The Substring() method is very helpful for working with PowerShell strings. In this PowerShell tutorial, I will explain how to use the Substring() method to extract parts of a string in PowerShell with a few real examples.
What is the Substring() Method in PowerShell
The Substring() method is a member of the String class in .NET. It allows you to extract a string portion based on a starting index and an optional length.
Method Overloads
The PowerShell Substring()
method has two overloads:
Substring(Int32 startIndex)
: Extracts a substring starting from the specified index to the end of the string.Substring(Int32 startIndex, Int32 length)
: Extracts a substring starting from the specified index with the specified length.
Syntax
Below is the syntax of the PowerShell substring() method.
$string.Substring(startIndex)
$string.Substring(startIndex, length)
Parameters
startIndex
: The zero-based starting position of the substring in the original string.length
: (Optional) The number of characters to include in the substring. If omitted, the substring extends from thestartIndex
to the end of the string.
Examples of Substring() in PowerShell
Now, let us check an example of a substring() in PowerShell. We will do all the operations using the below sting.
$names = "John, Emily, Michael, Sarah, David"
Extract from a Specific Index to the End
Suppose we want to extract all names starting from “Emily”. Below is the complete script.
# Original string
$names = "John, Emily, Michael, Sarah, David"
# Extract substring starting from index 6 to the end
$substring = $names.Substring(6)
Write-Output $substring
In this example, the substring starts at index 6 and includes all characters to the end of the string. Once you execute the above PowerShell script using VS code, it will give the below output:
Emily, Michael, Sarah, David
You can also see the output in the screenshot below:
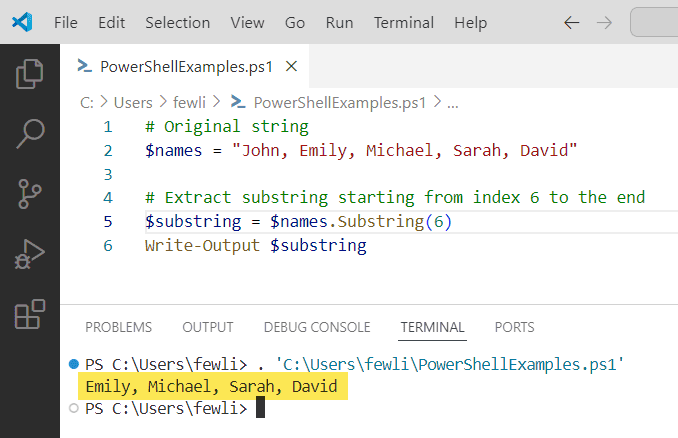
Extract a Substring with a Specific Length
Let us check out another example of how to extract a substring with a specific length.
Let’s extract just the name “Emily” from the above string.
Below is the complete PowerShell script.
# Original string with USA-based names
$names = "John, Emily, Michael, Sarah, David"
# Extract substring starting from index 6 with length 5
$substring = $names.Substring(6, 5)
Write-Output $substring
Here, the substring starts at index 6 and includes the next 5 characters.
You can see the output in the screenshot below after I expected the script:
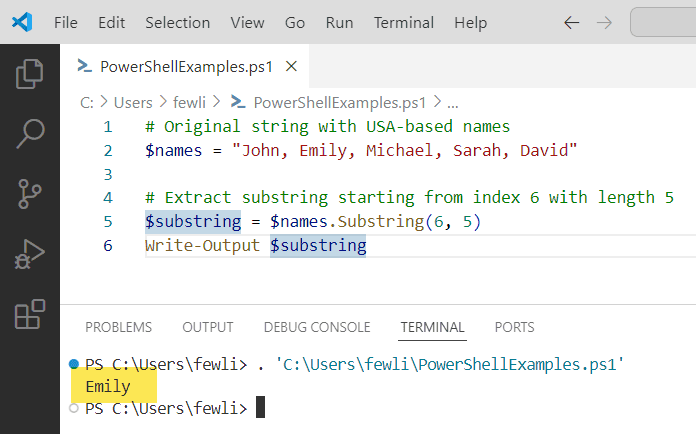
Extract the Last Name from the String
To extract the last name “David”, we need to calculate the starting index and use the Substring
method; below is the complete script.
# Original string with USA-based names
$names = "John, Emily, Michael, Sarah, David"
# Calculate the starting index for "David"
$startIndex = $names.LastIndexOf("David")
# Extract substring starting from the calculated index
$substring = $names.Substring($startIndex)
Write-Output $substring
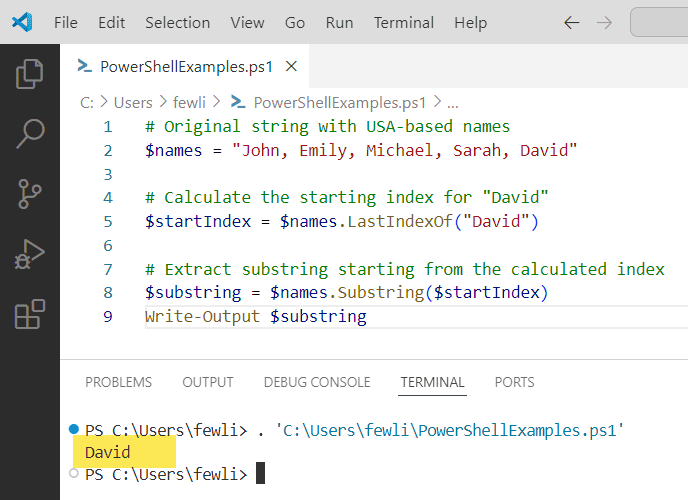
Extract a Specific Name by Index
If we want to extract “Michael”, we can specify the start index and length in PowerShell.
Below is the complete script.
# Original string with USA-based names
$names = "John, Emily, Michael, Sarah, David"
# Extract substring for "Michael"
$substring = $names.Substring(13, 7)
Write-Output $substring # Output: "Michael"
Error Handling While Using Substring() Method
It is always good to manage error handling while using the PowerShell Substring() method.
It’s important to ensure that the startIndex
and length
parameters are within the bounds of the string to avoid errors. PowerShell will throw an ArgumentOutOfRangeException
if the indices are invalid.
Check String Length
Before extracting a substring, you can check the length of the string to avoid errors. You can follow the below script.
# Original string with USA-based names
$names = "John, Emily, Michael, Sarah, David"
# Parameters for extracting a substring
$startIndex = 13
$length = 7
# Check if the startIndex and length are valid
if ($startIndex -lt $names.Length -and $startIndex + $length -le $names.Length) {
$substring = $names.Substring($startIndex, $length)
Write-Output $substring # Output: "Michael"
} else {
Write-Output "Invalid start index or length."
}
This script ensures that the startIndex
and length
are valid for the given string, preventing runtime errors.
Conclusion
The Substring() method in PowerShell extracts parts of a string. In this tutorial, I have explained how to use the PowerShell String() method with various examples and how to handle errors while using the substring() method in PowerShell.
You may like the following PowerShell tutorials:
- How to Convert String to Integer in PowerShell?
- Convert String to SecureString in PowerShell
- Convert Multiline String to Array in PowerShell
- Replace a String in Text File with PowerShell
- Convert String to DateTime in PowerShell
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com