If you are working in PowerShell, then you will get requirements to use looping to iterate items in a collection. In this tutorial, I will explain how to use PowerShell foreach loop with various real examples.
The foreach loop in PowerShell takes a collection of items and iterates over each item sequentially. It assigns each item to a temporary variable and executes a block of statements for every single item in the collection. The basic syntax of the foreach loop includes the temporary variable, the collection, and the script block containing the commands to be executed.
Now, let us understand how to use foreach loop in PowerShell with various examples.
Understanding PowerShell Foreach
In PowerShell, the ‘foreach’ loop is a fundamental construct that provides an efficient method to iterate over a collection of objects. This control statement is both powerful and essential for automating repetitive tasks.
Difference Between Foreach and Foreach-Object
Foreach is a statement in PowerShell used to execute a block of code for each item in a collection. In comparison, Foreach-Object is a cmdlet that streams objects through the pipeline, one at a time, and performs an operation on each.
The distinction lies in execution: the foreach statement in PowerShell processes items in memory, which can be faster, but Foreach-Object operates within the pipeline and is more memory efficient, suitable for handling large sets of data.
PowerShell Foreach Loop Structure
The structure of a foreach loop is designed to simplify the process of performing actions on each item of an iterable collection (like arrays or lists). An iterator represents each item as the loop progresses, allowing the code block inside the loop to manipulate or utilize the individual elements.
PowerShell Foreach Loop Syntax
The syntax of the foreach statement in PowerShell consists of a keyword followed by a pair of parentheses and braces. Here’s the syntax:
foreach ($item in $collection) {
# Code to execute for each item
}
$item
serves as the variable that holds the current element from the collection during each iteration.$collection
represents the array or list of objects that the loop will iterate over.
Write Foreach Loops in PowerShell
Now, let us understand with a few examples how to use the foreach loops in PowerShell.
Basic Foreach Loop Examples
A foreach
loop in PowerShell is structured to process each item within a collection such as an array. It uses a simple syntax as shown below:
$collection = @(1, 2, 3, 4, 5)
foreach ($item in $collection) {
# Script block to execute
Write-Host $item
}
In this example, each number in the $collection
array is output to the host. You can see the output in the screenshot below:
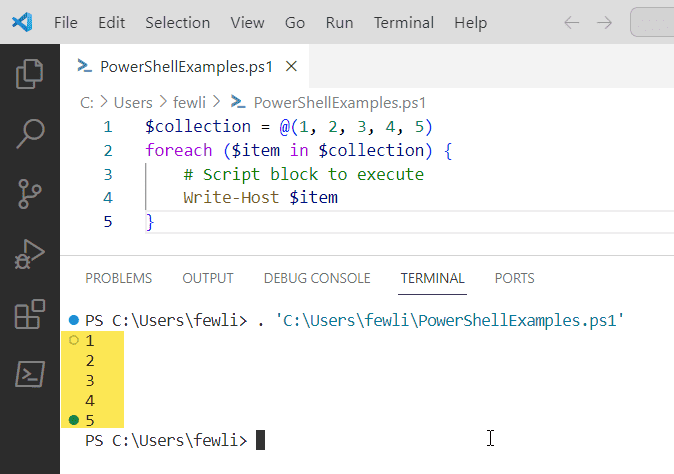
Using the $_ Variable in Loops
The $_
variable is a special variable in PowerShell that represents the current object in the pipeline. It’s most commonly used in conjunction with the ForEach-Object
cmdlet. Here’s how it is typically employed:
$collection | ForEach-Object {
Write-Host $_
}
Here, each item from $collection
is piped into ForEach-Object
and is accessed through the $_
variable.
Implementing Cmdlet Methods within Loops
Cmdlet methods can be integrated within a foreach
loop in PowerShell to act upon each item. This is especially powerful for managing a group of items using PowerShell cmdlets. An example would be iterating over a collection of files and renaming them:
$files = Get-ChildItem -Path "C:\MyFolder"
foreach ($file in $files) {
Rename-Item $file.FullName -NewName ("New_" + $file.Name)
}
The Rename-Item
cmdlet is used to prepend “New_” to each filename in the collection $files
.
Utilizing Pipeline and Cmdlets in PowerShell Foreach
PowerShell’s pipeline feature allows for efficient data management by enabling cmdlets to pass results directly to another cmdlet. The pipeline is particularly powerful when used with ForEach-Object
, and can be further refined with Select-Object
and Where-Object
for specialized data operations.
Using PowerShell Pipeline with Foreach-Object
The ForEach-Object cmdlet serves as a core component in harnessing the PowerShell pipeline’s capabilities. It processes each item passed from the pipeline individually, enabling actions to be performed on each item. For example:
Get-ChildItem -Path C:\Logs | ForEach-Object {
$_.LastWriteTime = (Get-Date)
}
In the PowerShell script above, Get-ChildItem
retrieves items from the specified path, which are then piped to ForEach-Object. Within the script block {}
, $_
represents each item iteratively, allowing modification of their properties, such as LastWriteTime
.
