PowerShell is a powerful scripting language that offers a variety of ways to manipulate strings. One common task is to replace parts of a string before a certain character. In this blog post, we’ll explore how to replace text in a string before a specific character using PowerShell.
I will also explain a few examples:
- Replace String Before Character in PowerShell
- How to Replace String After Character in PowerShell
- How to Replace String Between Two Characters in PowerShell
- Replace the last character in a string in PowerShell
PowerShell Replace String Before Character
PowerShell provides the -replace
operator, which is used for replacing text based on a regular expression pattern.
Example 1: Replace Everything Before a Colon
Let’s say you have a string that contains a time, and you want to replace everything before the colon (:) with the word “Hour”.
$string = "12:30 PM"
$newString = $string -replace '.*:', 'Hour:'
Write-Output $newString
This will output Hour:30 PM
. The .*:
pattern matches any character (.
) any number of times (*
) until it encounters a colon (:
), which it then replaces with “Hour:”.
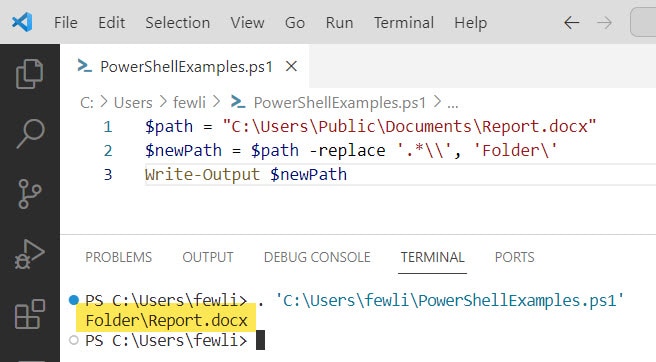
Example 2: Replace Everything Before a Slash
If you have a file path and you want to replace everything before the last backslash with “Folder”, you can use the following command:
$path = "C:\Users\Public\Documents\Report.docx"
$newPath = $path -replace '.*\\', 'Folder\'
Write-Output $newPath
The output will be Folder\Report.docx
. The pattern .*\\
uses \\
to match a literal backslash (since the backslash is an escape character in regular expressions, it must be escaped itself).
You can in the screenshot below:
Using the .Replace()
Method
For simple replacements that don’t require patterns, you can use the .Replace()
method. However, this method doesn’t directly support replacing text before a specific character. It’s more commonly used to replace exact strings.
$string = "Hello, World!"
$newString = $string.Replace("Hello", "Goodbye")
This will output Goodbye, World!
. But since .Replace()
doesn’t accept regular expressions, it’s not the best tool for replacing text before a character.
In some cases, you might want to remove characters from the beginning of a string rather than replace them. PowerShell offers the TrimStart()
method for this purpose.
If you have a string with a list of items separated by commas and you want to remove everything before the first comma, you can do:
$string = "Item1,Item2,Item3"
$newString = $string.Substring($string.IndexOf(',') + 1)
This will output Item2,Item3
. The IndexOf()
method finds the position of the first comma, and Substring()
is used to get the rest of the string starting from that position.
PowerShell Replace String After Character
In PowerShell, replacing a substring after a specific character involves using regular expressions with the -replace
operator.
Example: Replace Text After a Dash
Imagine you have a string with a dash, and you want to replace everything after the dash with “end”.
$string = "start-middle-end"
$newString = $string -replace '-.*', '-end'
This command will produce the output start-end
. The regex pattern '-.*'
matches a dash followed by any character (represented by .
) repeated any number of times (represented by *
).
Example: Replace Text After the First Occurrence of a Character
In some cases, you might want to replace text after the first occurrence of a character but keep subsequent characters intact.
$string = "item1,item2,item3"
$newString = $string -replace '^[^,]*,', 'item0,'
This will output item0,item2,item3
. The regex pattern ^[^,]*,
breaks down as follows:
^
asserts the position at the start of the string.[^,]*
matches any character except a comma ([^,]
) any number of times (*
).,
matches the comma character itself.
As a result, everything from the start of the string up to and including the first comma is replaced with item0,
.
Example: Replace Text After a Specific Word
If you want to replace text after a specific word, you can use a similar approach:
$string = "Error: The operation completed successfully."
