In this tutorial, I will explain how to set current date in datepicker using javascript or jQuery in SharePoint Online or SharePoint On-premises versions.
Here, in this example, I was creating an HTML input form for the SharePoint Online site. For that, I was using a date picker control. My requirement was to bind the current date to the date picker using jQuery.
The same jQuery code we can use to add a date picker control and set the default as of today’s date in SharePoint. The code you can add inside a script editor or content editor web part in SharePoint.
You can also learn how to create an HTML form in classic SharePoint sites; in modern SharePoint Online sites, you can not add any script editor web part.
HTML DatePicker jQuery
First, create a “DatePicker” by using the below HTML code:
<input type="date" id="SubmitDate" title="Submitdate"/>
In this HTML Code, I have given a date ID attribute as “SubmitDate”.
You can also use the jQuery date picker in SharePoint.
Set Current Date in DatePicker using jQuery
Now, we have to bind this date picker to the current date or today’s date using JQuery. The JQuery code is given below-
var now = new Date();
var day = ("0" + now.getDate()).slice(-2);
var month = ("0" + (now.getMonth() + 1)).slice(-2);
var today = now.getFullYear() + "-" + (month) + "-" + (day);
$('#SubmitDate').val(today);
Here in this JQuery Code, I have taken a Variable name as “now” and assigned the current date to the date picker. For that “now” variable, I have passed in the variable “day”, “month,” and “today” using Query String as “now.getDate()”, “now.getMonth()” and “now.getFullYear()”. So that we can get the date as a UTC Format like (YY-MM-DD).
To set Today’s date, write like below.
$('#SubmitDate').val(today);
The full code to bind the current date or today’s date to a date picker using jQuery in SharePoint looks like this below:
Select Date: <input type="date" id="SubmtDate" title="Select a date"/>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.4.2/jquery.min.js"></script>
<script language="javascript" type="text/javascript">
$(document).ready(function() {
setCurrentDate()
});
function setCurrentDate()
{
var now = new Date();
var day = ("0" + now.getDate()).slice(-2);
var month = ("0" + (now.getMonth() + 1)).slice(-2);
var today = now.getFullYear() + "-" + (month) + "-" + (day);
$('#SubmitDate').val(today);
}
</script>
Once you run the code, you can see the output looks like below in the SharePoint web part page.
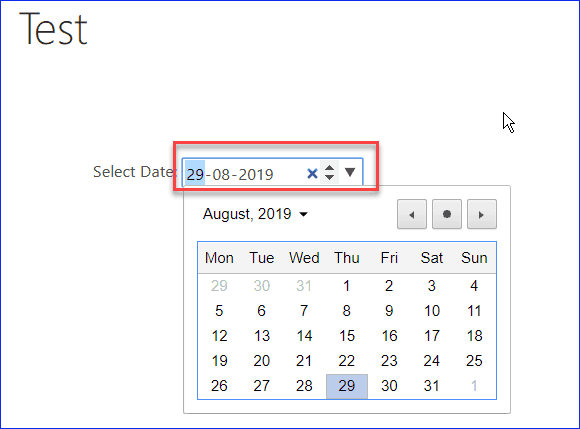
In this SharePoint tutorial, we discussed how to bind the current date or today’s date using JQuery in SharePoint.
How to set current date in datepicker using JavaScript
To set the current date in a datepicker using JavaScript, you can use the following example code:
// Assuming you have a datepicker input field with the id 'datepicker'
document.addEventListener('DOMContentLoaded', function() {
var today = new Date().toISOString().substr(0, 10); // Get current date in YYYY-MM-DD format
document.querySelector("#datepicker").value = today; // Set the current date as the value of the datepicker
});
Here is the complete code:
document.addEventListener('DOMContentLoaded', function() {...});
– This ensures that the JavaScript code runs only after the HTML document has been fully loaded.var today = new Date().toISOString().substr(0, 10);
– This line creates a newDate
object for the current date and time. ThetoISOString()
method converts the date to a string in the ISO 8601 format (YYYY-MM-DDTHH:mm:ss.sssZ). Thesubstr(0, 10)
method then extracts the first 10 characters of the ISO string, which represent the date portion (YYYY-MM-DD).document.querySelector("#datepicker").value = today;
– This line selects the HTML element with theid
ofdatepicker
and sets itsvalue
property to thetoday
variable, which is the current date in the required format.
