Do you want to know how to replace string in PowerShell? In this PowerShell tutorial, I will explain in detail “PowerShell replace string” with multiple examples.
To replace a string in PowerShell, use the -replace
operator followed by the pattern you want to match and the replacement string. For example, $string -replace 'oldString', 'newString'
will replace ‘oldString’ with ‘newString’ in $string
. For a simple, case-sensitive replacement, you can use the .Replace()
method: $string.Replace('oldString', 'newString')
. For more complex patterns, regex can be used with the -replace
operator for advanced string manipulation.
PowerShell replace string using -replace operator
PowerShell provides a powerful operator -replace
that allows you to replace text within a string using a regular expression (regex). The -replace
operator is case-insensitive by default, which means it does not differentiate between uppercase and lowercase characters when searching for the pattern to replace.
# Syntax:
# 'StringToSearch' -replace 'PatternToFind', 'ReplacementString'
# Example:
$text = 'Hello World'
$newText = $text -replace 'World', 'PowerShell'
Write-Output $newText # Output: Hello PowerShell
Once you run the PowerShell script, you can see the output in the screenshot below.
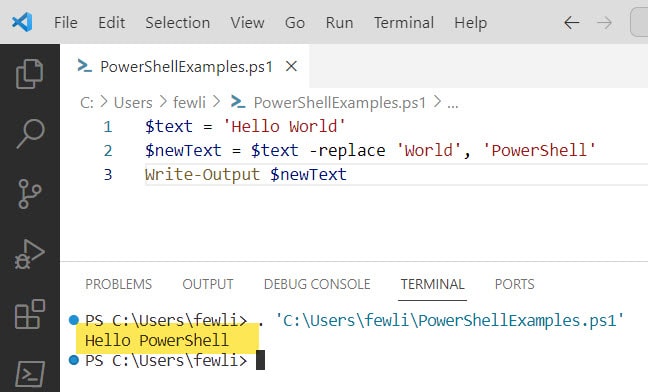
The -replace
operator uses regex, so you can perform complex pattern matching and replacements. For example, if you want to replace all digits in a string with a #
symbol, you could do the following:
$text = 'Order123'
$newText = $text -replace '\d', '#'
Write-Output $newText # Output: Order###
Replace string in PowerShell using the replace() method
You can use the .Replace()
method, which is a case-sensitive method from the .NET String class. This method does not use regex, and it only allows for simple direct string replacement.
# Syntax:
# 'StringToSearch'.Replace('OldValue', 'NewValue')
# Example:
$text = 'Good Morning'
$newText = $text.Replace('Morning', 'Evening')
Write-Output $newText # Output: Good Evening
The PowerShell .Replace()
method is straightforward and is best used when you need a simple replacement without the complexity of regex patterns.
PowerShell replace string at position
In PowerShell, if you need to replace a string at a specific position, you won’t use a direct built-in function like -replace
or .Replace()
, as these are designed for pattern matching rather than index-based replacement. Instead, you can use a combination of string methods to achieve this.
Here’s a step-by-step method to replace a string at a specific position:
- Use the
.Substring()
method to extract parts of the string before and after the position where the replacement needs to be made. - Concatenate the first part with the replacement string.
- Concatenate the result with the remaining part of the original string.
Here’s an example in PowerShell:
# Define the original string
$originalString = "Hello World"
# Define the replacement string
$replacementString = "PowerShell"
# Define the position to start the replacement
$startPosition = 6
# Perform the replacement
$firstPart = $originalString.Substring(0, $startPosition)
$secondPart = $originalString.Substring($startPosition + $replacementString.Length)
# Concatenate the parts with the replacement string
$newString = $firstPart + $replacementString + $secondPart
# Output the result
Write-Output $newString # Output: Hello PowerShell
In this example, “World” starts at position 6 in “Hello World”, and we’re replacing it with “PowerShell”. The .Substring()
method is used to split the string into two parts: before and after “World”. We then concatenate “Hello ” with “PowerShell” and the rest of the original string, if any.
Replace string at end in PowerShell
To replace a string at the end of another string in PowerShell, you can use the -replace
operator with a regular expression that targets the end of the string. The dollar sign $
in a regular expression indicates the end of a line or string. Here’s how you can use it:
# Original string
$originalString = "This is a test string test"
# String to find at the end
$stringToEnd = "test"
# Replacement string
$replacementString = "example"
# Replace the string at the end
$modifiedString = $originalString -replace "$stringToEnd$", $replacementString
# Output the new string
Write-Output $modifiedString
In this example, the -replace
operator looks for the word “test” at the end of $originalString
. The pattern "test$"
specifies that it should only match “test” when it appears at the end of the string. The matched text is then replaced with “example”.