$newString = $string -replace 'Error:.*', 'Error: An unexpected issue occurred'
The output will be Error: An unexpected issue occurred
. The pattern Error:.*
matches the word “Error:” and any text that follows it.
Example: Replace Text After a Colon
Another way to replace text after a specific character is to use the Substring()
method in combination with the IndexOf()
method in PowerShell.
$string = "Time: 12:30 PM"
$index = $string.IndexOf(':') + 1
$newString = $string.Substring(0, $index) + " 00:00 AM"
This will output Time: 00:00 AM
. Here’s what happens in the code:
IndexOf(':')
finds the position of the first colon.- Adding
1
to the index sets the starting point after the colon. Substring(0, $index)
extracts the text from the start of the string to the colon.- Concatenating
+ " 00:00 AM"
adds the new time to the extracted text.
PowerShell Replace String Between Two Characters
Replacing text between two specific characters in PowerShell can be accomplished using the -replace
operator with a regular expression (regex).
The -replace
operator in PowerShell allows you to specify a regex pattern to match the text you want to replace. Regex is a powerful tool for string manipulation that can match complex text patterns.
Example: Replace Text Between Parentheses
Consider a scenario where you have a string with text inside parentheses, and you want to replace the text within the parentheses with the word “Content”.
$string = "This is a sentence (with some words) in it."
$newString = $string -replace '\(.*?\)', '(Content)'
This command will produce the output This is a sentence (Content) in it.
The regex pattern '\(.*?\)'
breaks down as follows:
\(
and\)
match the literal parentheses characters. Parentheses are special characters in regex, so they need to be escaped with a backslash (\
)..*?
is a non-greedy match for any character (.
) repeated any number of times (*
), as few times as possible to make the regex match (?
), ensuring that only text between the closest pair of parentheses is matched.
Example: Replace Text Between Brackets
If you have a string with square brackets and you want to replace the content between them, you can use the following pattern:
$string = "This is a [sample] string."
$newString = $string -replace '\[.*?\]', '[replaced]'
The output will be This is a [replaced] string.
Here, \[
and \]
match the literal square brackets, and .*?
functions the same as in the previous example.
Example: Replace Text Between Two Specific Characters
To replace text between two specific characters, like a comma and a semicolon, you would use a pattern that matches those characters.
$string = "Start, replace this part; end."
$newString = $string -replace ',.*?;', ', new content;'
This will output Start, new content; end.
The pattern ',.*?;'
targets the text between the comma and the semicolon for replacement.
Replace the last character in a string in PowerShell
To replace the last character in a string in PowerShell, you can use the -replace
operator with a regular expression or manipulate the string using its methods such as Substring
.
Using the -replace
Operator with Regex
The -replace
operator can be used with a regex pattern that targets the last character of the string.
Here is an example.
$string = "Hello!"
$newString = $string -replace '.$', '?'
This command will replace the last character of $string
with a question mark, resulting in Hello?
. In the regex pattern:
.
matches any single character except a newline.$
asserts the position at the end of the string.
Together, '.$'
matches the last character in the string.
Using String Manipulation Methods
Another way to replace the last character of a string is by using the Substring
method to remove the last character and then appending the new character.
Here is an example.
$string = "Hello!"
# Remove the last character and store it in $newString
$newString = $string.Substring(0, $string.Length - 1)
# Append the new character
$newString += '?'
This will result in Hello?
. Here’s what happens in the code:
$string.Substring(0, $string.Length - 1)
takes a substring of$string
starting at index 0 and ending at the index just before the last character.+= '?'
appends the new character to the end of the modified string.
Both methods allow you to replace the last character in a string with PowerShell effectively. The -replace
operator with regex is concise and powerful for pattern matching, while string manipulation methods like Substring
offer a more direct approach when dealing with simple changes such as replacing the last character.
Replace multiple characters in a string in PowerShell
In PowerShell, to replace multiple characters in a string, you can use the -replace
operator or the .Replace()
method. The -replace
operator uses a regular expression for pattern matching, while the .Replace()
method is a straightforward string replacement that does not use regex.
