Recently, while working on an array of objects in PowerShell, I got a requirement to format it as a table. In this PowerShell tutorial, I will explain how to use the Format-Table PowerShell cmdlet to format an array of objects as a table in PowerShell.
To format an array of objects as a table in PowerShell, use the Format-Table cmdlet. This cmdlet takes your array and outputs it in a table format, with each object’s properties displayed as columns. You can customize the table with parameters like -Property to specify which columns to display, and -AutoSize to adjust column widths based on the content. Here’s a simple example: $myArray | Format-Table -Property Name, Age, Job -AutoSize.
Format An Array Of Objects As Table In PowerShell Using Format-Table
Here is an example of a PowerShell array of objects.
$myArray = @(
[PSCustomObject]@{Name='Alice'; Age=25; Job='Engineer'},
[PSCustomObject]@{Name='Bob'; Age=30; Job='Designer'},
[PSCustomObject]@{Name='Charlie'; Age=35; Job='Manager'}
)
Now, let us see how to format this array of objects as a table in PowerShell Using Format-Table cmdlet.
The PowerShell Format-Table
cmdlet is a powerful tool that formats the output of a command as a table with the selected properties of the object in each column. Here’s a simple example of how to use Format-Table
:
# Create an array of objects
$myArray = @(
[PSCustomObject]@{Name='Alice'; Age=25; Job='Engineer'},
[PSCustomObject]@{Name='Bob'; Age=30; Job='Designer'},
[PSCustomObject]@{Name='Charlie'; Age=35; Job='Manager'}
)
# Format the array as a table
$myArray | Format-Table
This would output:
Name Age Job
---- --- ---
Alice 25 Engineer
Bob 30 Designer
Charlie 35 Manager
You can also see the screenshot below after I executed the PowerShell script using VS code.
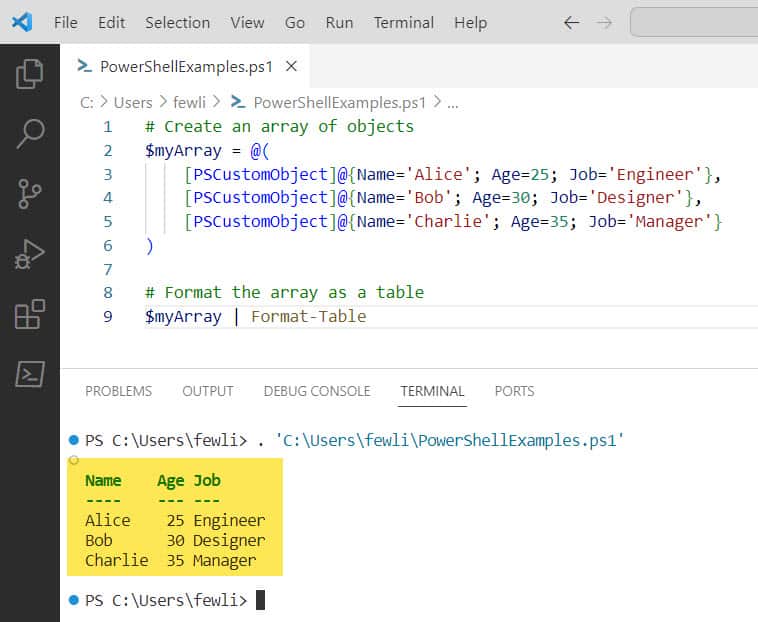
The Format-Table
cmdlet automatically selects the properties to display as columns. However, you can also specify which properties to display using the -Property
parameter:
# Format the array as a table with selected properties
$myArray | Format-Table -Property Name, Job
This would output:
Name Job
---- ---
Alice Engineer
Bob Designer
Charlie Manager
Customizing the Table Display
You can customize how Format-Table
displays the data in various ways. For example, you can control the width of the columns with the -AutoSize
parameter, which adjusts the column sizes based on the width of the data:
# Create an array of objects
$myArray = @(
[PSCustomObject]@{Name='Alice'; Age=25; Job='Engineer'},
[PSCustomObject]@{Name='Bob'; Age=30; Job='Designer'},
[PSCustomObject]@{Name='Charlie'; Age=35; Job='Manager'}
)
# Format the array as a table and auto-size the columns
$myArray | Format-Table -AutoSize
You can also create calculated properties to display in the table using a script block. Here’s an example where we add a new property that calculates the year of birth:
# Create an array of objects
$myArray = @(
[PSCustomObject]@{Name='Alice'; Age=25; Job='Engineer'},
[PSCustomObject]@{Name='Bob'; Age=30; Job='Designer'},
[PSCustomObject]@{Name='Charlie'; Age=35; Job='Manager'}
)
# Add a calculated property for YearOfBirth
$myArray | Format-Table Name, @{Name='YearOfBirth'; Expression={2024 - $_.Age}}
Once you execute the above code, you can see the output in the screenshot below.
