There will be times when you want to replace carriage returns in strings using PowerShell. In this tutorial, we will learn how to replace carriage returns in strings using PowerShell.
To replace a string containing a carriage return in PowerShell, use the -replace operator with the carriage return escape sequence `r. For example, $string -replace “r”, “replacement text”will replace all carriage returns in$stringwith “replacement text”. If you want to remove carriage returns entirely, use an empty string as the replacement:$string -replace “r”, “”.
Replace Carriage Returns in Strings Using PowerShell
A carriage return (CR) is a special character used to reset a device’s position to the beginning of a line of text. It is represented by the escape sequence \r
. In Windows, a combination of carriage return and line feed (LF), represented by \r\n
, is used to mark the end of a line.
To find carriage returns in a string, we can use the -match
operator in PowerShell. Here’s an example of how to detect if a string contains a carriage return:
$string = "This is a line of text.`rThis is another line of text."
if ($string -match "`r") {
Write-Host "Carriage return found!"
} else {
Write-Host "No carriage return found."
}
To replace carriage returns in strings, we use the -replace
operator in PowerShell. The syntax for the -replace
operator is 'pattern', 'replacement'
, where pattern
is the text you want to replace, and replacement
is the text you want to replace it with.
Here’s an example of how to replace carriage returns with a space in PowerShell:
$string = "This is a line of text.`rThis is another line of text."
$modifiedString = $string -replace "`r", " "
Write-Host $modifiedString
This will output:
This is a line of text. This is another line of text.
YOu can see the output in the screenshot below:
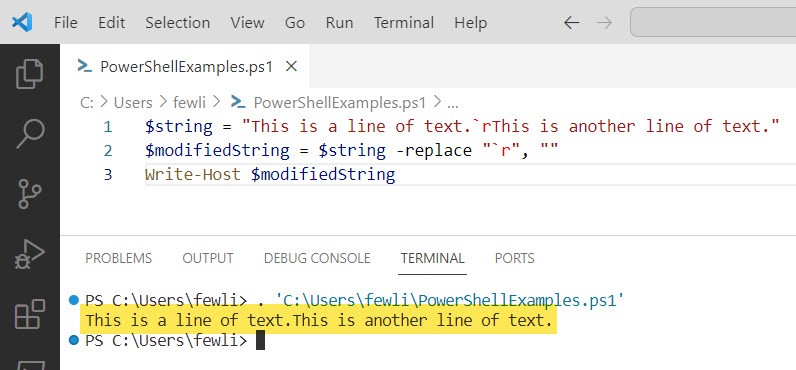
If you want to replace carriage returns with a newline character to maintain the line separation, you can do this:
$string = "This is a line of text.`rThis is another line of text."
$modifiedString = $string -replace "`r", "`n"
Write-Host $modifiedString
Removing Carriage Returns
Sometimes, you may want to remove carriage returns altogether. Here’s how you can do it:
$string = "This is a line of text.`rThis is another line of text."
$modifiedString = $string -replace "`r", ""
Write-Host $modifiedString
This will output:
This is a line of text.This is another line of text.
Working with Files
When dealing with text files, you might want to replace carriage returns within the file content. Here’s how you can read a file, replace carriage returns, and then write the content back to the file:
# Read the content of the file
$content = Get-Content -Path "C:\path\to\your\file.txt" -Raw
# Replace carriage returns with the desired string
$modifiedContent = $content -replace "`r", "`n"
# Write the modified content back to the file
Set-Content -Path "C:\path\to\your\file.txt" -Value $modifiedContent
Conclusion
In this PowerShell tutorial, I will explain, how to replace carriage returns in strings using PowerShell.
You may also like:
- Replace Strings Containing Backslashes in PowerShell
- PowerShell Replace String Case Insensitive
- Replace String Containing Double Quotes in PowerShell
- How to Replace Semicolon with Comma in PowerShell?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com