In PowerShell, you often need to manipulate strings, and one common task is replacing text within a string. Sometimes, you want to perform a replacement without worrying about the case (uppercase or lowercase) of the text. Let’s learn how to do a case-insensitive string replacement in PowerShell.
To perform a case-insensitive string replacement in PowerShell, you can use the -replace
operator, which is inherently case-insensitive. For example, $text = 'PowerShell'; $text -replace 'powershell', 'Bash'
will output ‘Bash’. If you need to ensure case sensitivity, use the -creplace
operator instead.
PowerShell Replace String Case Insensitive
PowerShell provides a -replace
operator that supports regular expressions and is, by default, case-insensitive. This makes it a powerful tool for string replacement tasks.
Example:
$text = "Hello World!"
$newText = $text -replace 'world', 'Universe'
Write-Host $newText
This will output Hello Universe!
even though the case of ‘world’ doesn’t match the case in the original string.
Make -replace Case Sensitive
If you need to make the -replace
operator case-sensitive, you can use -creplace
.
Example:
$text = "Hello World!"
$newText = $text -creplace 'world', 'Universe'
Write-Host $newText
In this case, the output will remain Hello World!
because ‘world’ does not match the case of ‘World’ in the original string.
Using .Replace()
Method
Another way to replace text in a string is by using the .Replace()
method of a string object. However, it’s important to note that this method is case-sensitive.
To perform a case-insensitive replacement using the .Replace()
method in PowerShell, you would first need to use a regular expression with the -match
operator or another method to identify the text to replace.
Example of Case-Insensitive Replacement with .Replace()
Here is a complete example of “PowerShell Replace String Case Insensitive”.
$text = "Hello World!"
$pattern = 'world'
$replacement = 'Universe'
if ($text -imatch $pattern) {
$regex = [regex]::new($pattern, 'IgnoreCase')
$newText = $regex.Replace($text, $replacement)
Write-Host $newText
}
This will output Hello Universe!
as it uses a regular expression with the IgnoreCase
option to perform a case-insensitive match and then replace.
You can see the output after I run the PowerShell script using VS code.
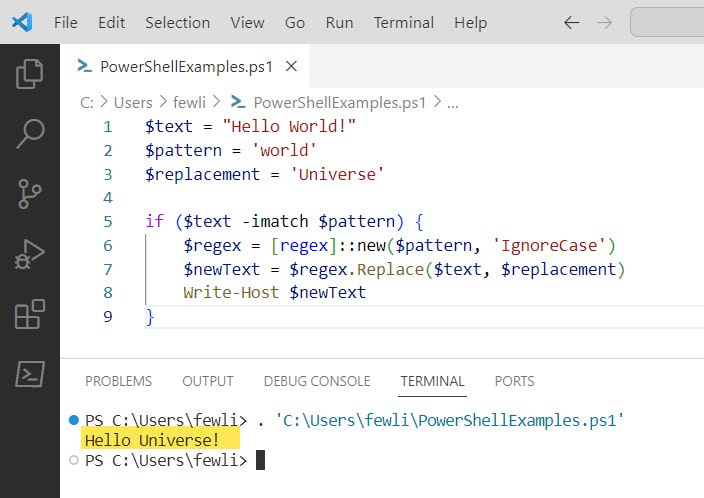
Summary
To replace a string in PowerShell without considering the case:
- Use the
-replace
operator for a simple, straightforward replacement. - Use
-creplace
if you need the replacement to be case-sensitive. - For the
.Replace()
method, use additional steps to handle case-insensitive scenarios.
Remember that the -replace
operator is your best friend for a quick and easy case-insensitive replacement in PowerShell.
You may also like:
- Replace Semicolon with Comma in PowerShell
- How to Replace String in PowerShell
- PowerShell Replace with Regular Expressions
- PowerShell Replace() Method
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com