While working on a PowerShell script, I got a requirement to check if a PowerShell variable in null or empty. In this PowerShell tutorial, we’ll explore how to check if a variable is null or empty in PowerShell using various methods.
To check if a variable in PowerShell is null or empty, you can use the -eq operator or the [string]::IsNullOrEmpty() method. For example, $variable -eq $null or [string]::IsNullOrEmpty($variable) will return True if the variable is null or an empty string, respectively. If you need to account for strings that contain only whitespace, use [string]::IsNullOrWhiteSpace($variable) instead.
Understanding $null in PowerShell
In PowerShell, $null
represents an absence of a value or an undefined value. It’s important to understand that $null
is not the same as an empty string or a space character; it’s a special variable that signifies ‘nothing’.
Here’s an example of setting a variable to $null
in PowerShell:
$value = $null
If you were to check the value of $value
, it would return nothing because it’s $null
.
Check if a Variable is Null or Empty in PowerShell
The simplest way to check if a variable in PowerShell is $null
is by using the equality operator -eq
. Here is a complete script.
$value = $null
if ($value -eq $null) {
"The variable is null."
} else {
"The variable is not null."
}
This will output “The variable is null.” if $value
is $null
.
I ran the PowerShell script using VS code and you can see the output in the screenshot below:
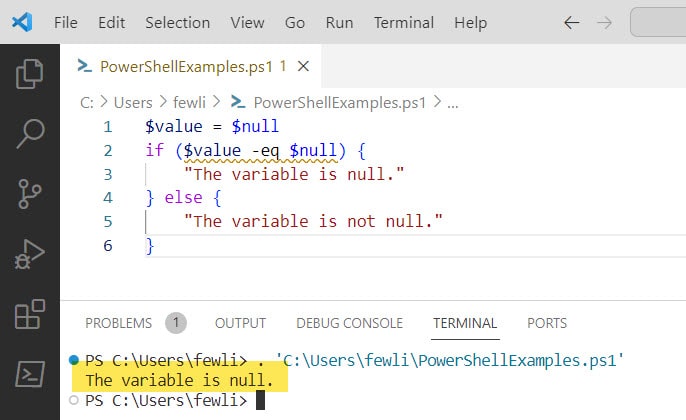
Checking for Empty Strings in PowerShell
In PowerShell, an empty string is different from $null
. It’s a string with a length of zero, represented as ""
or ''
. To check for an empty string in PowerShell, you can use the -eq
operator:
$stringValue = ""
if ($stringValue -eq "") {
"The string is empty."
} else {
"The string is not empty."
}
This will output “The string is empty.” if $stringValue
is an empty string.
Using IsNullOrEmpty Method
Another way to check for null or empty strings in PowerShell is by using the [string]::IsNullOrEmpty()
method from the .NET framework:
$stringValue = ""
if ([string]::IsNullOrEmpty($stringValue)) {
"The string is null or empty."
} else {
"The string is not null or empty."
}
This method returns true
if the variable is either $null
or an empty string in PowerShell.
You can see the output in the screenshot below:
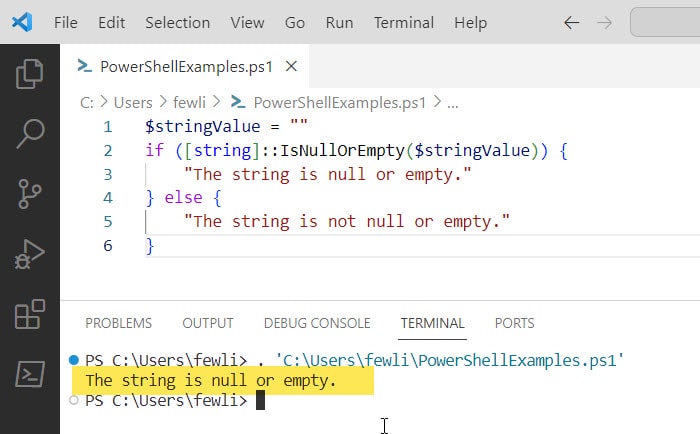
Using IsNullOrWhiteSpace Method
Sometimes a string may contain only whitespace characters, and you might want to treat it as empty. In this case, you can use the [string]::IsNullOrWhiteSpace()
method to check for null or empty in PowerShell:
$stringValue = " "
if ([string]::IsNullOrWhiteSpace($stringValue)) {
"The string is null, empty, or whitespace."
} else {
"The string has characters other than whitespace."
}
This method checks for $null
, empty, and whitespace-laden strings.
Example
Let’s say you have a script that processes user input. You want to ensure that the input is not $null
or empty before proceeding. Here’s how you might write that:
$userInput = Read-Host "Please enter your name"
if ([string]::IsNullOrWhiteSpace($userInput)) {
Write-Host "You did not enter a name!"
} else {
Write-Host "Hello, $userInput!"
}
This script prompts the user for their name and checks if the input is $null
, empty, or just whitespace. It only greets the user if they’ve entered a valid name.
Conclusion
By using the -eq
operator, the [string]::IsNullOrEmpty()
, or the [string]::IsNullOrWhiteSpace()
methods, you can easily check for $null
, empty, or whitespace-only strings in your PowerShell scripts.
In this PowerShell tutorial, I have explained different ways to check if a variable is null or empty in PowerShell.
You may also like:
- How to Set Environment Variables Using PowerShell?
- PowerShell Reference Variable
- Powershell Global Variable
- How to Convert String to Integer in PowerShell?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com