Do you need to convert multiline strings into arrays in PowerShell? In this PowerShell tutorial, I will explain to you different methods to convert a multiline string into an array in PowerShell.
To convert a multiline string into an array in PowerShell, you can use the -split
operator with a newline character as the delimiter. For example:
$multiLineString = "Line one`nLine two`nLine three"
$array = $multiLineString -split "`n"
This code splits the string at each newline (`n) into an array of lines, with each line becoming an element in the array.
PowerShell convert multiline string to array
There are different methods you can use to convert a multiline string to an array in PowerShell. We will check one-by-one methods to convert multiline strings to arrays in PowerShell with real examples.
Method 1: Using the -split Operator
The simplest way to convert a multiline string into an array is by using the -split
operator in PowerShell. This operator splits a string into substrings based on a delimiter, which by default is any whitespace character, including a newline.
Here’s an example:
$multiLineString = @"
Line one
Line two
Line three
"@
$array = $multiLineString -split "`r`n"
foreach ($line in $array) {
Write-Host $line
}
In this example, we’re using the carriage return and newline characters (r
n) as the delimiter to split the string into lines. The foreach
loop then iterates over each line in the array and outputs it to the host.
You can see the output in the screenshot below after I executed the code using VS code.
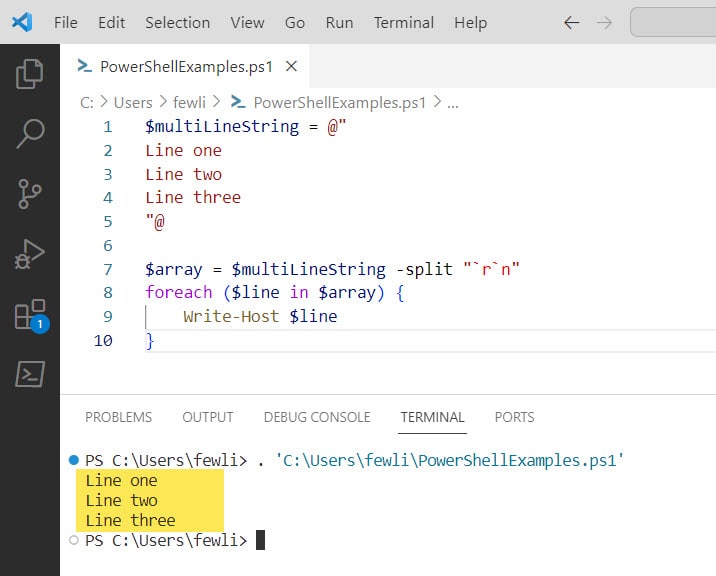
Method 2: Using the .Split() Method
Another way to convert a multiline string into an array in PowerShell is by using the .Split()
method of a string object. This method allows you to specify the delimiter as an argument.
Here’s how you can use it:
$multiLineString = @"
Line one
Line two
Line three
"@
$array = $multiLineString.Split("`r`n", [StringSplitOptions]::RemoveEmptyEntries)
foreach ($line in $array) {
Write-Host $line
}
The [StringSplitOptions]::RemoveEmptyEntries
option ensures that any empty entries are not included in the resulting array. Here is the screenshot below after I executed the code using VS code:
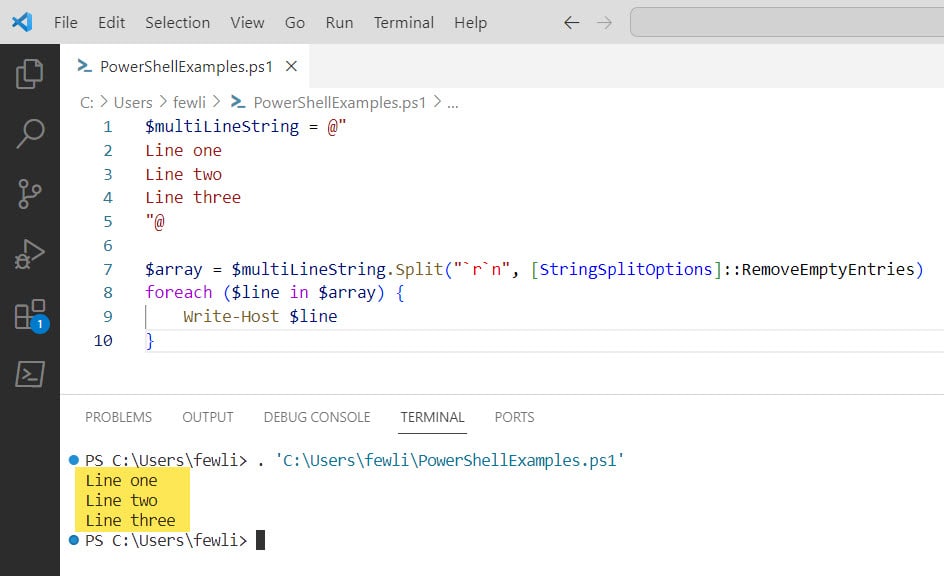
Method 3: Using the ConvertFrom-String Cmdlet
For more complex scenarios, you might want to use the ConvertFrom-String
cmdlet in PowerShell. This cmdlet is designed to convert structured text into objects. While it’s a bit more advanced, it can be handy when you need to parse a multiline string into a structured array.
Here’s a basic example:
$data = @"
Name: John
Age: 30
Occupation: Engineer
Name: Jane
Age: 25
Occupation: Designer
"@
$template = @"
{Name*:John} {Age*:30} {Occupation*:Engineer}
"@
$array = $data | ConvertFrom-String -TemplateContent $template
$array | Format-Table -AutoSize
In this example, we define a template that describes the structure of the data, and ConvertFrom-String
uses this template to parse the multiline string into an array of objects.
Method 4: Using the Out-String Cmdlet
The Out-String
cmdlet is typically used to convert objects into strings. However, you can combine it with other cmdlets to convert a multiline string into an array.
Here’s an example:
$multiLineString = @"
Line one
Line two
Line three
"@
$array = $multiLineString | Out-String -Stream
$array | ForEach-Object { Write-Host $_ }
The -Stream
parameter of Out-String
causes it to return an array of lines instead of a single string, which we can then iterate over.
Conclusion
Converting multiline strings into arrays is a common task in PowerShell that can be achieved through several methods, depending on the complexity of your data and your specific needs. The -split
operator and .Split()
methods are straightforward and effective for simple string splitting, while ConvertFrom-String
and Out-String
can be used for more complex or structured data.
I hope this PowerShell tutorial explains how to convert multiline strings to arrays in PowerShell using various methods.
You may also like:
- How to Loop Through an Array of Objects in PowerShell?
- How to Convert String to DateTime in PowerShell?
- How to Replace String in XML File using PowerShell?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com