Once you run the PowerShell script, you can see the output in the below screenshot.
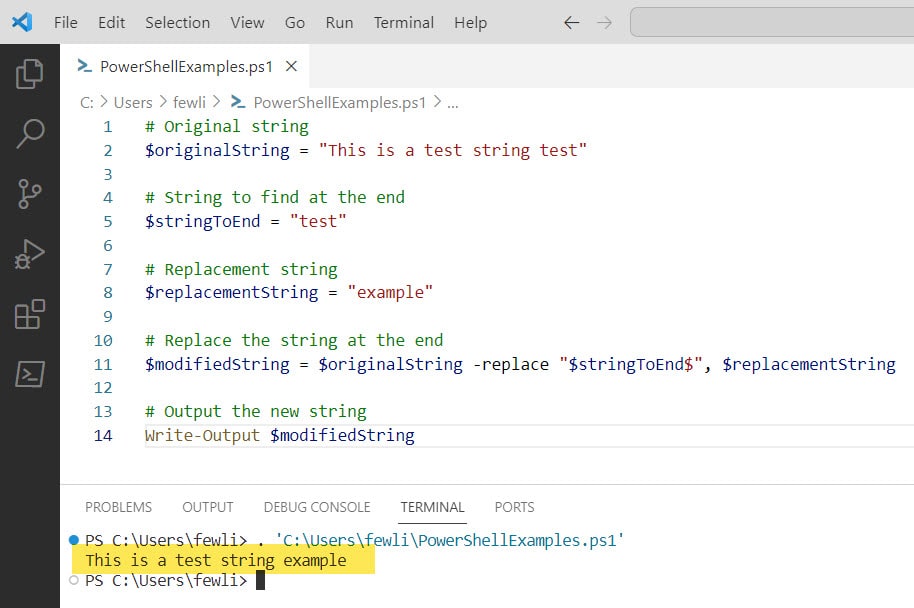
This is how to replace a string at the end in PowerShell.
PowerShell replace string between two words
To replace a string located between two specific words in PowerShell, you can use the -replace
operator with a regular expression. The regex pattern will include the two words as anchors with a wildcard pattern in between that matches any characters that occur between them.
Here’s the general approach:
# Original string
$originalString = "Start of the sentence to be replaced end of the sentence"
# Words that define the boundaries of the string to be replaced
$startWord = "Start"
$endWord = "end"
# Replacement string
$replacementString = "new content"
# Replace the string between the two words
$modifiedString = $originalString -replace "(?<=\b$startWord\b).*?(?=\b$endWord\b)", $replacementString
# Output the new string
Write-Output $modifiedString # "Start new content end of the sentence"
Once you execute the code, you can see the output in the screenshot below.
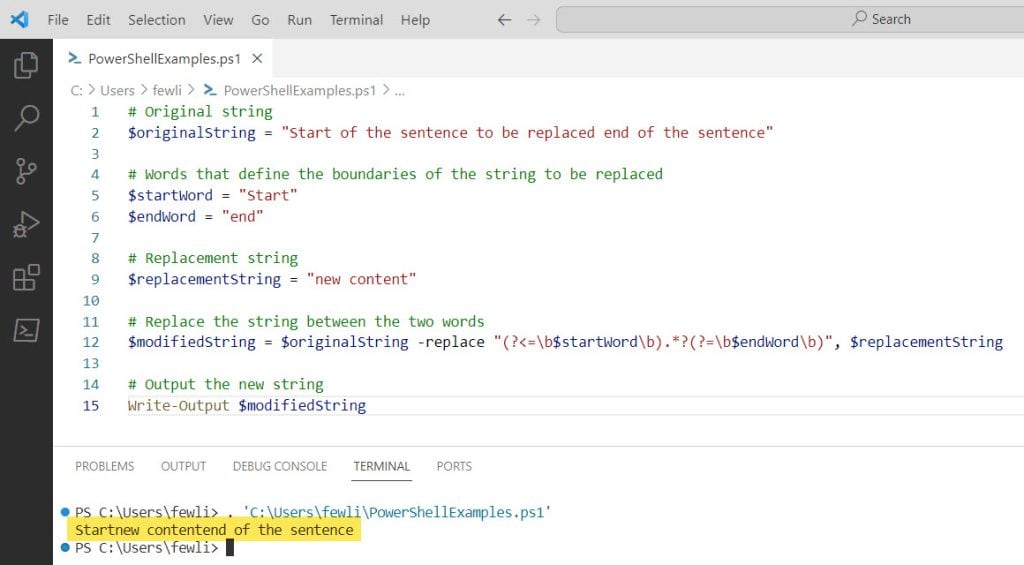
This is how to replace string between two words in PowerShell.
