In this PowerShell tutorial, I will explain to you how to count the number of objects in an array in PowerShell using different methods and real examples.
To count the number of objects in an array in PowerShell, you can utilize either the .Count
or .Length
property of the array. Here’s a simple example:
$myArray = @(1, 2, 3, 4, 5)
$objectCount = $myArray.Count
This will store the number of elements in $myArray
, which is 5
, into the variable $objectCount
. Both properties will yield the same result, providing a straightforward way to determine the size of an array.
Count the Number of Objects in an Array in PowerShell
To count the number of objects in an array in PowerShell, you can use the Count
property or the Length
property. These properties are available on all arrays and return the total number of elements in the array.
Here’s how you can use the Count
property:
# Define a custom object
$customObject1 = New-Object PSObject -Property @{
Name = "Object1"
Value = 10
}
$customObject2 = New-Object PSObject -Property @{
Name = "Object2"
Value = 20
}
# Create an array of custom objects
$myObjectArray = @($customObject1, $customObject2)
# Use the Count property to get the number of objects in the array
$numberOfObjects = $myObjectArray.Count
Write-Output "The array contains $numberOfObjects objects."
This script creates two custom objects and then places them into an array. The Count
property is then used to determine that the array contains 2 objects. The output will be “The array contains 2 objects.”
And here’s the Length
property, which achieves the same result:
# Define a custom object
$customObject1 = New-Object PSObject -Property @{
Name = "Object1"
Value = 10
}
$customObject2 = New-Object PSObject -Property @{
Name = "Object2"
Value = 20
}
# Create an array of custom objects
$myObjectArray = @($customObject1, $customObject2)
# Use the Length property to get the number of objects in the array
$numberOfObjects = $myObjectArray.Length
Write-Output "The array contains $numberOfObjects objects."
Just like the Count
property, the Length
property will return the number of elements in $myObjectArray
, which is 2
. The output will be “The array contains 2 objects.” Both properties are interchangeable for arrays in PowerShell.
You can see the output in the below screenshot.
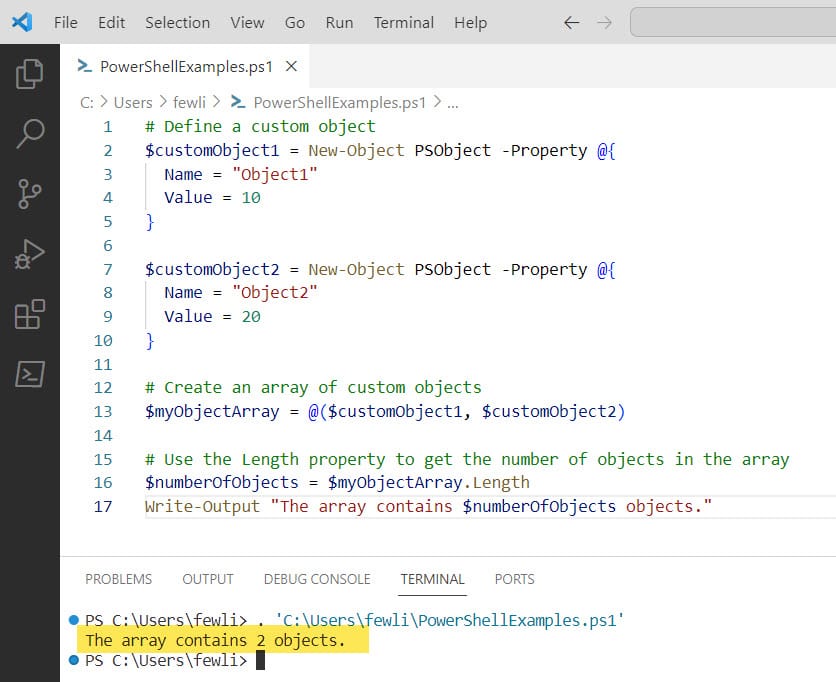
Count Single Objects and Empty Arrays in PowerShell
When you’re working with a variable that you expect to be an array, it’s important to handle cases where the variable might not actually contain an array. For example, if the command that you expect to return an array returns a single object or $null
, the Count
property won’t be present, and trying to access it will result in an error.
To ensure that you always have an array, you can use the array subexpression operator @()
. This operator ensures that whatever you’re working with is treated as an array, even if it’s a single object or $null
.
Here’s an example:
# This might return a single object, $null, or an array
$result = Get-SomeData
# Ensure $result is treated as an array
$arrayResult = @($result)
# Now it's safe to use the Count property
$numberOfItems = $arrayResult.Count
Write-Output "The array contains $numberOfItems items."
Count Objects in Arrays of Arrays in PowerShell
If you have an array of arrays, counting the objects can be a bit more complex, depending on whether you want the total count of all items in all subarrays or the count of the subarrays themselves.
To count the total number of items in all subarrays, you can use the Measure-Object
cmdlet with the -Sum
parameter:
$multiArray = @(@('Apple', 'Banana'), @('Cherry', 'Date'), @('Elderberry'))
$totalCount = ($multiArray | Measure-Object -Property Count -Sum).Sum
Write-Output "The total number of items in all subarrays is $totalCount."
This will output the total count of all items across all subarrays.
Count Objects with Custom Properties in PowerShell
If you have an array of custom objects, you might want to count the number of objects based on a specific property. For example, if you have an array of process objects, you might want to count how many are using more than a certain amount of memory.
Here’s how you can do that:
# Get a list of processes and convert it to an array
$processes = Get-Process
$processArray = @($processes)
# Count the number of processes using more than 100MB of memory
$highMemoryCount = ($processArray | Where-Object { $_.WS -gt 100MB }).Count
Write-Output "There are $highMemoryCount processes using more than 100MB of memory."
In this example, $_
represents each object in the array, and WS
is a property that holds the working set size (memory usage) of the process. The Where-Object
cmdlet filters the array, and then we count the filtered results.
Conclusion
In PowerShell, you can always use the Count
or Length
properties to get the number of items in an array. Use the array subexpression operator @()
to ensure you’re always working with an array, and use Measure-Object
and Where-Object
to count items in more complex scenarios.
In this PowerShell tutorial, I have explained how to Count the Number of Objects in an Array in PowerShell.
You may also like:
- How To Compare Array Of Objects In PowerShell?
- How to Remove Duplicate Objects from an Array in PowerShell?
- How to Remove the First and Last Item in an Array in PowerShell?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com