Do you want to get the first item in the array in PowerShell? In this PowerShell tutorial, I will explain different ways to access the first item in an array in PowerShell.
To access the first item in an array in PowerShell, use the index 0
for the array. For example, if you have an array $cities
, you can retrieve the first item with $cities[0]
. This is because arrays in PowerShell are zero-indexed, meaning the counting starts from 0
.
Get the first element in an array in PowerShell
In PowerShell, arrays are used to store a collection of items. When you want to access the first item in an array, you can do so by using its index. PowerShell Arrays are zero-indexed, meaning the first item is at index 0.
Here are a few methods that explain how to get the first item in a PowerShell array, along with examples:
1. Get the first item in an array using the index in PowerShell
The most straightforward method to get the first item in an array is by using its index directly in PowerShell. Here is the complete PowerShell script.
# Define an array with city names
$cities = 'New York', 'Los Angeles', 'Chicago'
# Access the first city using its index
$firstCity = $cities[0]
# Display the first city
Write-Output $firstCity
When this script is run, it will output “New York"
, which is the first item in the $cities
array. You can see the screenshot below for the output after I executed the code using VS code.
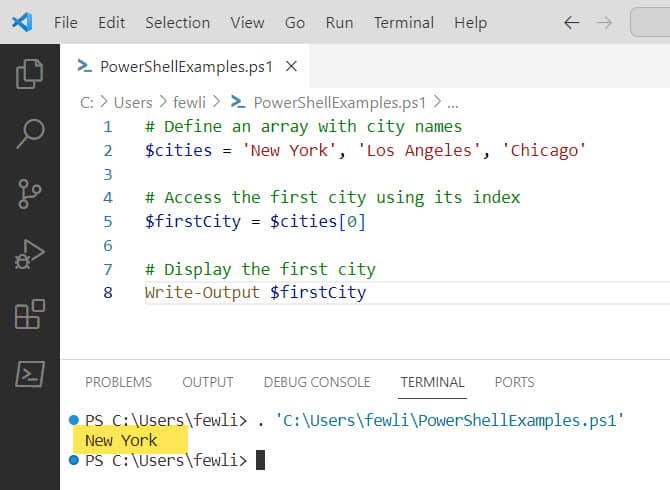
2. Using the Select-Object Cmdlet
Another method to get first element in array in PowerShell is by using the Select-Object
cmdlet with the -First
parameter, which is useful when working with pipelined commands:
# Define an array with city names
$cities = 'New York', 'Los Angeles', 'Chicago'
# Get the first city using Select-Object
$firstCity = $cities | Select-Object -First 1
# Display the first city
Write-Output $firstCity
This script will also output “New York"
by selecting the first item from the $cities
array. Check out the screenshot below, I have executed the above script in my system.
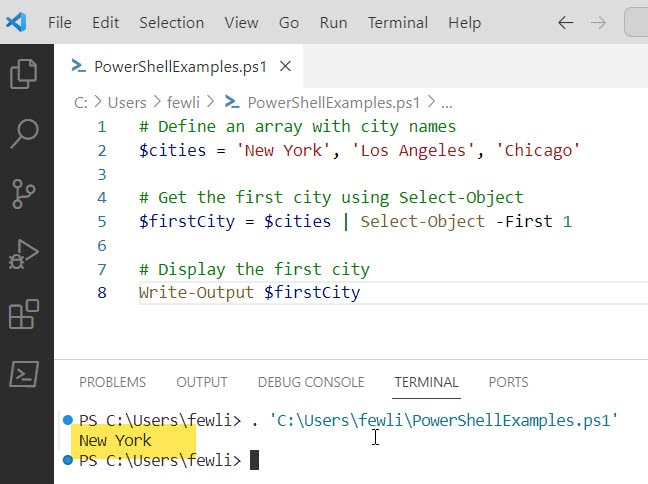
3. Using Array Slicing
You can also use array slicing to get a subset of the array, which includes just the first item of the PowerShell array:
# Define an array with city names
$cities = 'New York', 'Los Angeles', 'Chicago'
# Get the first city using array slicing
$firstCity = $cities[0..0]
# Since slicing returns an array, we need to access the first element
$firstCity = $firstCity[0]
# Display the first city
Write-Output $firstCity
This method uses array slicing to create a sub-array containing only the first city and then accesses the first element of that sub-array, resulting in New York
.
You can see the screenshot below after I executed the complete PowerShell script.
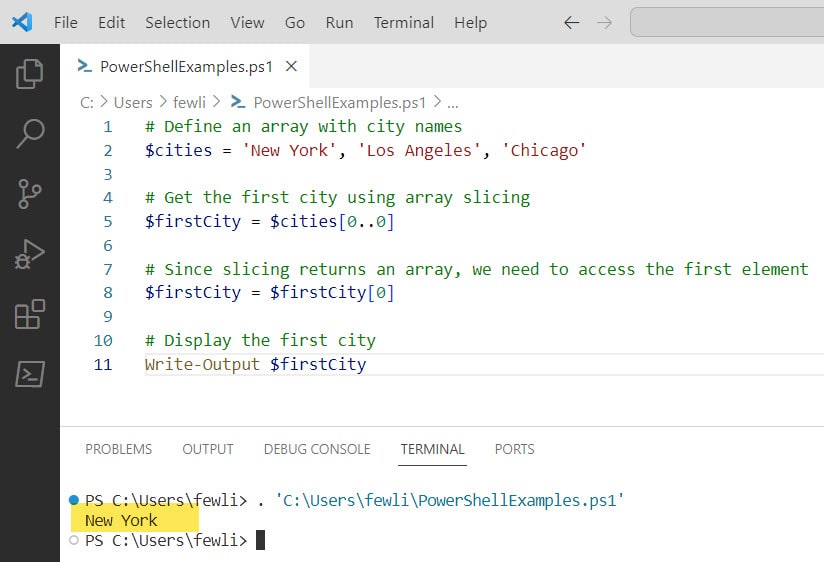
4. Using the ForEach-Object Cmdlet
If you’re dealing with a situation where you want to iterate over an array but only handle the first item, you could use ForEach-Object
with a break statement:
# Define an array with city names
$cities = 'New York', 'Los Angeles', 'Chicago'
# Iterate over the array and break after the first city
$cities | ForEach-Object {
$firstCity = $_
break
}
# Display the first city
Write-Output $firstCity
This script will iterate over the $cities
array and output the first city, New York
, then it will exit the loop immediately after processing the first item.
Conclusion
In PowerShell, accessing the first item in an array is most commonly done by referencing the item at index 0. However, depending on the context, other methods like Select-Object
, array slicing, or ForEach-Object
may be more appropriate. In this PowerShell tutorial, I have explained different ways or methods to get the first element in the array in PowerShell.
You may also like:
- How to Loop Through a PowerShell Array?
- How To Check If Array Is Empty In PowerShell?
- Get The Last Item In an Array in PowerShell
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com