While working with a Typescript application, I got a requirement to convert string to number in Typescript. In this Typescript tutorial, I will explain how to convert a string to a number in Typescript using various methods.
To convert a string to a number in Typescript, you can use the unary plus (+) operator, the Number() constructor, or the parseInt() and parseFloat() functions depending on whether you’re expecting an integer or a floating-point number. The unary plus is the most concise method, as it directly converts a string like “123” to the number 123.
Different Methods to Convert String to Number in TypeScript
In TypeScript, converting strings to numbers is a common task when one desires to perform numerical operations on values originally formatted as text. The language provides several methods, each suitable for different scenarios.
Unary Plus (+
): A quick and shorthand way to convert a string to a number, the unary plus operator converts a string containing a decimal integer, like “123”, into an integer. Here is an example:
let value: string = "123";
let numberValue: number = +value; // becomes 123
This method also works for floating-point numbers, but it does not parse strings with extra characters or return special values for boolean inputs.
parseInt()
: Dedicated to parsing integer values, parseInt()
converts a string to an integer, ignoring any characters after a non-digit one:
let floatString: string = "123.45";
let intValue: number = parseInt(floatString); // becomes 123
// parseInt ignores the '.45'
You can see the output in the screenshot below.
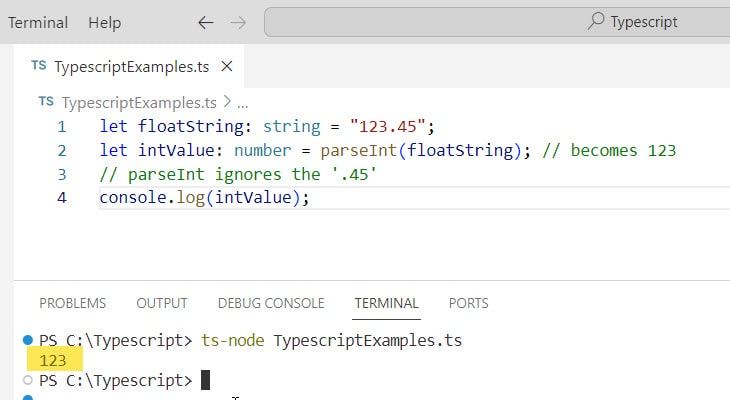
parseFloat()
: This function parses floating-point numbers:
let floatString: string = "123.45";
let floatValue: number = parseFloat(floatString); // becomes 123.45
Number()
: The Number
constructor can be used to convert strings to number values, including integers and floating-point numbers:
let numericString: string = "123.45";
let numberValue: number = Number(numericString); // becomes 123.45
The Number()
constructor returns NaN
for strings that do not represent valid numbers.
In applications where precise numerical conversion is essential, it is crucial to use the method best suited to the data format and the desired number type — whether an integer or a floating-point number. These built-in methods offer robust and reliable ways to convert strings to numbers in TypeScript.
Convert String with Comma to Number in TypeScript
When working with numerical strings that include commas, such as “1,000” or “10,000.50”, one must first remove the commas before converting the string to a number. TypeScript, being a superset of JavaScript, follows the same procedures as JavaScript for string to number conversion.
Step 1: Remove Commas Before the conversion, all comma characters need to be eliminated from the string. This can be done using the replace
method with a regular expression:
let stringWithCommas = "1,234,567.89";
let stringWithoutCommas = stringWithCommas.replace(/,/g, '');
The replace
method here uses the global flag (g
) to replace all occurrences of the comma in the string.
Step 2: Convert to Number Once the commas are removed, the string can be converted to a number using either the Number
constructor, parseFloat
, or the unary +
operator:
- Using
Number
:
let numberValue = Number(stringWithoutCommas);
- Using
parseFloat
:
let numberValue = parseFloat(stringWithoutCommas);
- Using Unary
+
:
let numberValue = +stringWithoutCommas;
Type Considerations It’s important to note that TypeScript is strongly typed. When parsing strings to numbers, one should ensure that the resulting variable is typed as a number to avoid type errors:
let numericValue: number = +stringWithoutCommas;
This method is straightforward and effective for converting a string containing commas to a number in TypeScript.
TypeScript Convert String to Number with 2 Decimal Places
When working with TypeScript, one may often need to convert string values to numbers with precision, such as having two decimal places. This conversion is crucial when dealing with monetary values or measurements where exactness is key.
To achieve this, TypeScript offers built-in functions, and developers can leverage these functions to parse strings and format numbers effectively. Here’s a streamlined approach:
Using parseFloat and toFixed
- First, use the
parseFloat
function to convert the string to a float number. - Then, apply the
toFixed
method, which formats a number using fixed-point notation, rounding to a specified number of decimal places.
let stringToConvert = "123.456";
let numberWithTwoDecimals = parseFloat(stringToConvert).toFixed(2);
This code snippet results in a string “123.46” due to the rounding by toFixed
.
You can see the output in the screenshot below after executing the code using VS code.
