There might have been scenarios where you want to replace a string containing special characters in PowerShell. In this tutorial, I will explain to you different examples of how to replace strings containing special characters in PowerShell.
To replace a string containing special characters in PowerShell, you can use the -replace operator with an escape character. For example, if you want to replace a string with a dollar sign $, you would use a backtick to escape it: $text -replace “\$oldString”, “newString”. For regex patterns, use a backslash to escape special regex characters, like so: $text -replace “file_\d{4}-\d{2}-\d{2}”, “newFileName”`.
Replace String Containing Special Characters in PowerShell
In the context of text processing, special characters are characters that have a specific meaning within a language or format. For example, in PowerShell (and many other programming languages), characters like $
, *
, ?
, and others are considered special because they serve as wildcards or have other control functions.
When you want to replace strings that contain these characters, you might run into issues because PowerShell will try to interpret them rather than treat them as literal characters. To avoid this, you need to escape these characters.
Escaping special characters in PowerShell can be done using the backtick character `
, which is PowerShell’s escape character. However, when working with regular expressions (regex), you will need to use a backslash \
to escape special characters.
Using the -replace Operator
PowerShell provides the -replace
operator for string replacement operations. Here’s a simple example:
# Replace a simple string
$text = "Hello World"
$newText = $text -replace "World", "PowerShell"
Write-Output $newText
This will output Hello PowerShell
.
Now, let’s say you have a string with a special character:
# Replace a string containing a special character
$text = "Hello `$World"
$newText = $text -replace "\`$World", "PowerShell"
Write-Output $newText
In this example, we escape the $
character with a backtick to ensure it is treated as a literal character. The output will be Hello PowerShell
.
You can see the screenshot below for the output after I run the script using VS code.
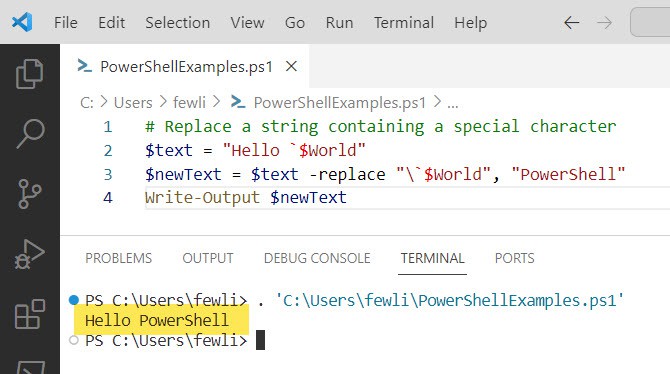
Using Regex with -replace
For more complex patterns and string replacements involving special characters, you can use regex with the -replace
operator. Here’s an example that uses regex to replace a string containing special characters:
# Using regex to replace a string with special characters
$text = "File name: file_2024-01-13.txt"
$newText = $text -replace 'file_\d{4}-\d{2}-\d{2}', 'document'
Write-Output $newText
In this regex pattern, \d{4}-\d{2}-\d{2}
matches a date format, and we replace it with the word ‘document’. The output will be File name: document.txt
.
Check the screenshot below:
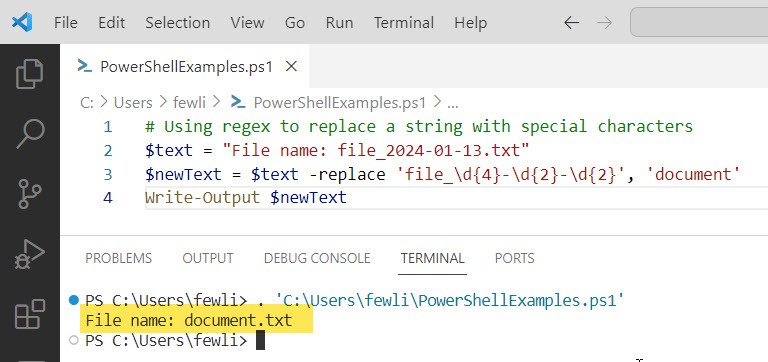
Handling Complex Patterns
Sometimes you may need to replace strings that contain a combination of literal text and special characters. Here’s how you can handle such situations:
# Complex pattern replacement
$text = "Contact support at support@example.com for assistance."
$newText = $text -replace 'support@example\.com', 'helpdesk@example.com'
Write-Output $newText
In the above example, we escape the dot .
in the email address because in regex, a dot matches any character. By escaping it, we ensure it matches an actual dot. The output will be Contact support at helpdesk@example.com for assistance.
Conclusion
Replacing strings, especially those containing special characters, is a common task in PowerShell scripting. By using the -replace
operator and understanding how to escape special characters, you can effectively manage and manipulate strings in your scripts. In this PowerShell tutorial, I have explained how to replace strings containing special characters in PowerShell with various examples.
You may also like:
- Replace Strings Containing Backslashes in PowerShell
- PowerShell Replace String Before Character
- Convert Array To Comma Separated String In PowerShell
- PowerShell Substring() Example
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com