One common task is replacing semicolons with commas, particularly when dealing with CSV (Comma-Separated Values) files that incorrectly use semicolons as delimiters. PowerShell provides a straightforward and efficient way to replace semicolon with comma in PowerShell.
To replace semicolons with commas in PowerShell, use the .Replace()
method on a string, like $string.Replace(';', ',')
. For example, if you have a string $data = 'apple;banana;cherry'
, you can run $data.Replace(';', ',')
to get 'apple,banana,cherry'
. This method is simple and effective for quick replacements in strings.
PowerShell Replace Semicolon with Comma
In PowerShell, strings are objects that have methods you can use to manipulate them. One of these methods is the .Replace()
method, which allows you to replace occurrences of a specified substring with another substring.
Here is the basic syntax for the .Replace()
method:
$string.Replace('oldChar', 'newChar')
In this syntax, $string
is your original string, oldChar
is the character you want to replace, and newChar
is the character you want it replaced with.
Example-1: Replacing Semicolons with Commas in a String
Let’s start with a simple example. Suppose you have a single string that contains semicolons, and you want to replace them with commas:
# Original string with semicolons
$originalString = 'One;Two;Three;Four'
# Replace semicolons with commas
$modifiedString = $originalString.Replace(';', ',')
# Output the modified string
Write-Output $modifiedString
When you run this script, the output will be:
One,Two,Three,Four
Once you run the PowerShell script using VS code, you can see the output in the screenshot below:
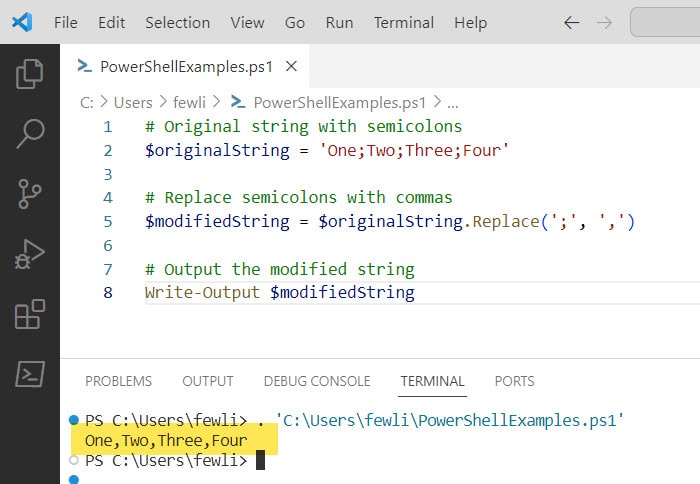
Example-2: Working with Files
Replacing characters becomes slightly more complex when dealing with files in PowerShell. Here’s how you can replace semicolons with commas in the contents of a file in PowerShell:
- Read the contents of the file into a variable.
- Use the
.Replace()
method to replace all semicolons with commas. - Write the modified content back to the file or a new file.
Here’s a complete example that reads a file, replaces semicolons with commas, and writes the content back to a new file:
# Path to the original file
$originalFilePath = 'C:\file.txt'
# Path to the modified file
$modifiedFilePath = 'C:\modified_file.txt'
# Read the content of the original file
$originalContent = Get-Content -Path $originalFilePath
# Replace semicolons with commas in the content
$modifiedContent = $originalContent -replace ';', ','
# Write the modified content to a new file
$modifiedContent | Set-Content -Path $modifiedFilePath
After running this script, you’ll have a new file with all semicolons replaced by commas.
Conclusion
PowerShell’s replace methods are powerful tools for string manipulation. By understanding how to use the .Replace()
method and the -replace
operator, you can quickly and efficiently transform text and data within your scripts. Whether you’re a beginner or an experienced PowerShell user, these methods are essential for handling and preparing data for processing or analysis.
In this PowerShell tutorial, I have explained how to replace semicolons with commas in PowerShell with examples.
You may also like:
- PowerShell get-childitem sort by date
- PowerShell If Date Is Older Than 30 Days
- How to Get-Date Without Time in PowerShell?
- PowerShell Replace String Case Insensitive
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com