In PowerShell, arrays are collections of items where each item has an index. To get the last item in a PowerShell array, you can use different methods. Below is a complete tutorial on how to retrieve the last item from an array in PowerShell.
To get the last item in a PowerShell array, you can use a negative index: $lastItem = $array[-1]
. This retrieves the last element directly. Alternatively, use the Select-Object
cmdlet with -Last 1
to achieve the same result: $lastItem = $array | Select-Object -Last 1
. Both methods will return the last item of the array.
Get The Last Item In an Array in PowerShell
Now, let us check the below methods to get the last element in a PowerShell array.
1. Using Index
PowerShell allows you to use a negative index to access items from the end of the array. -1
will always give you the last item:
# Define an array with city names
$cities = 'New York', 'Los Angeles', 'Chicago'
# Access the last city using a negative index
$lastCity = $cities[-1]
# Display the last city
Write-Output $lastCity
This script will output Chicago
, which is the last item in the $cities
array. You can check the output in the screenshot below after I executed the PowerShell script using VS code.
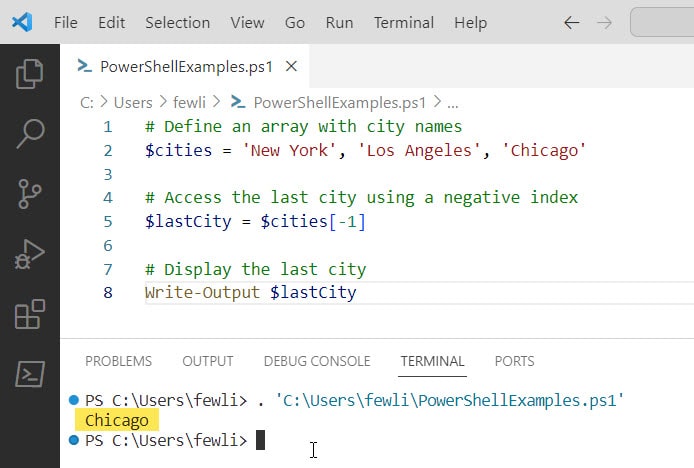
2. Using the Length
Property
You can also use the Length
property of a PowerShell array to calculate the index of the last item:
# Define an array with city names
$cities = 'New York', 'Los Angeles', 'Chicago'
# Calculate the index of the last city
$lastIndex = $cities.Length - 1
# Access the last city using the calculated index
$lastCity = $cities[$lastIndex]
# Display the last city
Write-Output $lastCity
This script will also output Chicago
. It uses the Length
property to find the index of the last item in the $cities
array. Check out the output in the screenshot below:
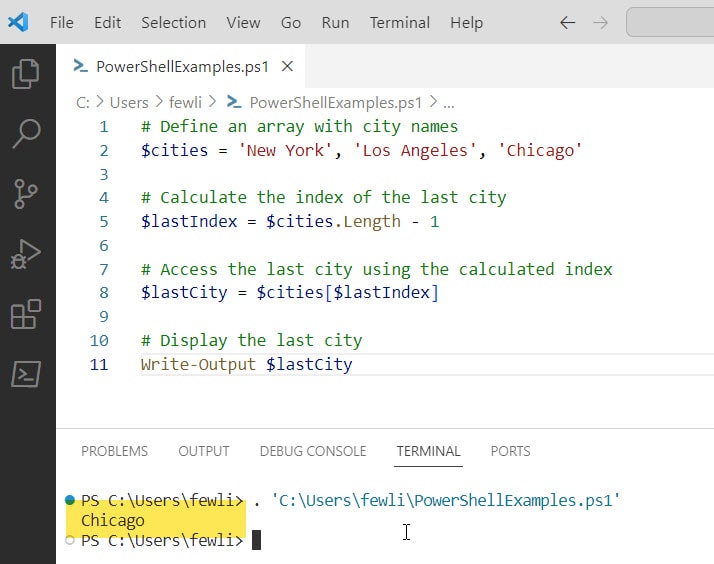
3. Using the Select-Object
Cmdlet
The Select-Object
cmdlet can be used with the -Last
parameter to select the last item of the PowerShell array:
# Define an array
$array = 'Red', 'Green', 'Blue'
# Get the last item using Select-Object
$lastItem = $array | Select-Object -Last 1
# Display the last item
Write-Output $lastItem
This will output Blue
by selecting the last item from the PowerShell array. Here is the screenshot below for your reference.
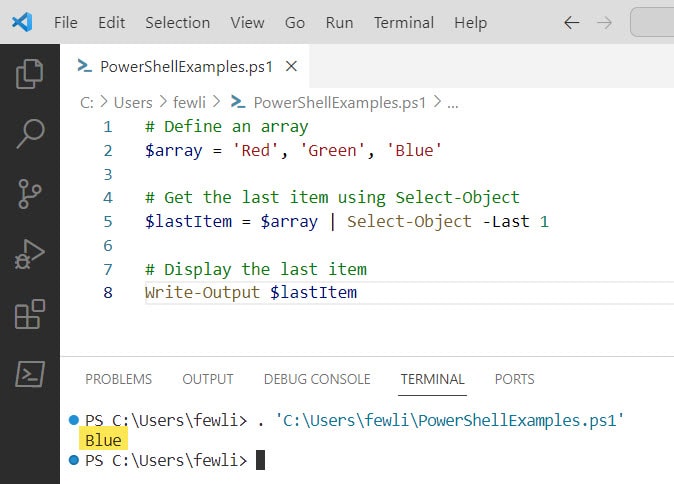
Conclusion
In PowerShell, you can get the last item of an array by using a negative index, by calculating the index with the Length
property, or by using the Select-Object
cmdlet with the -Last
parameter.
In this PowerShell tutorial, I have explained how to get the last element of an array in PowerShell by using various methods.
You may also like:
- How to Replace a String in an Array Using PowerShell?
- How To Check If Array Is Empty In PowerShell?
- How To Access First Item In an Array In PowerShell?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com