Optimizing Tasks with Select-Object and Where-Object Cmdlets
PowerShell’s Select-Object
and Where-Object
cmdlets serve as precision tools for filtering and shaping data within a pipeline.
Select-Object is utilized to select specific properties from objects:
Get-Process | Select-Object -Property ProcessName, CPU
This command displays a list of processes, but only outputs the ProcessName and CPU properties of each object.
On the other hand, Where-Object applies a filter criterion to include only items that meet a certain condition:
Get-Service | Where-Object { $_.Status -eq 'Running' }
Here, Get-Service
generates a list of services, which the Where-Object
cmdlet filters, passing through only those services currently running as indicated by their Status
property.
Advanced Foreach Loop Usage in PowerShell
Let us see some advanced usage of the PowerShell Foreach loop. Users can embed conditional logic and manage the file system more effectively through specialized implementations of PowerShell foreach loop.
Implementing Conditional Logic in Loops
The foreach
loop in PowerShell is capable of more complex tasks when combined with conditional logic such as if
statements. This allows the script to execute commands based on certain conditions within each iteration. For example, to process only specific objects from a collection:
$collection = Get-ChildItem -Path C:\Logs\*.log
foreach ($item in $collection) {
if ($item.Length -gt 1MB) {
# Process only files larger than 1MB
# [Perform action]
}
}
In the example above, the if
statement ensures that only files larger than 1MB are processed. This demonstrates how to selectively manipulate items in a collection for more advanced data handling.
Using Foreach for File and Directory Management
Leveraging the foreach
loop for file and directory management can streamline various tasks such as renaming, moving, or archiving files. Utilize the Get-ChildItem
cmdlet to obtain the collection of files or directories, then iterate and manage them:
$directories = Get-ChildItem -Path C:\Projects -Directory
foreach ($dir in $directories) {
# Use the foreach-method to execute a block of code for each directory
# [Perform actions on each directory, such as renaming]
}
To manage files within these directories, the script might incorporate the foreach-object
cmdlet in a pipeline to efficiently handle each file one by one without loading all items into memory simultaneously. This is particularly useful for large numbers of files or limited memory environments:
Get-ChildItem -Path C:\Projects\*.ps1 -File | ForEach-Object {
# Process each .ps1 file individually using $_ to represent the current file
# [Perform actions on each file]
}
These examples illustrate how one can apply sophisticated loop constructs to optimize file and directory operations in PowerShell scripts.
Improve Performance with Foreach loop in PowerShell
In PowerShell, the foreach loop is a common method for iterating through collections. Despite its usefulness, its performance can lag with large datasets. This section explains how to enhance the efficiency and speed of the foreach loop.
Parallel Processing with Foreach-Object -Parallel
The Foreach-Object -Parallel
feature introduced in PowerShell 7.0 allows for parallel execution of code blocks across multiple objects. It executes the script block for each item in the pipeline, simultaneously running in separate threads. This approach can significantly increase the efficiency of processing large numbers of items.
- Syntax:
$collection | Foreach-Object -Parallel {
# Script block to execute
} -ThrottleLimit <number>
- ThrottleLimit: One can specify the maximum number of concurrent threads with the
-ThrottleLimit
parameter. By careful adjustment, users may prevent over-utilization of system resources.
Monitoring Loop Performance with Measure-Command
The Measure-Command
cmdlet in PowerShell provides a convenient way to monitor the time taken by script blocks or commands. Users can wrap their foreach loop with this cmdlet to capture performance metrics, making it easier to benchmark and identify potential inefficiencies.
Example:
Measure-Command {
foreach ($item in $collection) {
# Code to execute for each item
}
}
By harnessing parallel processing and scrutinizing loop execution time with Measure-Command
, users can fine-tune their scripts to be more efficient and performant.
Error Handling and Debugging in Foreach loop
Let us see, how to do error handling and debugging in a PowerShell foreach loop with examples.
Error handling inside a Foreach Loop
When one iterates over a collection using a foreach loop, it’s possible that individual iterations could lead to errors. Trapping these errors is done by enclosing statements within a try..catch
block. Here’s an example of trapping errors in a PowerShell script block inside a foreach loop:
foreach ($item in $collection) {
try {
# Potentially problematic code here
}
catch {
# Error handling code here
}
}
In the try
block, the script executes the code that might throw an error, while in the catch
block, the script handles the error. For instance, if the code attempts to modify an object that does not exist, PowerShell generates an error, which is then caught by the catch block, allowing the script to continue or log the error without terminating.
