Recently, I got a requirement in PowerShell to convert a string to int. I tried different methods to do so. In this tutorial, I will explain how to convert string to integer in PowerShell using various methods. Using different examples, I will also show you how to convert string to number with decimal in PowerShell.
To convert a string to an integer in PowerShell, use the cast operator by prefixing the string variable with [int], like [int]$integerValue = “123”. Alternatively, you can use the ToInt32 method from the .NET Convert class: $integerValue = [convert]::ToInt32(“123”). For error handling, the [int]::TryParse() method is useful as it avoids exceptions if the conversion fails.
Convert String to Integer in PowerShell
A PowerShell string is a sequence of characters, while an integer is a whole number without a fractional component. Converting a string to an integer in PowerShell means interpreting the characters in the string as a numerical value.
Here, let us check different methods to convert a string to an integer in PowerShell using various methods.
Method 1: Type Casting
Type casting is the simplest method to convert a string to an integer. It involves prefixing the variable with the desired data type in square brackets. Here’s an example:
[string]$stringNumber = "42"
[int]$integerNumber = [int]$stringNumber
Write-Host $integerNumber
This script will output 42
as an integer. The [int]
cast tells PowerShell to interpret $stringNumber
as an integer.
Once you execute the PowerShell script, you can see the output in the screenshot below.
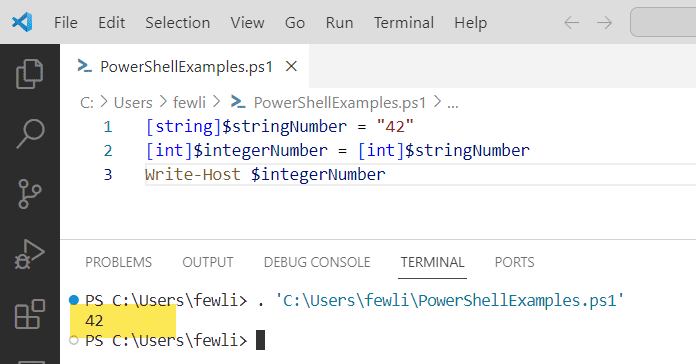
Method 2: Using the -as Operator
Another way to perform the conversion is by using the -as
operator, which attempts to convert the value on its left to the type specified on its right:
$stringNumber = "42"
$integerNumber = $stringNumber -as [int]
Write-Host $integerNumber
This will also result in 42
being output as an integer. The -as
operator is safe to use because if the conversion fails, it will return $null
instead of throwing an exception.
Method 3: Parse and TryParse Methods
The [int]::Parse()
method is a more explicit way to convert a string to an integer. It’s a static method of the Int32
structure that attempts to parse a string as an integer:
$stringNumber = "42"
$integerNumber = [int]::Parse($stringNumber)
Write-Host $integerNumber
If $stringNumber
is not a valid integer, this method will throw an exception. To avoid exceptions and handle conversion errors more gracefully, you can use the TryParse
method:
$stringNumber = "42"
$integerNumber = 0
[bool]$result = [int]::TryParse($stringNumber, [ref]$integerNumber)
if ($result) {
Write-Host $integerNumber
} else {
Write-Host "Conversion failed"
}
The TryParse
method returns a boolean indicating whether the conversion succeeded and outputs the converted integer through its second parameter.
You can see the output in the screenshot below:
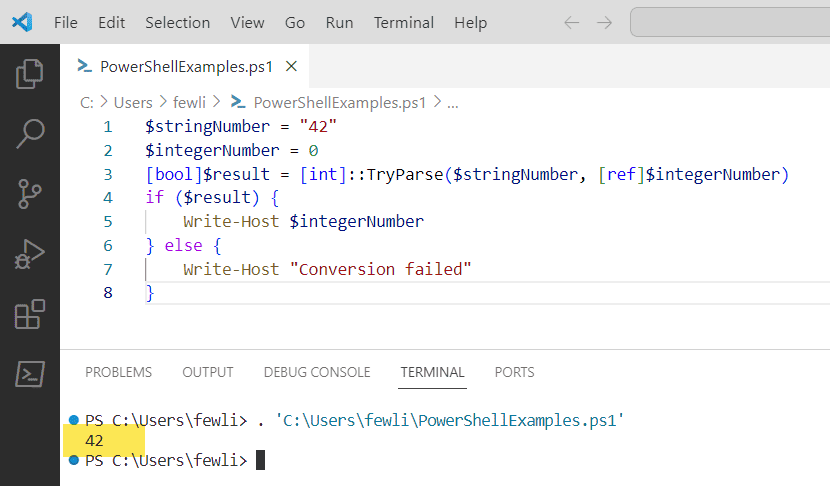
Method 4: Convert Class
The Convert
class in .NET provides a ToInt32
method that can convert various types to a 32-bit integer. Here’s how you can use it in PowerShell:
$stringNumber = "42"
$integerNumber = [convert]::ToInt32($stringNumber)
Write-Host $integerNumber
This method is similar to Parse
, but it can handle null
and other types more gracefully.
