In this PowerShell tutorial, I will show you how to call a function in an if statement in PowerShell.
In PowerShell, you can call a function within an if
statement to execute code conditionally based on the function’s return value. For example, if you have a function called Get-Status
that returns $true
or $false
, you can use it in an if
statement like this:
if (Get-Status) {
# Code to execute if the function returns $true
}
This allows you to perform complex checks and execute conditional logic cleanly and efficiently within your scripts.
PowerShell call function in if statement
An if
statement in PowerShell evaluates an expression placed within parentheses. If the expression evaluates to $true
, the code block following the statement is executed. If the expression evaluates to $false
, the code block is skipped.
Here’s a simple example of an if
statement:
$condition = $true
if ($condition) {
Write-Host "The condition is true!"
}
In the above code, since $condition
is $true
, the message “The condition is true!” will be printed to the console.
In PowerShell, functions are blocks of code that you can reuse. They can take input, process it, and return the result. You can call a function inside an if
statement to evaluate its return value as part of the condition.
Here’s a basic function definition:
function Test-Function {
param($value)
return $value -eq 'PowerShell'
}
This function, Test-Function
, takes a parameter $value
and returns $true
if $value
is equal to the string ‘PowerShell’.
Example 1: Function Call Directly in If Statement
You can call this PowerShell function directly within an if
statement like so:
if (Test-Function -value 'PowerShell') {
Write-Host "The value is PowerShell!"
}
In this example, if you pass ‘PowerShell’ to Test-Function
, the if
statement will execute the Write-Host
command.
Here is the complete PowerShell script:
function Test-Function {
param($value)
return $value -eq 'PowerShell'
}
if (Test-Function -value 'PowerShell') {
Write-Host "The value is PowerShell!"
}
You can see the output in the screenshot below after I executed the PowerShell script using VS code.
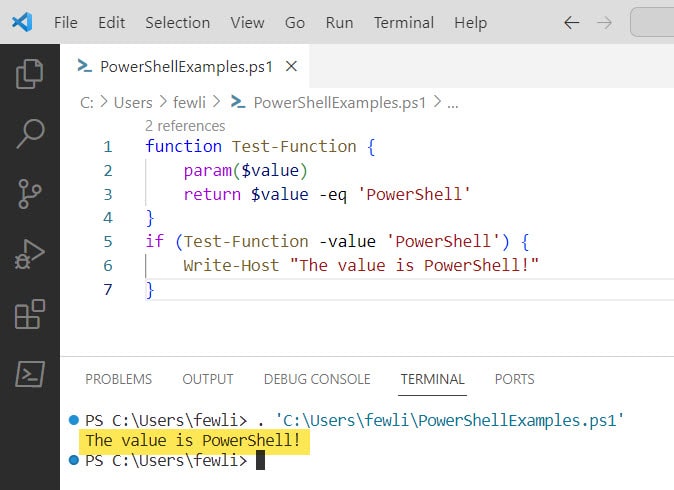
Example 2: Function Call with Comparison Operator
Sometimes, you might want to compare the function’s return value using a comparison operator. Here’s how you can do that:
$result = Test-Function -value 'Script'
if ($result -eq $true) {
Write-Host "The value is PowerShell!"
} else {
Write-Host "The value is not PowerShell!"
}
In this case, since ‘Script’ is not equal to ‘PowerShell’, the else
block will be executed, printing “The value is not PowerShell!” to the console.
Example 3: Using Function Call with Logical Operators
You can also use logical operators with function calls in if
statements to create more complex conditions:
function Check-Number {
param($number)
return $number -gt 10
}
$number = 15
if (Check-Number -number $number -and $number -lt 20) {
Write-Host "The number is greater than 10 and less than 20."
}
If the number is greater than 10 and less than 20, the message will be printed to the console.
Conclusion
In this PowerShell tutorial, I have explained how to call a function in an if statement in PowerShell with a few examples.
You may also like:
- PowerShell If Date Is Older Than 30 Days
- How to Get Current Function Name in PowerShell?
- Set Environment Variables Using PowerShell
- How to Replace String in PowerShell
- PowerShell Function With Parameters
- PowerShell Function Return Values
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com