Backslashes are often used in file paths and as escape characters, which can make string replacement a bit tricky. In this tutorial, we will explore how to replace strings containing backslashes using PowerShell.
To replace a string containing a backslash in PowerShell, you need to escape each backslash with another backslash in the string you want to replace. Use the -Replace
operator with the pattern and replacement strings. For example, $replacedString = $originalString -Replace 'C:\\\\OldPath', 'D:\\\\NewPath'
will replace C:\OldPath
with D:\NewPath
in the $originalString
.
Replace Strings Containing Backslashes in PowerShell
In PowerShell, the backslash (\
) is an escape character. This means that it is used to denote special characters or to allow special characters to be used as literals. For example, if you want to include a double quote inside a string that is enclosed in double quotes, you would use a backslash to escape it: "He said, \"Hello\"."
When dealing with strings that contain backslashes, you need to escape each backslash with another backslash. For example, a file path like C:\Folder\File.txt
would be represented as C:\\Folder\\File.txt
in a PowerShell string.
Using the -Replace Operator
PowerShell offers a -Replace
operator that can be used to replace text in a string. Here’s the basic syntax:
$string -Replace 'pattern', 'replacement'
Let’s say we want to replace the file path C:\Folder\Old
with D:\NewFolder\New
. Here’s how you would do it:
$path = 'C:\Folder\Old'
$newPath = $path -Replace 'C:\\Folder\\Old', 'D:\\NewFolder\\New'
Write-Output $newPath
Once you run the PowerShell script using VS code, you can see the output in the screenshot below.
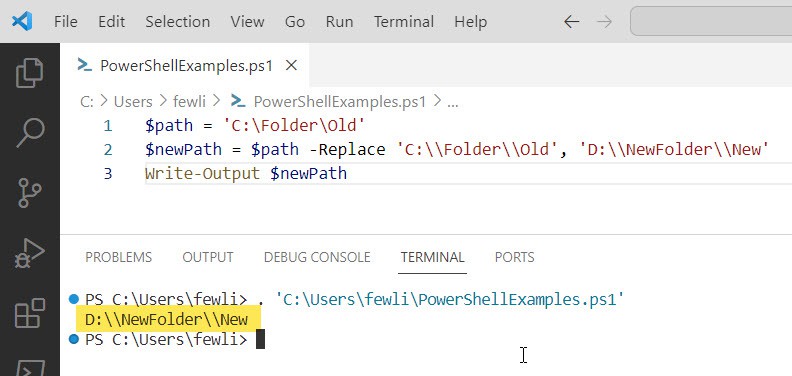
Using the [regex]::Escape() Method
If you’re dealing with strings that contain many backslashes or special characters, manually escaping each one can be error-prone. Instead, you can use the [regex]::Escape()
method to escape the entire string automatically in PowerShell:
$path = 'C:\Folder\Old'
$escapedPath = [regex]::Escape($path)
$newPath = $escapedPath -Replace 'C:\\\\Folder\\\\Old', 'D:\\NewFolder\\New'
Write-Output $newPath
Note that when using [regex]::Escape()
, the backslashes in the output are doubled, so you need to double the backslashes in your replacement pattern as well.
Replacing Strings in Files
To replace strings containing backslashes in files, you can combine the -Replace
operator with the Get-Content
and Set-Content
cmdlets in PowerShell:
$file = 'C:\path\to\your\file.txt'
$content = Get-Content $file -Raw
$newContent = $content -Replace 'C:\\\\Folder\\\\Old', 'D:\\NewFolder\\New'
Set-Content -Path $file -Value $newContent
Using the -Raw
parameter with Get-Content
reads the file content as a single string, which is necessary for multiline replacements.
Conclusion
Replacing strings containing backslashes in PowerShell requires an understanding of how the escape character works. By using the -Replace
operator and, if necessary, the [regex]::Escape()
method, you can accurately target and replace strings with backslashes in them in PowerShell. In this PowerShell tutorial, I have explained how to replace strings containing backslashes in PowerShell.
You may also like:
- PowerShell Get-Date To String
- How to Replace String Containing Double Quotes in PowerShell?
- PowerShell Replace String Case Insensitive
- How to Replace Semicolon with Comma in PowerShell?
- Replace String In JSON File Using PowerShell
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com