Handling Non-Numeric Strings
It’s essential to consider what happens when a string cannot be converted to an integer. For instance, if you try to convert a string with alphabetical characters, all the methods discussed will fail or return $null
. It’s good practice to validate the string before attempting conversion or to use TryParse
to handle potential errors without throwing exceptions.
Advanced Conversion Scenarios
Sometimes, strings represent numbers in different bases, such as hexadecimal. PowerShell can handle these cases as well:
$hexString = "2A"
$integerNumber = [convert]::ToInt32($hexString, 16)
Write-Host $integerNumber
This script will output 42
, which is the decimal equivalent of the hexadecimal number 2A
.
PowerShell: convert string to int error handling
Error handling is an important aspect when converting strings to integers in PowerShell, especially when the string may not represent a valid integer. PowerShell provides several methods to handle errors that may occur during the conversion process.
Using TryParse for Error Handling
The [int]::TryParse()
method in PowerShell is specifically designed for error handling. It attempts to parse a string as an integer and returns a Boolean value indicating success or failure. It also outputs the result of the conversion to a variable passed by reference.
Here’s an example:
$stringNumber = "notanumber"
$integerNumber = 0
$result = [int]::TryParse($stringNumber, [ref]$integerNumber)
if ($result) {
"Conversion successful: $integerNumber"
} else {
"Conversion failed."
}
If $stringNumber
is not a valid integer, $result
will be $false
, and $integerNumber
will remain 0
.
You can see in the screenshot below that I executed the script using VS code.
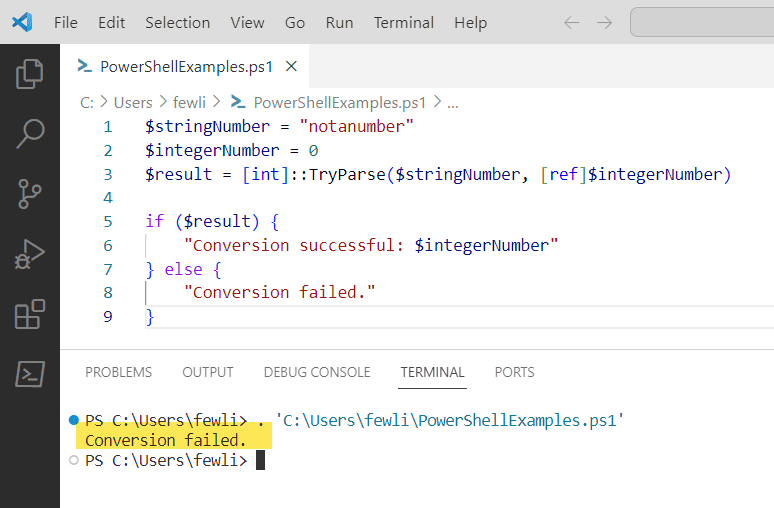
Using Try/Catch Blocks
Another method for error handling is using try/catch blocks in PowerShell. When using methods like [int]::Parse()
or [convert]::ToInt32()
, which can throw exceptions on failure, and wrap these calls in a try/catch block to handle the error gracefully.
Example:
$stringNumber = "notanumber"
try {
$integerNumber = [int]::Parse($stringNumber)
"Conversion successful: $integerNumber"
} catch [System.FormatException] {
"Conversion failed: Input string was not in a correct format."
} catch {
"Conversion failed with an unexpected error: $_"
}
In this example, if the string is not a valid integer, a System.FormatException
will be caught and handled.
Using Conditional Logic with -as Operator
The -as
operator in PowerShell can be used for error handling because it returns $null
if the conversion is not possible, which can be checked using conditional logic.
Example:
$stringNumber = "notanumber"
$integerNumber = $stringNumber -as [int]
if ($null -eq $integerNumber) {
"Conversion failed."