Debugging Foreach Loops in Scripts
Debugging a foreach loop involves checking that each iteration of the loop runs as expected. In PowerShell scripts, one can use the following methods to debug foreach loops:
- Write-Host: This cmdlet can output the current value being processed in the loop, helping to identify at which point an error occurs.
foreach ($item in $collection) {
Write-Host "Processing item: $item"
# Foreach loop code here
}
- Breakpoints: Set breakpoints in the script to pause execution at certain lines, which is especially useful in Integrated Scripting Environment (ISE) or Visual Studio Code.
- Verbose Output: Use the
-Verbose
flag to provide detailed information about operations performed within the loop, including any errors.
Best Practices and Tips to use in foreach loop
When using PowerShell foreach loops, it is crucial to apply best practices to ensure code efficiency and maintainability. This section covers the vital nuances and techniques for achieving optimal results with foreach loops.
Effective Use of Begin, Process, and End Blocks
In PowerShell, the Begin
, Process
, and End
blocks are utilized to effectively structure code in a pipeline-optimized loop using ForEach-Object
. One should use the Begin
block for one-time preliminary actions, such as setting initial variables or runspace configurations, which are executed before processing any input objects.
Within the Process
block, the input objects are processed individually. This is where each object passed to the loop sees the action, and it runs once for each object in the pipeline. The End
block should contain commands that execute after all objects have been processed, such as cleanup tasks or reporting.
- Begin block: Set initial variables or runspace configurations.
- Process block: Execute actions for every single object.
- End block: Perform cleanup tasks or aggregations post processing.
Avoiding Common Mistakes in Foreach Loops
Common pitfalls in PowerShell foreach loops can be avoided by:
- Not modifying the collection being iterated over within the
foreach
loop, as it can lead to unexpected behavior. - Being cautious of the scope of variables inside and outside the
foreach
loop to prevent overwriting important data. - Ensuring the proper utilization of
$_
or$PSItem
automatic variables within theForEach-Object
cmdlet to access the current object in the pipeline.
Nested Foreach Loop Considerations
When using nested foreach loops in PowerShell, it is essential to:
- Manage scopes meticulously to avoid naming collisions and ensure variables are modified in the intended context.
- Be aware of the performance implications. Nested loops increase the complexity of the operation, which can lead to longer execution times, especially when handling large collections.
- Carefully consider the use of
begin
andend
blocks in each level of the nested loops to manage resources and variable scopes appropriately.
Frequently Asked Questions
What is the syntax for a foreach loop in PowerShell?
In PowerShell, the foreach loop syntax involves specifying the variable to represent each element, the collection to iterate over, and the script block of code to execute for each element. The structure looks like this:foreach ($element in $collection) { # Script block to execute }
Can you provide examples of using foreach loops in PowerShell?
Certainly, one can utilize a foreach loop to iterate through items and perform actions on them. For example, to print each number in an array:$numbers = 1..5 foreach ($number in $numbers) { Write-Host $number }
How do you iterate over an array using PowerShell’s foreach?
Iterating over an array with foreach in PowerShell is straightforward. One assigns the array to a variable and then passes through each item using a loop. For example:$colors = 'Red', 'Green', 'Blue' foreach ($color in $colors) { Write-Host $color }
What is the purpose of the $_ symbol in PowerShell scripts?
The $_
symbol in PowerShell scripts represents the current object in the pipeline and is used within script blocks, mainly with cmdlets like ForEach-Object
.
How does the ForEach-Object cmdlet differ from the foreach statement in PowerShell?
ForEach-Object
is a cmdlet used in pipeline operations for each item that is passed through the pipeline, while the foreach statement is a looping construct that iterates over a collection, which doesn’t necessarily come from the pipeline.
What are some common mistakes to avoid when using foreach loops in PowerShell?
Common mistakes include modifying the collection being iterated over inside the loop, which can lead to unexpected results, or inefficiently using foreach loops where a cmdlet could be more performant. It’s crucial to understand the context and choose the appropriate looping mechanism.
Conclusion
Using the foreach
loop in PowerShell allows a user to process items in a collection one by one efficiently. It has been demonstrated that this loop structure is essential for automating repetitive tasks and easily handling arrays or hashtables.
Best Practices:
- Define clear start and end conditions for the
foreach
loop to prevent infinite loops. - Utilize
$_
within the loop to reference the current object in the pipeline when usingForEach-Object
. - Consider memory management with large collections, as
foreach
can be memory-intensive.
In practice, a user can combine the foreach
loop with other cmdlets like Get-Process
to filter processes or with Get-Content
to read through the content of a file line by line. This seamless integration underpins the versatility of foreach
in scripting scenarios.
Example:
$processes = Get-Process
foreach ($process in $processes) {
if ($process.Name -like 'A*') {
Write-Host "$($process.Name) starts with A"
}
}
I hope now you learn how to use foreach loop in PowerShell.
You may also like:
- How to Add Comments in PowerShell
- How to Pass Objects to Functions in PowerShell?
- How to Set Environment Variables Using PowerShell?
- PowerShell unblock-file
- Foreach vs Foreach-Object in PowerShell
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com