PowerShell replace string beginning with
In PowerShell, to replace a string that begins with a specific pattern, you can use the -replace
operator along with a regular expression that targets the beginning of the desired text. The caret symbol ^
in a regular expression signifies the start of a string or line.
Here’s how to use this in a PowerShell command:
# Original string
$originalString = "TextToBeReplaced Rest of the string"
# Pattern that the string starts with
$beginningPattern = "TextToBeReplaced"
# Replacement string
$replacementString = "NewText"
# Replace the string that begins with the pattern
$modifiedString = $originalString -replace "^$beginningPattern", $replacementString
# Output the new string
Write-Output $modifiedString # "NewText Rest of the string"
In this example, the pattern ^TextToBeReplaced
is used with the -replace
operator. The ^
anchors the pattern to the start of the string, ensuring that TextToBeReplaced
is only matched if it is at the very beginning of $originalString
. The matched text is then replaced with NewText
.
Once you execute the code, you can see the output in the screenshot below:
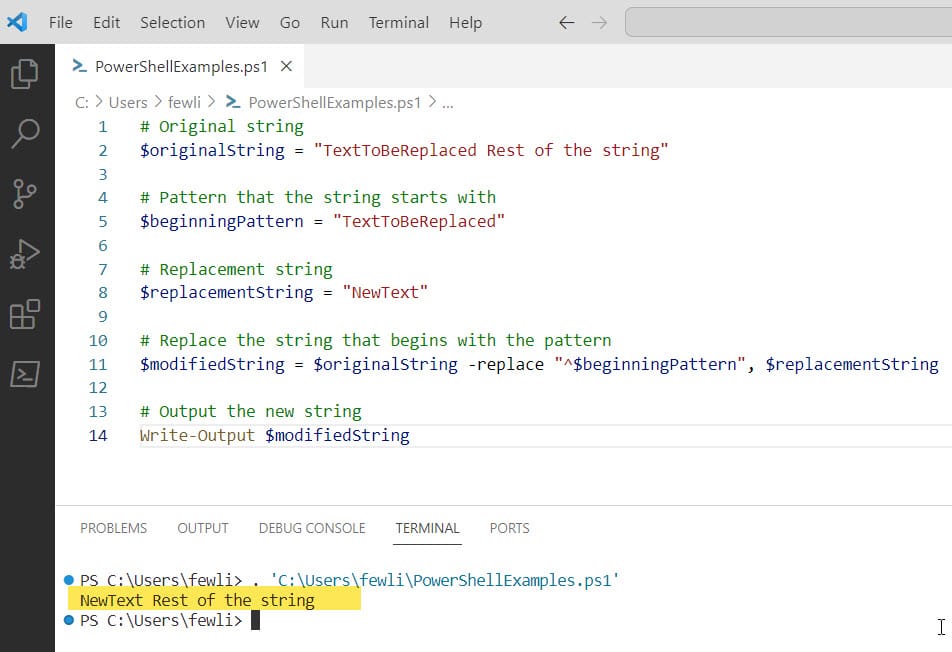
PowerShell replace string brackets
Replacing strings that contain brackets in PowerShell can be a bit tricky because brackets ([]
) have special meanings in regular expressions—they define a character class. To replace strings that contain square brackets, you need to escape them by prefixing them with a backslash (\
) in your pattern.
Here’s an example of how to replace a string that includes square brackets in PowerShell:
# Original string
$originalString = "This is a [sample] string with [brackets]."
# String to find (with brackets escaped)
$stringToFind = "\[sample\]"
# Replacement string
$replacementString = "(example)"
# Replace the string that includes brackets
$modifiedString = $originalString -replace $stringToFind, $replacementString
# Output the new string
Write-Output $modifiedString # "This is a (example) string with [brackets]."
In this example, the $stringToFind
is \[sample\]
, where the square brackets are escaped with backslashes. The -replace
operator uses this pattern to find the substring [sample]
in $originalString
and replace it with (example)
.
Here, you can see the output in the screenshot below after I executed the PowerShell script using Visual Studio code.
