle sensitive information. Whether you’re using plain text or encrypted strings, PowerShell provides a straightforward way to work with secure data. With the ConvertTo-SecureString
cmdlet in PowerShell, you can easily convert a string to a SecureString in PowerShell.
You may also like:
- How to Convert String to Integer in PowerShell?
- How to Check if a Variable is Null or Empty in PowerShell?
- How to Replace a String in Text File with PowerShell?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com