Recently, I was working with an array of objects in PowerShell where I was in need to loop through an array of objects in PowerShell. In this blog post, we’ll explore how to loop through an array of objects in PowerShell using various methods.
To loop through an array of objects in PowerShell, you can use the foreach
loop, which allows you to iterate over each object and access its properties directly. Here’s a quick example:
foreach ($obj in $arrayOfObjects) {
# Perform actions with $obj's properties
"Name: $($obj.Name), Value: $($obj.Value)"
}
This loop will go through each object in $arrayOfObjects
, and you can perform any actions or access any properties within the loop body.
Arrays of Objects in PowerShell
Before checking into the looping methods, let’s first understand what an array of objects is. In PowerShell, an array is a data structure that can hold a collection of items. When we talk about an array of objects, we mean an array where each item is an object with properties and methods.
For example, consider an array of custom objects representing employees:
$employees = @(
@{Name="John Doe"; Department="IT"; Salary=60000},
@{Name="Jane Smith"; Department="HR"; Salary=65000},
@{Name="Jim Brown"; Department="Finance"; Salary=70000}
)
# Convert each hashtable to a PSCustomObject
$employees = $employees | ForEach-Object { [PSCustomObject]$_ }
In this array, each employee is represented by a PSCustomObject
with properties Name
, Department
, and Salary
.
Loop Through an Array of Objects in PowerShell
1. Using the foreach Loop
The foreach
loop is one of the simplest and most common ways to iterate over an array of objects in PowerShell. The syntax is straightforward:
$employees = @(
@{Name="John Doe"; Department="IT"; Salary=60000},
@{Name="Jane Smith"; Department="HR"; Salary=65000},
@{Name="Jim Brown"; Department="Finance"; Salary=70000}
)
foreach ($employee in $employees) {
# Access the properties of each object
"Name: $($employee.Name), Department: $($employee.Department), Salary: $($employee.Salary)"
}
In this loop, $employee
represents the current object in the array as the loop iterates through each item.
After I executed the code using VS code, you can see the output in the screenshot below. You can also use Windows PowerShell ISE to execute the code.
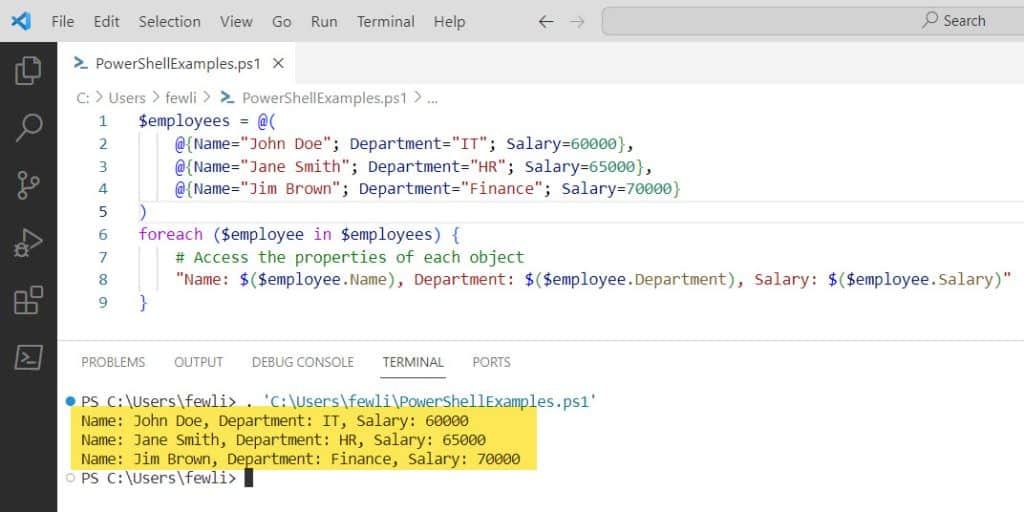
2. Using the For Loop
The for loop provides more control over the iteration process and is useful when you need to access the array by index. Here is the complete PowerShell script to loop through an array of objects.
$employees = @(
@{Name="John Doe"; Department="IT"; Salary=60000},
@{Name="Jane Smith"; Department="HR"; Salary=65000},
@{Name="Jim Brown"; Department="Finance"; Salary=70000}
)
for ($i = 0; $i -lt $employees.Length; $i++) {
$employee = $employees[$i]
"Name: $($employee.Name), Department: $($employee.Department), Salary: $($employee.Salary)"
}
Here, $i
is the index variable that starts at 0 and increments until it reaches the end of the array.