By including this JavaScript code in your HTML file, the datepicker field will automatically display the current date when the page is loaded.
For a more detailed example, you might include the HTML for the datepicker input:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Datepicker Example</title>
</head>
<body>
<!-- Datepicker input field -->
<input type="date" id="datepicker">
<!-- JavaScript to set the current date in the datepicker -->
<script>
document.addEventListener('DOMContentLoaded', function() {
var today = new Date().toISOString().substr(0, 10);
document.querySelector("#datepicker").value = today;
});
</script>
</body>
</html>
In this complete example, when you load the HTML page in a browser, you will see an input field of type date
that will have the current date pre-filled.
Set current date in datepicker using JavaScript
Setting the current date in a datepicker using JavaScript can be easily done by manipulating the value of the date input field. Here’s a simple example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Set Current Date in Datepicker</title>
</head>
<body>
<input type="date" id="datepicker">
<script>
// Create a new Date object for the current date
var today = new Date();
// Format the date to YYYY-MM-DD
var dateStr = today.getFullYear() + '-' + ('0' + (today.getMonth() + 1)).slice(-2) + '-' + ('0' + today.getDate()).slice(-2);
// Set the formatted date string as the value of the datepicker input
document.getElementById('datepicker').value = dateStr;
</script>
</body>
</html>
In this example:
- An
<input>
element of the typedate
with the IDdatepicker
is defined in the HTML. - A
<script>
tag contains JavaScript that will set the input’s value to the current date. - A new
Date
object is created to get the current date. - The date is formatted to a string in the
YYYY-MM-DD
format. This is necessary because the value of an<input type="date">
must be in this format. The month and day are padded with zeros to ensure they are always two digits, using('0' + (today.getMonth() + 1)).slice(-2)
for the month and('0' + today.getDate()).slice(-2)
for the day. - Finally,
document.getElementById('datepicker').value = dateStr;
sets the value of the datepicker input to the formatted date string, which represents the current date.
When you load this HTML in a browser, the datepicker will display the current date by default.
Set today date in datepicker using jQuery
To set the current date in a datepicker using jQuery, you would use the jQuery datepicker
method along with the setDate
property. Below is an example of how to set today’s date in Datepicker using jQuery.
First, ensure you include jQuery and jQuery UI in your HTML file because the datepicker function is part of jQuery UI, not the core jQuery library:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>jQuery Datepicker Example</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/base/jquery-ui.css">
<script src="//code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.min.js"></script>
</head>
<body>
<!-- Datepicker input field -->
<input type="text" id="datepicker">
<!-- JavaScript to set the current date in the datepicker using jQuery -->
<script>
$(document).ready(function() {
// Initialize the datepicker
$("#datepicker").datepicker();
// Set the date to today's date
$("#datepicker").datepicker("setDate", new Date());
});
</script>
</body>
</html>
Here’s a breakdown of the code:
- Include jQuery and jQuery UI libraries in the head section of your HTML. These are necessary to use the datepicker functionality.
<input type="text" id="datepicker">
– This line creates an input field where the jQuery UI datepicker will be attached.- The script block contains jQuery code that initializes the datepicker and sets its date.
$(document).ready(function() {...});
– This is a jQuery function that makes sure the code inside it only runs once the DOM is fully loaded.$("#datepicker").datepicker();
– This line initializes the datepicker on the input field with the IDdatepicker
.$("#datepicker").datepicker("setDate", new Date());
– This line sets the date of the datepicker to the current date. Thenew Date()
JavaScript function creates a new Date object representing the current date and time.
With this code, when the page loads, the input field will display a datepicker when clicked, and the datepicker will show the current date as selected.
You may also like the following tutorials:
- SharePoint Calendar Color Code
- How to Use SharePoint Group Calendar Web Part?
- Create Calendar Events from a SharePoint list using Power Automate
- SharePoint Interview Questions And Answers
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com