Here’s an example using the -replace
operator:
# Using -replace operator with regex
$string = "Hello World, this is PowerShell"
$string -replace 'o', '0' -replace 'l', '1'
This script will output: He110 W0r1d, this is P0werShe11
. Check the screenshot below; I have already executed the script in VS code.
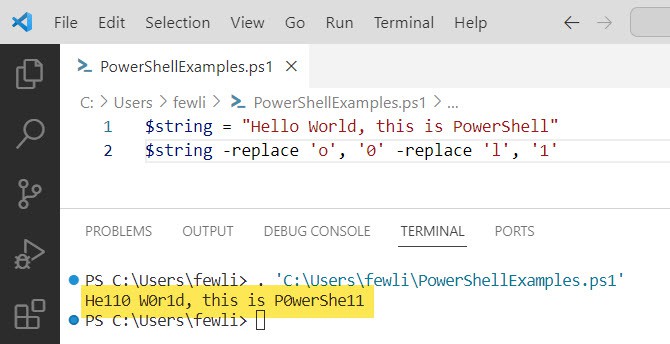
Here’s an example using the .Replace()
method:
# Using .Replace() method
$string = "Hello World, this is PowerShell"
$string = $string.Replace('o', '0').Replace('l', '1')
This script will also output: He110 W0r1d, this is P0werShe11
The .Replace()
method is case-sensitive and does not support regex, so it’s typically used for simple replacements. If you need case-insensitive or regex-based replacements, you should use the -replace
operator.
For more complex patterns and multiple replacements, you might need to chain the replacements, as shown in the examples above. If you want to replace multiple different characters with a single call, you can use a regex character class with the -replace
operator:
# Using -replace operator with regex character class
$string = "Hello World, this is PowerShell"
$string -replace '[aeiou]', '*'
This will replace all vowels with an asterisk, resulting in: H*ll* W*rld, th*s *s P*w*rSh*ll
PowerShell replace all occurrences in string
In PowerShell, to replace all occurrences of a substring within a string, you can use either the -replace
operator or the .Replace()
method. The -replace
operator is more powerful as it supports regular expressions, allowing for pattern matching and replacement, while the .Replace()
method is simpler and only replaces literal strings.
Here’s an example using the -replace
operator to replace all occurrences of “cat” with “dog”:
# Using -replace operator
$text = "The cat sat on the catwalk."
$newText = $text -replace 'cat', 'dog'
Write-Output $newText
This script will output: The dog sat on the dogwalk.
You can see the screenshot below:
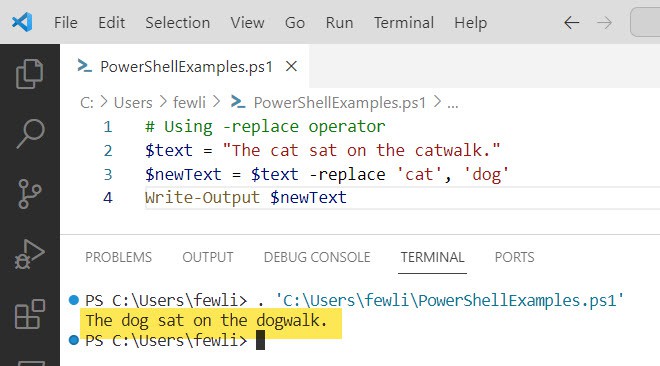
Here’s an example using the .Replace()
method:
# Using .Replace() method
$text = "The cat sat on the catwalk."
$newText = $text.Replace('cat', 'dog')
Write-Output $newText
This script will also output: The dog sat on the dogwalk.
The .Replace()
method is case-sensitive and only replaces exact matches, while the -replace
operator can be made case-insensitive by adding the i
modifier after the regex pattern: 'pattern','replacement','i'
.
Conclusion
In this PowerShell tutorial, I have explained how to replace string before character in PowerShell and the below examples.
- PowerShell Replace String Before Character
- PowerShell Replace String After Character
- PowerShell Replace String Between Two Characters
- Replace the last character in a string in PowerShell
You may also like:
- How To Split Comma Separated String To Array In PowerShell?
- How to Check if an Array Contains a String in PowerShell?
- Replace String Containing Special Characters in PowerShell
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com