Sometimes, you might need to convert string to date in PowerShell. This PowerShell tutorial will explore various methods to convert strings into DateTime objects in PowerShell with real examples.
To convert a string to a DateTime object in PowerShell, you can use the cast operator [datetime]
, the Parse
method, or the ParseExact
method for specific formats. For example, $dateTime = [datetime]"2024-01-20"
converts a standard date string into a DateTime object. For custom formats, you might use $dateTime = [datetime]::ParseExact("20-01-2024", "dd-MM-yyyy", $null)
. These methods provide a straightforward way to handle date and time conversions in your PowerShell scripts.
DateTime Object in PowerShell
In PowerShell, a DateTime object represents dates and times with a high degree of accuracy. It contains information about the year, month, day, hour, minute, second, and even fractions of a second, as well as the time zone.
Let us see various methods of converting string to datetime in PowerShell.
Method 1: Using the Cast Operator
The simplest way to convert a string to a DateTime object is by using the cast operator [datetime]
. This method attempts to interpret the string as a date and time in PowerShell.
# Example of using the cast operator
$dateString = "2024-01-20"
$dateTime = [datetime]$dateString
This example will convert the string “2024-01-20” to a DateTime object representing January 20, 2024.
After I executed the code using VS Code, you can see the output in the screenshot below:
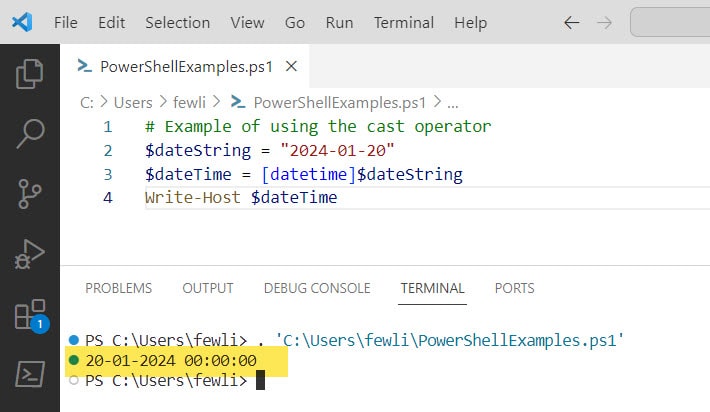
Method 2: Using the Parse Method
Another common method is to use the DateTime
class’s Parse
method in PowerShell to convert string to datetime. This is useful when your string is in a standard date and time format that PowerShell can recognize.
# Example of using the Parse method
$dateString = "January 20, 2024"
$dateTime = [datetime]::Parse($dateString)
This will create a DateTime object for the given date string.
Method 3: Using the ParseExact Method
You can use the parseExact PowerShell method when dealing with custom date and time formats. This method allows you to specify the exact format of your input string.
# Example of using ParseExact
$dateString = "20-01-2024 14:55"
$format = "dd-MM-yyyy HH:mm"
$cultureInfo = [System.Globalization.CultureInfo]::InvariantCulture
$dateTime = [datetime]::ParseExact($dateString, $format, $cultureInfo)
In this example, the date and time string “20-01-2024 14:55” is parsed according to the specified format “dd-MM-yyyy HH:mm”.
Method 4: Using the TryParse and TryParseExact Methods
For more robust error handling, you can use the TryParse
and TryParseExact
PowerShell methods. These methods return a boolean indicating whether the conversion was successful, and the result is returned in an output parameter.
# Example of using TryParse
$dateString = "2024-01-20"
[datetime]$dateTime = $null
$result = [datetime]::TryParse($dateString, [ref]$dateTime)
If $result
is $true
, the $dateTime
variable will contain the valid DateTime object.
Method 5: Using the Get-Date Cmdlet
PowerShell also provides a cmdlet named Get-Date
that can be used to convert a string to a DateTime object.
# Example of using Get-Date
$dateString = "01/20/2024"
$dateTime = Get-Date $dateString
This is a more PowerShell-centric way to perform the conversion and can be easier to read and write.
Formatting Dates in PowerShell
After converting a string to a DateTime object in PowerShell, you might want to format it. You can use the ToString
method to format your DateTime object into a string representation that suits your needs.
# Example of formatting a date
$dateTime = Get-Date
$formattedDate = $dateTime.ToString("yyyy-MM-dd")
This will format the current date into a string with the format “year-month-day” in PowerShell.
You can see the output in the screenshot below:
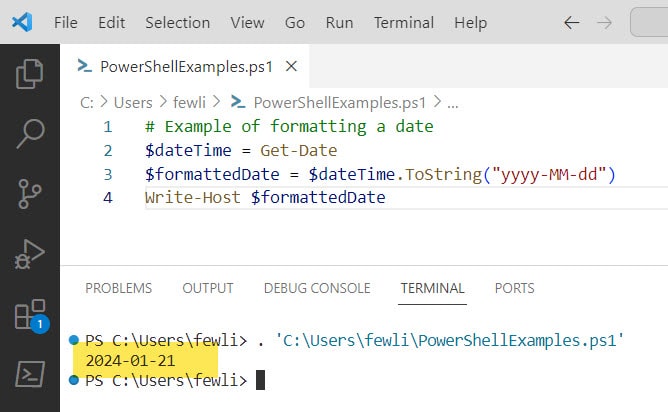
PowerShell convert string to datetime am/pm
In PowerShell, when you need to convert a string that includes an AM/PM designator to a DateTime object, you can use the ParseExact
or TryParseExact
methods of the DateTime class. These methods allow you to specify the exact format of your date and time string, including the AM/PM part.