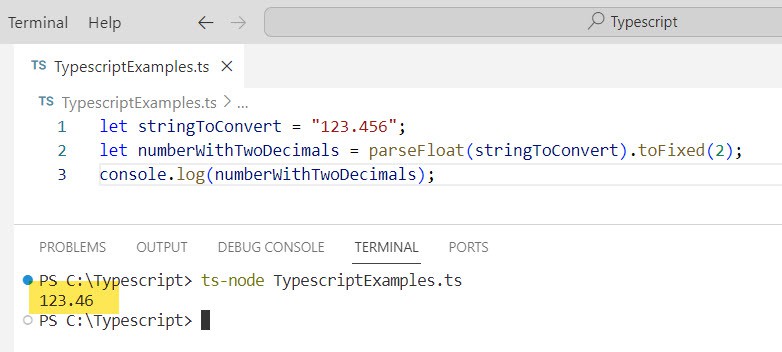
Ensuring Numeric Type
While toFixed
returns a string, you might need a literal number type for further numerical operations. To convert the string back to a number, prepend the +
unary operator.
let numericValue = +numberWithTwoDecimals;
numericValue
shall now be of type number with a value of 123.46.
Handling Non-Numeric Strings
If the input string does not contain a valid number, parseFloat
returns NaN
. Consequently, applying toFixed
to NaN
also yields ‘NaN’. It’s important when converting strings to implement checks for NaN
to avoid runtime errors.
Using these methods, one can reliably transform string data into numbers with exactly two decimal places. Efficient handling of such conversions is vital in producing accurate and reliable numeric representations in a TypeScript-based application.
TypeScript Convert Nullable String to Number
When dealing with nullable strings in TypeScript that need to be converted to numbers, one must handle the potential null
and undefined
values to ensure proper number conversion. TypeScript, being a typed superset of JavaScript, allows for various methods to handle this conversion.
Firstly, one must check for the null
or undefined
values before attempting to convert the string. This can be done using a straightforward conditional check:
let nullableString: string | null = ...; // Your nullable string
let numberValue: number | null = nullableString ? Number(nullableString) : null;
If the value is neither null
nor undefined
, the Number
function can be used to attempt a conversion. It is crucial to note that Number(null)
returns 0
, but in cases where null
should not default to 0
, the ternary operator, as shown above, preserves the null
value.
Consider the following scenarios:
- Simple Conversion: A string like
"123"
gets converted to123
. - Floating Points: If the string is
"123.45"
, it’s converted to123.45
. - Invalid Numbers: A string like
"abc"
or an empty string""
returnsNaN
(Not a Number).
For TypeScript specifics:
- Type Annotations: Ensure type safety by annotating the type as
number | null
for nullable numbers. - Strict Null Checks: Compile with
--strictNullChecks
to prevent unintendednull
orundefined
assignments.
Here’s a summary in tabular form for quick reference:
Input | Check null | Conversion Function | Output Type |
---|---|---|---|
"123" | No | Number(nullableString) | number |
null | Yes | Ternary check [1] | null |
"abc" | Conditional | Number(nullableString) | NaN |
[1] nullableString ? Number(nullableString) : null
In essence, converting nullable strings to numbers in TypeScript requires cautious checks and appropriate handling of special cases to maintain both type safety and the integrity of the values being processed.
TypeScript Convert Hex String to Number
In TypeScript, converting a hexadecimal string to a number can be efficiently achieved using the native parseInt
function with a radix of 16. This process involves passing two arguments to parseInt
: the string to be converted and the base for the radix conversion (16 for hexadecimal).
Example:
let hexString: string = "1f4";
let decimalNumber: number = parseInt(hexString, 16);
console.log(decimalNumber); // Output: 500
The parseInt
function parses the hex string up to the first character that is not a valid hex digit. If the string does not begin with a valid hex digit, parseInt
will return NaN
(Not a Number).
Handling NaN
Output:
let invalidHexString: string = "xyz";
let result: number = parseInt(invalidHexString, 16);
if (isNaN(result)) {
console.log("Invalid hex string"); // Output: Invalid hex string
}
When working with large hex numbers that may exceed the safe integer range, it’s recommended to use BigInt
for proper conversion without precision loss:
BigInt Conversion:
let largeHexString: string = "1f4b3c4d5e6f";
let bigIntNumber: BigInt = BigInt("0x" + largeHexString);
console.log(bigIntNumber); // Output: 54239351471119n
Prepending "0x"
to the hex string informs the BigInt
constructor that the string is a hexadecimal number.
Using these methods, developers can confidently handle hexadecimal string conversion to numbers, ensuring the resulting values are correctly parsed and assigned within their TypeScript applications.
Conclusion
In TypeScript, you can use various methods to convert a string to a number. One can opt for unary plus (+
) for a quick conversion of a simple numerical string. Functions like parseInt()
and parseFloat()
are suitable when needing to parse strings containing integers and floating-point numbers, respectively.
Method | Use Case |
---|---|
Number() | Converts any string to a number if possible |
parseInt() | Extracts an integer from the beginning of a string |
parseFloat() | Extracts a floating-point number from a string |
Unary plus (+ ) | Converts a simple numeric string to a number |
However, handling potential NaN (Not a Number) values that emerge when a string cannot be converted into a number is important. Consider validations to ensure that the conversion is safe and aligns with the expected data flows of your program. A developer should always account for edge cases, such as empty strings or strings with non-numeric characters to maintain the integrity of their software.
I hope you got the complete idea of how to convert string to number in Typescript.
You may also like:
- How to Convert a Dictionary to an Array in Typescript?
- How to convert an array to a dictionary in Typescript?
- How to Convert Typescript Dictionary to String?
- Convert Date to String Format dd/mm/yyyy in Typescript
- How to Convert String to Date in TypeScript?
- How to Convert String to Enum in Typescript?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com