} else {
"Conversion successful: $integerNumber"
}
This method is less explicit than TryParse
but can be useful for simple conversions where the presence of a non-integer value is not critical.
When converting strings to integers in PowerShell, it’s important to anticipate and handle potential errors. The [int]::TryParse()
method is generally the safest and most robust approach, as it avoids throwing exceptions altogether.
Try/catch blocks are useful when you need to catch and handle specific exceptions from methods that do not have a TryParse
equivalent. The -as
operator is a simpler but less informative method that can be used when you don’t need detailed error information.
Convert string to number with decimal in PowerShell
Converting a string to a number with decimals in PowerShell involves parsing the string as a floating-point number instead of an integer. In PowerShell, you can use [double]
or [decimal]
for higher precision if needed.
1. Using Type Casting
To convert a string to a double in PowerShell, you can cast the string directly:
[string]$stringNumber = "123.45"
[double]$doubleNumber = [double]$stringNumber
Write-Host $doubleNumber
For a decimal, the process is similar:
[string]$stringNumber = "123.45"
[decimal]$decimalNumber = [decimal]$stringNumber
Write-Host $decimalNumber
2. Using the Convert Class
The Convert
class provides methods to convert strings to numbers with decimals in PowerShell:
[string]$stringNumber = "123.45"
[double]$doubleNumber = [convert]::ToDouble($stringNumber)
Write-Host $doubleNumber
For converting to a decimal:
[string]$stringNumber = "123.45"
[decimal]$decimalNumber = [convert]::ToDecimal($stringNumber)
Write-Host $decimalNumber
3. Error Handling with TryParse
For error handling, you can use the TryParse
method of the double
or decimal
type to convert the string and catch any conversion errors safely in PowerShell:
[string]$stringNumber = "notanumber"
[double]$doubleNumber = 0
$result = [double]::TryParse($stringNumber, [ref]$doubleNumber)
if ($result) {
"Conversion successful: $doubleNumber"
} else {
"Conversion failed."
}
For decimals:
[string]$stringNumber = "notanumber"
[decimal]$decimalNumber = 0
$result = [decimal]::TryParse($stringNumber, [ref]$decimalNumber)
if ($result) {
"Conversion successful: $decimalNumber"
} else {
"Conversion failed."
}
4. Using -as Operator
The -as
operator can also be used for converting strings to numbers with decimals in PowerShell, with the added benefit of not throwing an error on failure:
[string]$stringNumber = "123.45"
[double]$doubleNumber = $stringNumber -as [double]
if ($doubleNumber -eq $null) {
"Conversion failed."
} else {
"Conversion successful: $doubleNumber"
}
You can see the output in the screenshot below:
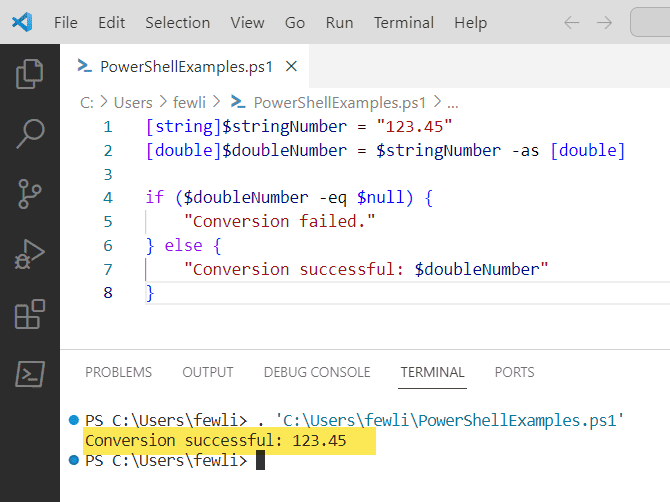
When converting strings to numbers with decimals in PowerShell, you have several options, including type casting, using the Convert
class, or employing the TryParse
method for error handling. The -as
operator provides a simpler but less explicit alternative. Whether you choose [double]
or [decimal]
depends on the level of precision required for your specific use case.
Conclusion
In this PowerShell tutorial, I have explained how to convert a string to an integer in PowerShell using various methods.
- Type Casting
- Using the -as Operator
- Parse and TryParse Methods
- Convert Class
Also, I have explained:
- PowerShell convert string to int error handling
- Convert string to number with decimal in PowerShell
You may also like:
- How to Convert Multiline String to Array in PowerShell?
- How to Convert String to DateTime in PowerShell?
- How To Convert Array To Comma Separated String In PowerShell?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com