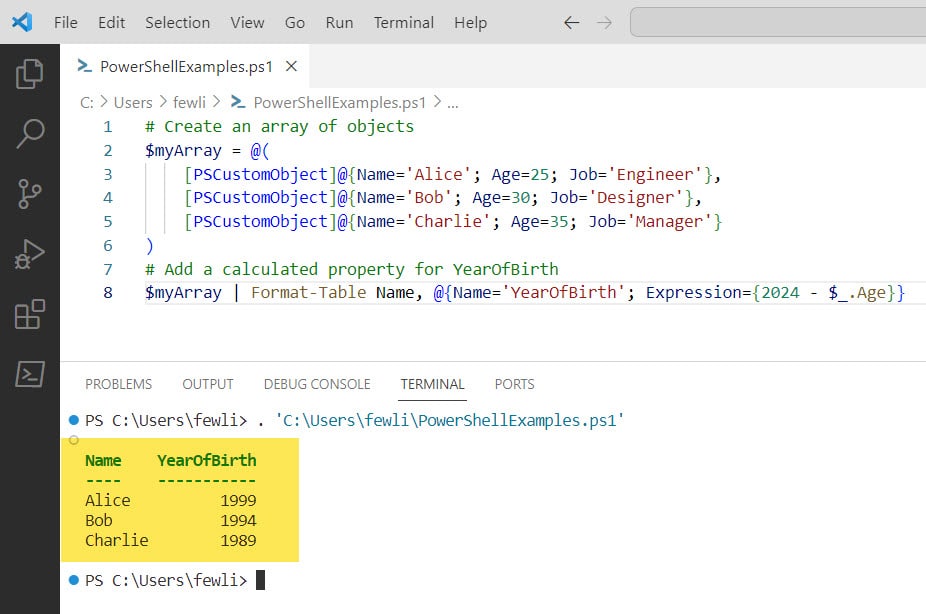
Advanced Formatting with Hashtables
Sometimes, you may have an array of hashtables instead of objects. You can still use Format-Table
to display this data in a table format. Here’s an example:
# Create an array of hashtables
$myHashtableArray = @(
@{Name='Alice'; Age=25; Job='Engineer'},
@{Name='Bob'; Age=30; Job='Designer'},
@{Name='Charlie'; Age=35; Job='Manager'}
)
# Convert each hashtable to a PSCustomObject and format as a table
$myHashtableArray | ForEach-Object { [PSCustomObject]$_ } | Format-Table
This would output the same table as before, with each hashtable being converted to an object before formatting.
Exporting Tables to Files
Once you have your data formatted as a table, you might want to export it to a file for further analysis or reporting. PowerShell makes this easy with cmdlets like Export-Csv
:
# Create an array of objects
$myArray = @(
[PSCustomObject]@{Name='Alice'; Age=25; Job='Engineer'},
[PSCustomObject]@{Name='Bob'; Age=30; Job='Designer'},
[PSCustomObject]@{Name='Charlie'; Age=35; Job='Manager'}
)
# Export the table to a CSV file
$myArray | Format-Table | Export-Csv -Path 'myData.csv' -NoTypeInformation
Conclusion
Formatting an array of objects as a table in PowerShell is very easy with the Format-Table cmdlet. It provides a quick way to view and analyze structured data clearly and organized. Using parameters and calculated properties, you can customize the table to display exactly what you need. Additionally, you can easily export your tables to files for further use.
In this PowerShell tutorial, I have explained how to format an array of objects as a table in PowerShell using the Format-Table PowerShell command.
You may also like:
- How to Pass Objects to Functions in PowerShell?
- How to Remove Empty Lines from an Array in PowerShell?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com