3. Using the ForEach-Object Cmdlet
The ForEach-Object
cmdlet is a pipeline-based method that allows you to perform an action on each object in the array. Here is the PowerShell script to loop through an array of objects in PowerShell using the ForEach-Object Cmdlet.
$employees = @(
@{Name="John Doe"; Department="IT"; Salary=60000},
@{Name="Jane Smith"; Department="HR"; Salary=65000},
@{Name="Jim Brown"; Department="Finance"; Salary=70000}
)
$employees | ForEach-Object {
"Name: $($_.Name), Department: $($_.Department), Salary: $($_.Salary)"
}
In this example, $_
represents the current object being passed through the pipeline.
You can see the output in the screenshot below after I executed the above PowerShell script.
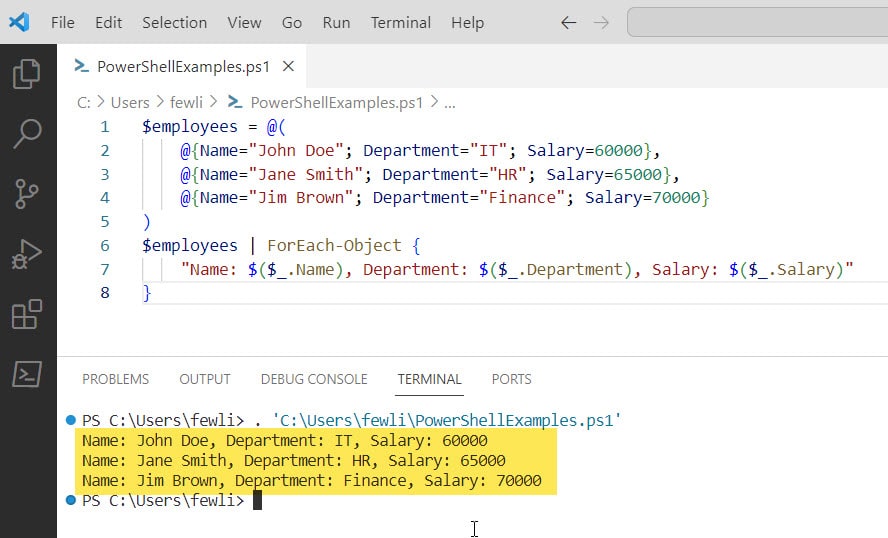
Sometimes, you may want to loop through a subset of the array of objects that meets certain criteria. You can use the Where-Object
cmdlet to filter the array of objects:
$employees | Where-Object { $_.Department -eq 'IT' } | ForEach-Object {
"Name: $($_.Name), Department: $($_.Department), Salary: $($_.Salary)"
}
This will only loop through employees in the IT department.
4. Using the while and do-while Loops
The while
and do-while
loops are not commonly used for iterating over arrays of objects in PowerShell but can be useful in certain scenarios:
$employees = @(
@{Name="John Doe"; Department="IT"; Salary=60000},
@{Name="Jane Smith"; Department="HR"; Salary=65000},
@{Name="Jim Brown"; Department="Finance"; Salary=70000}
)
$i = 0
while ($i -lt $employees.Length) {
$employee = $employees[$i]
"Name: $($employee.Name), Department: $($employee.Department), Salary: $($employee.Salary)"
$i++
}
# do-while example
$i = 0
do {
$employee = $employees[$i]
"Name: $($employee.Name), Department: $($employee.Department), Salary: $($employee.Salary)"
$i++
} while ($i -lt $employees.Length)
These loops continue to execute as long as the condition $i -lt $employees.Length
is true.
Conclusion
Looping through an array of objects in PowerShell is an essential requirement. PowerShell provides different methods to loop through an array of objects in PowerShell using a foreach
loop, for
loop, ForEach-Object
cmdlet, etc.
In this PowerShell tutorial, I have explained, how to loop through an array of objects in PowerShell using various methods:
- Using the foreach Loop
- Using the For Loop
- Using the ForEach-Object Cmdlet
- Using the while and do-while Loops
You may also like:
- How To Compare Array Of Objects In PowerShell?
- How to Convert Multiline String to Array in PowerShell?
- How To Sort Array Of Objects In PowerShell?
- How to Count the Number of Objects in an Array in PowerShell?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com