This is a very common requirement to exit a function without terminating the entire PowerShell script. In this PowerShell tutorial, I will explain how to exit a function without stopping the script in PowerShell.
To exit a function in PowerShell without terminating the entire script, you can use the return
keyword within the function. This will stop the execution of the current function and hand control back to the script, allowing the rest of the script to continue running. For example, using return
by itself will simply exit the function, while return $value
will exit and pass the value back to the calling code.
Exit a Function Without Stopping the Script in PowerShell
To exit a function in PowerShell, you can use the return
keyword. When PowerShell encounters return
, it immediately stops executing the current function and returns control to the calling scope.
Here’s a simple example:
function Get-Result {
$value = 10
# Condition to exit the function
if ($value -eq 10) {
return
}
# This line will not be executed if the condition above is met
Write-Host "Value is not 10"
}
# Calling the function
Get-Result
# The script continues here
Write-Host "Function has exited, but script continues..."
In this example, the script will output “Function has exited, but script continues…” and not “Value is not 10” because the function exited before it could reach that line.
Once you run the PowerShell script, you can see the output in the screenshot below:
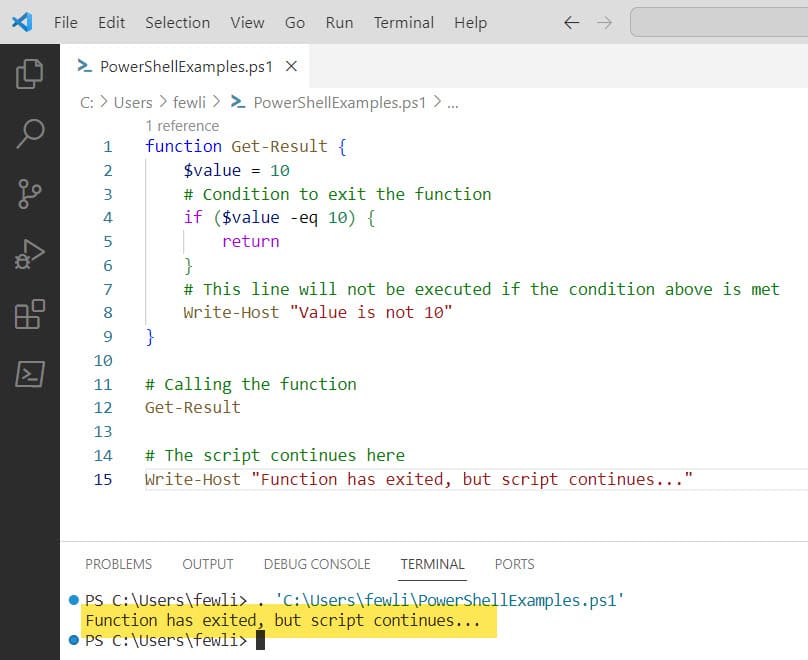
Using return to Exit with a Value in PowerShell function
The return
keyword can also be used to exit a function in PowerShell and pass a value back to the caller.
function Get-Multiplier {
param ($number)
# If the number is greater than 100, return it multiplied by 2
if ($number -gt 100) {
return $number * 2
}
# Otherwise, just return the number
return $number
}
# Store the returned value in a variable
$result = Get-Multiplier -number 150
Write-Host "The result is $result"
In this case, the output will be “The result is 300” because the function returned 150 multiplied by 2.
Continuing a PowerShell Script After a Function Call
When you exit a function using return
, the rest of the script will continue to run. This is different from using exit
, which will terminate the entire script or PowerShell session. It’s important to understand this distinction to control the flow of your scripts effectively.
Here are some additional tips for controlling script flow:
- Use
return
to exit a function and optionally return a value. - Use
exit
to terminate the entire script (or PowerShell session if not running a script). - Use
break
to exit a loop or a switch statement.
Conclusion
Understanding how to exit a function without stopping the entire PowerShell script is crucial when writing PowerShell scripts. Using return
allows you to control the flow of your functions and ensure that your scripts continue to run as intended. In this PowerShell tutorial, I have explained how to exit a function without stopping the script in PowerShell.
You may also like:
- PowerShell If Date Is Older Than 30 Days
- How to Get-Date Without Time in PowerShell?
- How to Replace Semicolon with Comma in PowerShell?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com