Do you want to know about the PowerShell function return values? In this PowerShell tutorial, I will explain how PowerShell functions handle return values, with examples.
PowerShell Function Return Value
In PowerShell, a function can return a value using the return
keyword. However, it’s important to note that PowerShell functions also return values implicitly. This means that any output which is not captured or suppressed will be returned by the function.
Here’s a simple example:
function Get-MultipliedValue {
param($value1, $value2)
return $value1 * $value2
}
$result = Get-MultipliedValue -value1 5 -value2 10
Write-Host "The result is $result"
In this example, the function Get-MultipliedValue
takes two parameters and returns their product.
PowerShell Function Return String
Returning a string from a PowerShell function is straightforward. If you output a string without assigning it to a variable, it becomes the return value.
Example:
function Get-Greeting {
param($name)
return "Hello, $name!"
}
$greeting = Get-Greeting -name "John"
Write-Host $greeting
This function, Get-Greeting
, returns a string that greets the user by name.
Here is you can see the output in the screenshot below:
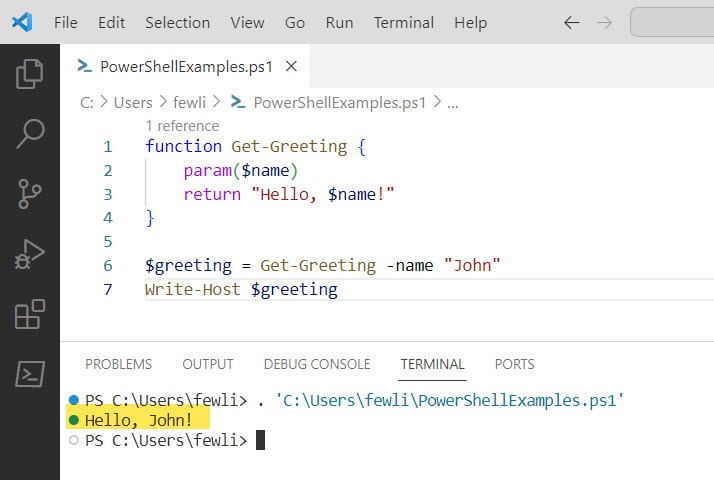
PowerShell Function Return Boolean
A boolean return value is often used to indicate success or failure. Here’s how you can return a boolean from a function:
function Test-IsEven {
param($number)
return $number % 2 -eq 0
}
$isEven = Test-IsEven -number 4
Write-Host "Is the number even? $isEven"
The function Test-IsEven
checks whether a number is even and returns $true
or $false
.
Once you run the PowerShell script, you can see the output in the screenshot below.
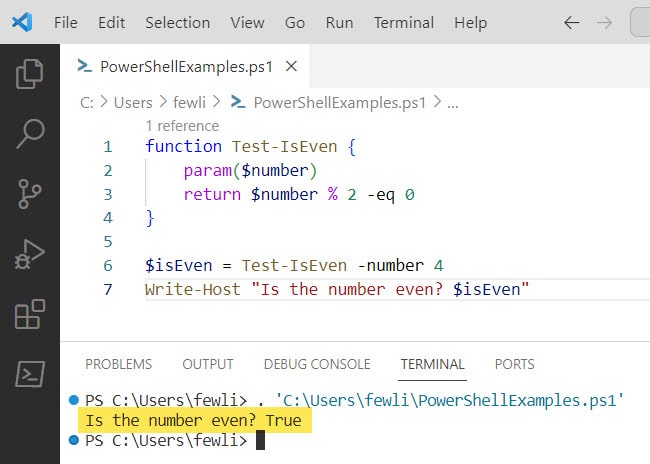
PowerShell Function Return Multiple Values
You can use an array, a hashtable, or a custom object to return multiple values from a function. Here’s an example using a hashtable in PowerShell:
function Get-MathResults {
param($number)
$squared = $number * $number
$sqrt = [math]::Sqrt($number)
return @{Squared = $squared; SquareRoot = $sqrt}
}
$results = Get-MathResults -number 9
$results.Squared
$results.SquareRoot
This function returns a hashtable with the squared value and the square root of the input number.
You can see the output in the screenshot below after running the code using VS code.
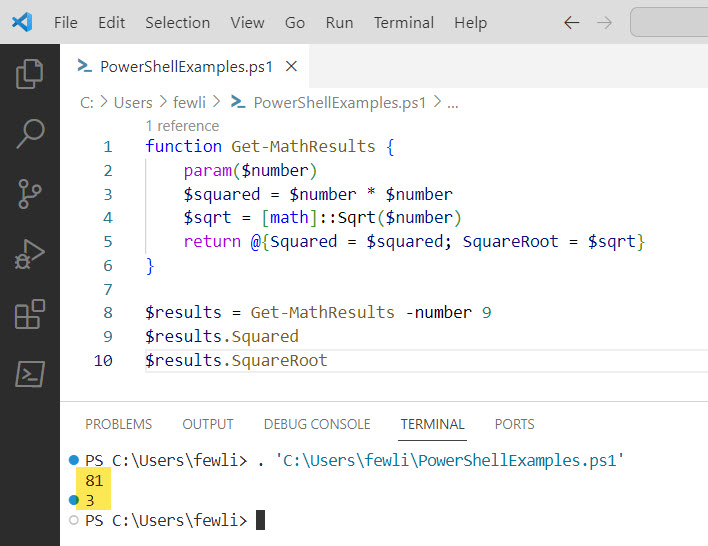
PowerShell Function Return Array
Arrays are useful when you want to return a collection of items. Here’s how you can return an array from a function:
function Get-EvenNumbers {
param($max)
$evenNumbers = 2..$max | Where-Object { $_ % 2 -eq 0 }
return $evenNumbers
}
$evenNumbersUpToTen = Get-EvenNumbers -max 10
$evenNumbersUpToTen
The Get-EvenNumbers
function returns an array of even numbers up to the specified maximum.
Conclusion
PowerShell functions can return values in various ways. Whether you’re returning a single value, a string, a boolean, multiple values using a hashtable, or an array, PowerShell provides the flexibility to handle each scenario. In this PowerShell tutorial, I have explained about the PowerShell return values.
You may also:
- How to Exit a Function Without Stopping the Script in PowerShell?
- How to Call Function in If Statement in PowerShell?
- PowerShell Function Array Parameters
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com