Most of the time, you might get a requirement to convert an array to a comma separated string in PowerShell. In this PowerShell tutorial, I will explain how to convert an array to a comma-separated string in PowerShell using various methods.
To convert an array to a comma-separated string in PowerShell, use the -join
operator. For example, $myArray = @('apple', 'banana', 'cherry')
can be converted to a string by $commaSeparated = $myArray -join ','
. This will output apple,banana,cherry
. It’s a quick and efficient way to concatenate array elements into a single, comma-delimited string.
Convert Array To Comma Separated String In PowerShell
Here are different methods to convert a PowerShell array to a comma-separated string. Let us understand each method with examples.
Method 1: Using the -join Operator
The -join
operator is the simplest way to convert an array to a comma-separated string in PowerShell. Here’s an example:
# Define an array
$myArray = @('apple', 'banana', 'cherry')
# Convert array to comma-separated string
$commaSeparated = $myArray -join ','
# Output the result
Write-Host $commaSeparated
As you can see in the screenshot below, this will output after I execute the PowerShell script.
apple,banana,cherry
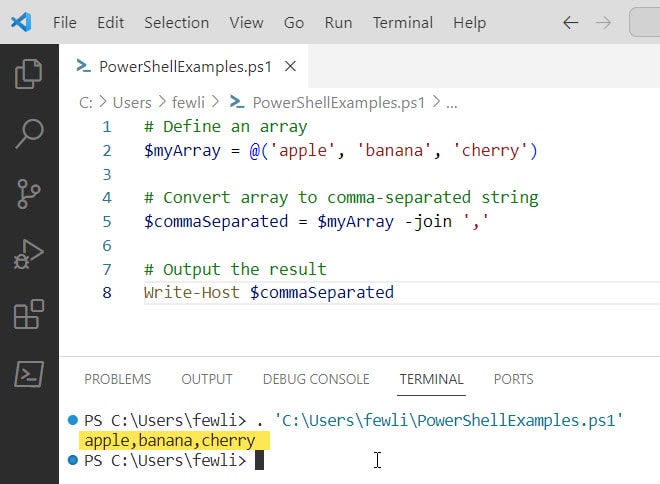
Method 2: Using a ForEach Loop
If you want more control over the process, you can use a foreach
loop to construct the string manually in PowerShell:
# Define an array
$myArray = @('apple', 'banana', 'cherry')
# Initialize an empty string
$commaSeparated = ""
# Loop through each item in the array
foreach ($item in $myArray) {
# Add item to the string with a comma
$commaSeparated += $item + ','
}
# Remove the trailing comma
$commaSeparated = $commaSeparated.TrimEnd(',')
# Output the result
Write-Host $commaSeparated
This will give you the same output as the first method. Check the screenshot below:
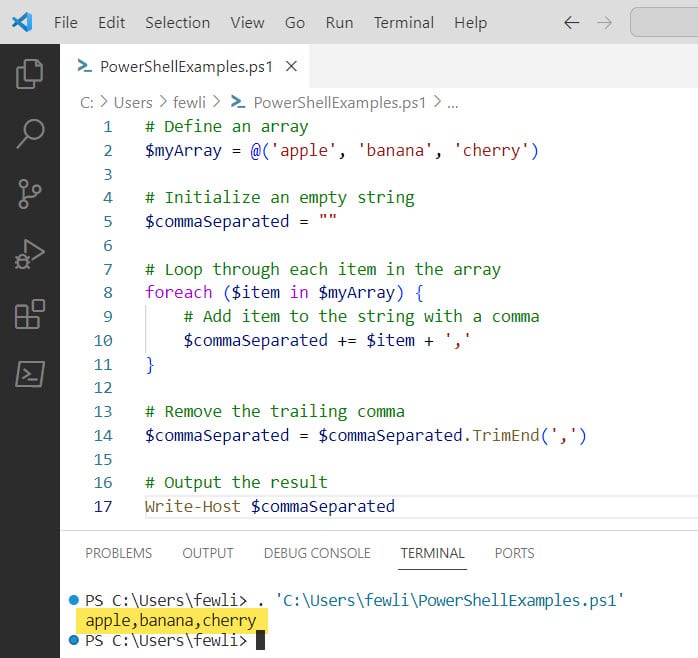
Method 3: Using the Out-String Cmdlet
You can also use the Out-String
cmdlet in PowerShell to convert the array to comma-separated string in PowerShell, although it’s not as straightforward for this particular task:
# Define an array
$myArray = @('apple', 'banana', 'cherry')
# Convert array to comma-separated string and remove newlines and excess spaces
$commaSeparated = $myArray | Out-String -Stream | ForEach-Object { $_.Trim() } -join ','
# Output the result
Write-Host $commaSeparated
This will also output:
apple,banana,cherry
Method 4: Using the ConvertTo-Csv Cmdlet
If your array consists of objects with properties, you can use the ConvertTo-Csv
cmdlet to convert it to a CSV format, which is inherently comma-separated:
# Define an array of objects
$myArray = @(
[PSCustomObject]@{Name='apple'; Color='green'},
[PSCustomObject]@{Name='banana'; Color='yellow'},
[PSCustomObject]@{Name='cherry'; Color='red'}
)
# Convert array to CSV format
$csvFormat = $myArray | ConvertTo-Csv -NoTypeInformation
# Convert CSV to array of strings (excluding the header)
$csvLines = $csvFormat -split "`n" | Select-Object -Skip 1
# Join the CSV lines into a single comma-separated string
$commaSeparated = $csvLines -join ','
# Output the result
Write-Host $commaSeparated
This will output each object’s properties in a comma-separated fashion:
"apple","green","banana","yellow","cherry","red"
Please note that the ConvertTo-Csv
cmdlet includes quotation marks around each element by default.
Conclusion
In this PowerShell tutorial, I have explained different methods to convert an array to a comma-separated string in PowerShell. The -join
operator provides the most straightforward and concise approach, while a foreach
loop offers more control and customization. For objects with properties, the ConvertTo-Csv
cmdlet can be particularly useful, although it may require additional formatting.
You may also like:
- Split Comma Separated String To Array In PowerShell
- How to Check if an Array Contains a String in PowerShell?
- How to Get The Last Item In an Array in PowerShell?
- How to Remove Empty Lines from an Array in PowerShell?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com