Here’s an example using ParseExact
:
$dateString = "01-20-2024 02:30 PM"
$format = "MM-dd-yyyy hh:mm tt"
$cultureInfo = [System.Globalization.CultureInfo]::InvariantCulture
$dateTime = [datetime]::ParseExact($dateString, $format, $cultureInfo)
In this example, “MM-dd-yyyy hh tt” is the format string. MM
and dd
represent the two-digit month and day, yyyy
represents the four-digit year, hh
represents the two-digit hour in a 12-hour clock, mm
represents the two-digit minutes, and tt
represents the AM/PM designator.
Using ParseExact
, PowerShell interprets the string based on the provided format and creates a DateTime object accordingly. If the string does not match the format, an exception will be thrown. To avoid exceptions and handle errors more gracefully, you can use TryParseExact
which will return a boolean indicating the success or failure of the conversion.
Convert string to datetime milliseconds in PowerShell
In PowerShell, converting a string to a DateTime object with millisecond precision involves parsing the string with a format that includes milliseconds. The ParseExact
or TryParseExact
methods of the DateTime class can be used for this purpose, as they allow you to specify a custom format that includes milliseconds.
Here’s an example using ParseExact
:
$dateString = "2024-01-20 15:04:30.123"
$format = "yyyy-MM-dd HH:mm:ss.fff"
$cultureInfo = [System.Globalization.CultureInfo]::InvariantCulture
$dateTime = [datetime]::ParseExact($dateString, $format, $cultureInfo)
In the format string yyyy-MM-dd HH:mm:ss.fff
:
yyyy
represents the four-digit year.MM
represents the two-digit month.dd
represents the two-digit day.HH
represents the two-digit hour in a 24-hour clock.mm
represents the two-digit minutes.ss
represents the two-digit seconds.fff
represents the milliseconds.
This example will convert the string “2024-01-20 15:04:30.123” to a DateTime object, including the milliseconds. If the input string does not match the specified format, including the milliseconds, ParseExact
will throw an exception. To handle this scenario without exceptions, use TryParseExact
, which returns a boolean indicating whether the conversion was successful, and outputs the DateTime object if it was.
PowerShell convert string to datetime with timezone
Converting a string to a DateTime object with timezone information in PowerShell can be done using the ParseExact
method, which allows for specifying the format of the input string, including timezone designations. However, it’s important to note that the resulting DateTime object will not inherently store the timezone information; it will either convert the time to the local system’s timezone or to Coordinated Universal Time (UTC).
Here’s an example of converting a string including timezone information to a DateTime object:
$dateString = "2024-01-20T15:04:30-05:00" # Date string with timezone offset
$format = "yyyy-MM-ddTHH:mm:ssK" # Format string with 'K' for timezone designator
$cultureInfo = [System.Globalization.CultureInfo]::InvariantCulture
$dateTimeWithOffset = [datetimeoffset]::ParseExact($dateString, $format, $cultureInfo)
In the format string yyyy-MM-ddTHH:mm:ssK
:
yyyy
represents the four-digit year.MM
represents the two-digit month.dd
represents the two-digit day.THH:mm:ss
represents the ‘T’ character followed by two-digit hour, minute, and second, respectively.K
represents the timezone information.
In this example, the timezone information is indicated by the -05:00
at the end of the string, which means that the time is offset by 5 hours from UTC. The ParseExact
method parses the string and applies the offset to the resulting DateTimeOffset
object. The DateTimeOffset
type is used instead of DateTime
because it can represent points in time relative to UTC, including the offset.
If you need to convert the time to another timezone, you can use the TimeZoneInfo
class along with methods like ConvertTimeBySystemTimeZoneId
to translate the DateTimeOffset
to the desired timezone.
Conclusion
Converting strings to DateTime objects in PowerShell can be achieved through various methods. In this PowerShell tutorial, I have explained the below 5 methods to convert string to datetime in PowerShell.
- Using the Cast Operator
- Using the Parse Method
- Using the ParseExact Method
- Using the TryParse and TryParseExact Methods
- Using the Get-Date Cmdlet
Also, I have explained how to convert string to datetime with timezone in PowerShell and examples on PowerShell convert string to datetime am/pm and PowerShell convert string to datetime milliseconds.
You may also like:
- PowerShell Get-date Format Milliseconds
- How to Get-Date Without Time in PowerShell?
- PowerShell If Date Is Older Than 30 Days
- How to Convert String to SecureString in PowerShell
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com