Splitting a comma-separated string into an array in PowerShell is a common task that can be performed using the -split
operator or the .Split()
method. Here’s a step-by-step guide to learn how to split comma separated string to array in PowerShell.
To split a comma-separated string into an array in PowerShell, use the -split operator or the .Split() method. For instance, $array = $commaSeparatedString -split “,” will split the string at each comma. Alternatively, $array = $commaSeparatedString.Split(“,”) achieves the same result, creating an array where each element is a substring from the original string separated by commas.
PowerShell split comma-separated string to array
Now, let us check different methods to split a comma-separated string to an array in PowerShell.
Using the -split Operator
The -split
operator is used to split a string into substrings based on a specified delimiter in PowerShell.
Here is a full PowerShell script.
# Define a comma-separated string
$commaSeparatedString = "Chicago,New York,Dallas"
# Use the -split operator to split the string into an array
$array = $commaSeparatedString -split ","
# Display the array
$array
In this example, the string “Chicago,New York,Dallas” is split at each comma, resulting in an array containing “Chicago”, “New York”, and “Dallas”. You can check out the screenshot below, after I executed the PowerShell script using VS code.
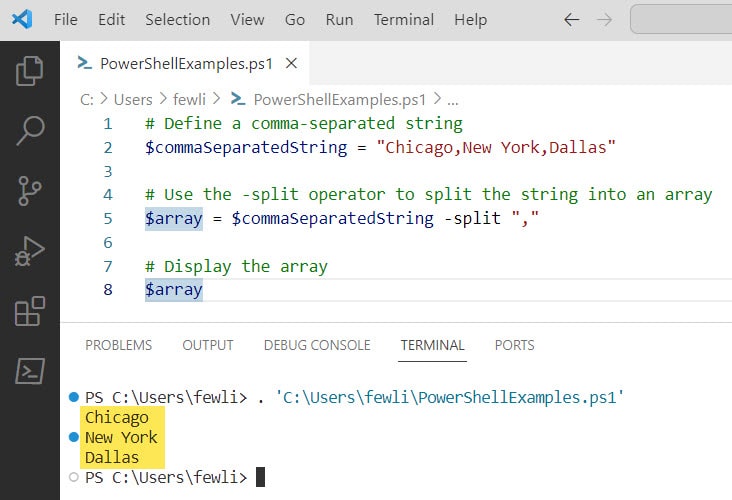
Using the .Split() Method
The .Split()
method is a string method that splits a string into an array of substrings based on the delimiter(s) you provide in PowerShell. Here is a complete example
# Define a comma-separated string
$commaSeparatedString = "Chicago,New York,Dallas"
# Use the .Split() method to split the string into an array
$array = @($commaSeparatedString.Split(","))
# Display the array
$array
Just like with the -split
operator, the .Split()
method in this example splits the string into an array where each city is an element in the array. You can see the screenshot below:
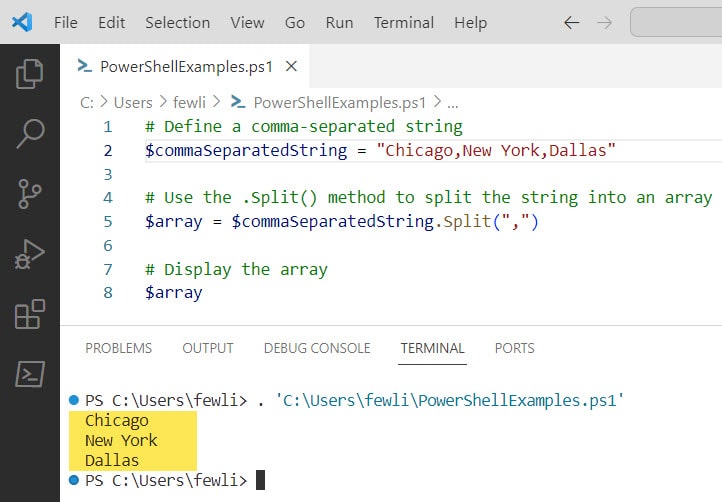
Handling Multiple Commas in PowerShell string
Sometimes, your string might contain multiple consecutive commas, and you might want to ignore empty entries that result from them. Here is an example:
# Define a string with multiple commas
$commaSeparatedString = "apple,,banana,,cherry"
# Use the -split operator with the option to remove empty entries
$array = $commaSeparatedString -split ",", 0, "RemoveEmptyEntries"
# Display the array
$array
In this example, the -split
operator is used with additional options to remove any empty entries that result from splitting at consecutive commas.
Advanced Splitting using regx
You can also use regular expressions with the -split
operator in PowerShell for more advanced splitting scenarios. Here is an example.
# Define a comma-separated string with different whitespace characters
$commaSeparatedString = "apple, banana, cherry, date"
# Use the -split operator with a regular expression to split the string into an array
# The regular expression here is a comma followed by zero or more whitespace characters
$array = $commaSeparatedString -split ",\s*"
# Display the array
$array
This example uses a regular expression ,\s*
to split the string, which means it looks for a comma followed by any number of whitespace characters.
Conclusion
These examples should give you a clear understanding of how to split a comma-separated string into an array in PowerShell. The -split
operator is versatile and can be used for simple and complex string splitting tasks. The .Split()
method is straightforward and is best for simple scenarios. Remember that PowerShell is case-insensitive by default, so -split
and .Split()
can be used interchangeably. However, it’s good practice to be consistent in your scripting style.
In this PowerShell tutorial, I have explained different methods to split a comma separated string into an array in PowerShell.
You may also like:
- PowerShell Replace String Before Character
- How to Check if an Array Contains a String in PowerShell?
- Replace Carriage Returns in Strings Using PowerShell
- Replace String Containing Special Characters in PowerShell
- PowerShell Substring() Example
- How to Split a String by Length in PowerShell?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com