The replace function in PowerShell is used to substitute characters or strings with new ones, which can be particularly useful when you need to update text or data dynamically. In this PowerShell tutorial, I will show you how to use the PowerShell replace() method with various examples.
The PowerShell Replace() method is a string method that allows for case-sensitive replacement of specified characters or strings within a given string. It is straightforward to use and does not support regular expressions. For example, $text = “Hello World”.Replace(“World”, “PowerShell”) would result in $text containing “Hello PowerShell”. This method is ideal for simple text replacements when the exact case of the text to be replaced is known.
Replace() method in PowerShell
The .Replace()
method is a straightforward way to replace text in a string. This method is case-sensitive and is a part of the string object in PowerShell, which means it can be called directly on any string.
Here’s a simple example:
$string = "Hello, World!"
$newString = $string.Replace("World", "PowerShell")
Write-Output $newString
This will output:
Hello, PowerShell!
In this example, we replaced the word “World” with “PowerShell” in the string $string
. You can see the output in the screenshot below:
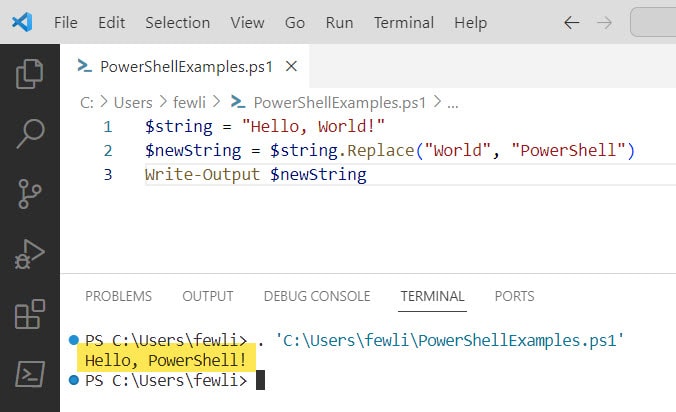
The .Replace()
method is case-sensitive. If you need to perform a case-insensitive replacement using this method, you’ll need to manipulate the string to have a uniform case before performing the replace operation.
PowerShell -replace Operator
Another way to replace text in PowerShell is by using the -replace
operator. Unlike the .Replace()
method, -replace
is case-insensitive by default and uses regular expressions, which makes it more powerful and flexible.
Here’s how you can use it:
$string = "Hello, World!"
$newString = $string -replace "world", "PowerShell"
Write-Output $newString
This will output the same result as before, even though “world” is in lowercase:
Hello, PowerShell!
You can also use regular expressions with the -replace
operator for more complex patterns. For example, if you want to replace all digits in a string with a #
symbol, you can do the following:
$string = "Order 12345"
$newString = $string -replace "\d", "#"
Write-Output $newString
This will output:
Order #####
The \d
is a regular expression that matches any digit.
With the -replace
operator, you can chain replacements to substitute multiple patterns in one go. Here’s an example:
$string = "Hello, World! Welcome to PowerShell."
$newString = $string -replace "Hello", "Hi" -replace "World", "Everyone"
Write-Output $newString
This will output:
Hi, Everyone! Welcome to PowerShell.
Conclusion
The replace function in PowerShell is a powerful feature that allows you to modify strings efficiently. Whether you use the .Replace()
method for simple, case-sensitive replacements or the -replace
operator for more complex, case-insensitive, and regex-based substitutions, understanding how to replace text is crucial for any PowerShell scripter.
You may also like:
- PowerShell Get-Date To String
- PowerShell Get-Date Add Days
- PowerShell Functions
- PowerShell unblock-file
- PowerShell Foreach With Examples
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com