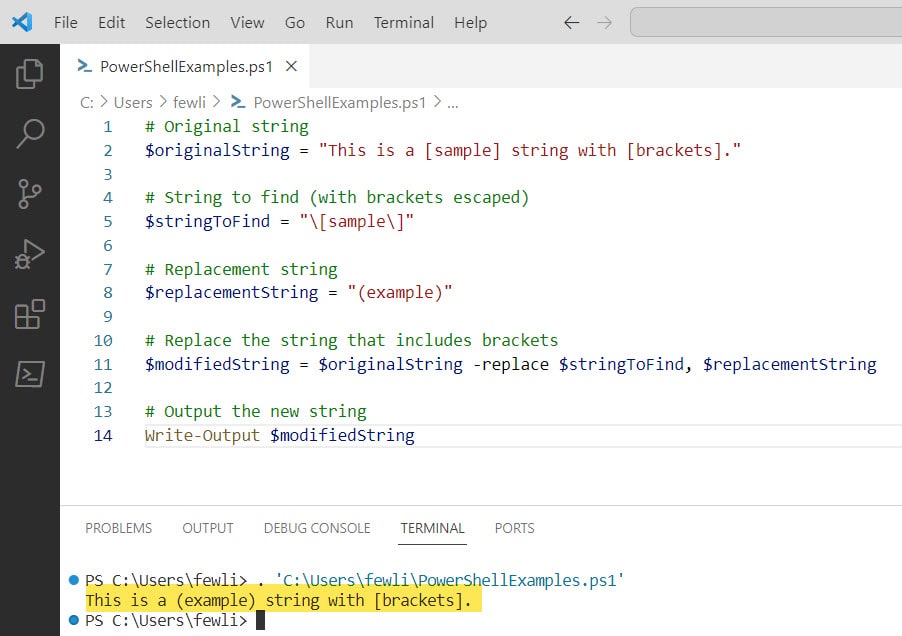
If you want to replace all occurrences of strings within brackets, you can use a regex pattern like '\[.*?\]'
:
# Replace all strings within brackets
$modifiedString = $originalString -replace '\[.*?\]', '(example)'
# Output the new string
Write-Output $modifiedString # "This is a (example) string with (example)."
The pattern \[.*?\]
uses a non-greedy match (the ?
after .*
) to replace everything between the first opening bracket [
and the closest closing bracket ]
with (example)
. The non-greedy match ensures that it doesn’t match across multiple sets of brackets.
PowerShell replace string by index
In PowerShell, replacing a string by index means that you want to change the content of a string starting at a particular character position. Since PowerShell doesn’t have a built-in method specifically for index-based replacement, this can be achieved by combining the .Substring()
method to split the original string and then concatenate the parts with the new string.
Here’s a step-by-step explanation of how to replace part of a string by index:
- Determine the indexes: Decide the starting index and the length of the substring you want to replace.
- Extract the substrings: Use
.Substring()
to get the parts of the string before and after the segment you want to replace. - Concatenate the new string: Combine the first part, the new string, and the second part to form the full string with the replacement.
Here’s an example in PowerShell:
# Define the original string
$originalString = "Hello World"
# Define the new string to insert
$newString = "Universe"
# Define the start index and length of the substring to replace
$startIndex = 6
$lengthToReplace = 5 # 'World' has 5 characters
# Extract the parts of the original string
$firstPart = $originalString.Substring(0, $startIndex)
$secondPart = $originalString.Substring($startIndex + $lengthToReplace)
# Combine the parts with the new string
$modifiedString = $firstPart + $newString + $secondPart
# Output the modified string
Write-Output $modifiedString # "Hello Universe"
In this example, we replace the word ‘World’ (which starts at index 6 and has a length of 5 characters) with the word ‘Universe’. We use .Substring(0, $startIndex)
to get the part of the string before ‘World’, and .Substring($startIndex + $lengthToReplace)
to get the part of the string after ‘World’. We then concatenate these parts with ‘Universe’ to form the new string.
PowerShell replace string at beginning of line
In PowerShell, to replace a string at the beginning of a line within a larger block of text or within a file, you can use the -replace
operator with a regular expression that targets the start of a line. The caret symbol ^
in a regular expression signifies the start of a string, and when used with the multiline mode modifier (?m)
, it can match the start of any line within a multiline string.
Here’s an example of how to replace a string at the beginning of a line:
# Multiline string
$multiLineString = @"
This is the first line.
Start This is the second line.
This is the third line.
Start This is the fourth line.
"@
# String to find at the beginning of a line
$stringToFind = "Start"
# Replacement string
$replacementString = "Begin"
# Replace the string at the beginning of a line
$modifiedString = $multiLineString -replace "(?m)^$stringToFind", $replacementString
# Output the new string
Write-Output $modifiedString
In this example, the pattern (?m)^Start
includes the multiline mode modifier (?m)
, which changes the behavior of the caret ^
to match the start of any line, not just the start of the string. The Start
string is then replaced with Begin
at the beginning of each line where it is found.
Conclusion
In this PowerShell tutorial, I have explained how to replace string in PowerShell using replace() method. Also, I have shown a lot of examples related to PowerShell replace string.
You may also like:
- How to Replace a String in an Array Using PowerShell?
- PowerShell Function With Parameters
- Replace Strings Containing Backslashes in PowerShell
- Replace Carriage Returns in Strings Using PowerShell
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com