In this tutorial, I have explained how to convert a dictionary to an array in Typescript using various methods with complete code and examples.
To convert a dictionary to an array in Typescript, you can use various methods like Object.keys(), Object.values(), etc. For example. const populationArray = Object.values(statePopulation); console.log(populationArray);
A dictionary in Typescript is a collection of key-value pairs, where each unique key maps to a value. In TypeScript, this is typically represented by an object or a Map. Arrays are fundamental data structures that are easy to iterate, sort, and manipulate. By converting dictionaries into arrays, we can leverage array methods and capabilities to work with our data more effectively.
Convert Dictionary to Array in Typescript
Here, I will show you the below three methods to convert a dictionary to an array in Typescript.
- Using Object.keys()
- Using Object.values()
- Using Object.entries()
Using Object.keys()
One of the various ways to convert a Typescript dictionary to an array is to use Object.keys(). This function returns an array of a given object’s own enumerable property names. Here is a complete code:
interface StatePopulation {
[state: string]: number;
}
const statePopulation: StatePopulation = {
California: 39538223,
Texas: 29145505,
Florida: 21538187,
NewYork: 20201249,
};
const statesArray = Object.keys(statePopulation).map((key) => ({
state: key,
population: statePopulation[key],
}));
console.log(statesArray);
Once I ran the code using the Visual Studio code editor, you can see the output below (check the screenshot also):
Output:
[
{ state: 'California', population: 39538223 },
{ state: 'Texas', population: 29145505 },
{ state: 'Florida', population: 21538187 },
{ state: 'NewYork', population: 20201249 }
]
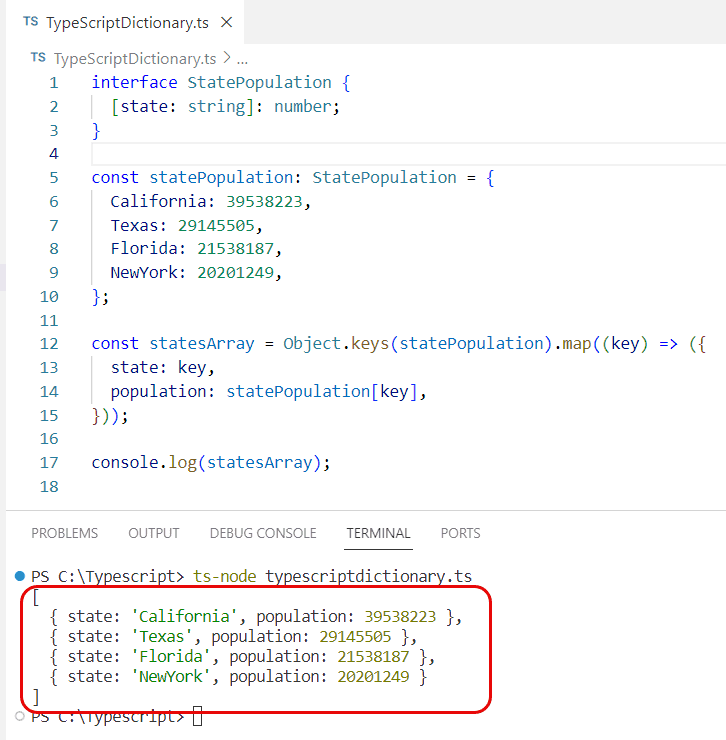
Using Object.values()
If we’re only interested in the values and not the keys, we can use Object.values()
If you want to get the values and not the keys, then you can use the object.values() code to convert the dictionary to an array in Typescript. Here is the complete code:
interface StatePopulation {
[state: string]: number;
}
// Example dictionary with state populations
const statePopulation: StatePopulation = {
California: 39538223,
Texas: 29145505,
Florida: 21538187,
NewYork: 20201249,
};
// Using Object.values() to get an array of population numbers
const populationArray = Object.values(statePopulation);
console.log(populationArray);
Output:
[ 39538223, 29145505, 21538187, 20201249 ]
Check out the screenshot below for the output after I ran the code using Visual Studio code.
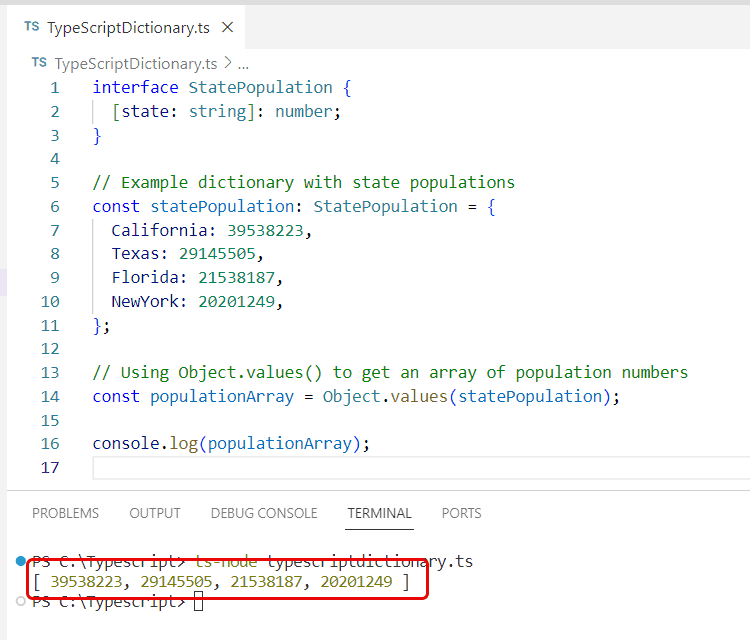
Using Object.entries()
When we need both keys and values in the form of an array, you can use the Object.entries() method in Typescript. This method retrieves both keys and values from the dictionary and converts them into an array of objects in Typescript.
interface StatePopulation {
[state: string]: number;
}
// Example dictionary with state populations
const statePopulation: StatePopulation = {
California: 39538223,
Texas: 29145505,
Florida: 21538187,
NewYork: 20201249,
};
// Using Object.entries() to get an array of objects with state and population
const stateEntriesArray = Object.entries(statePopulation).map(([state, population]) => ({
state,
population,
}));
console.log(stateEntriesArray);
Once I run the code, you can see the output below and check the screenshot.
Output:
[
{ state: 'California', population: 39538223 },
{ state: 'Texas', population: 29145505 },
{ state: 'Florida', population: 21538187 },
{ state: 'NewYork', population: 20201249 }
]
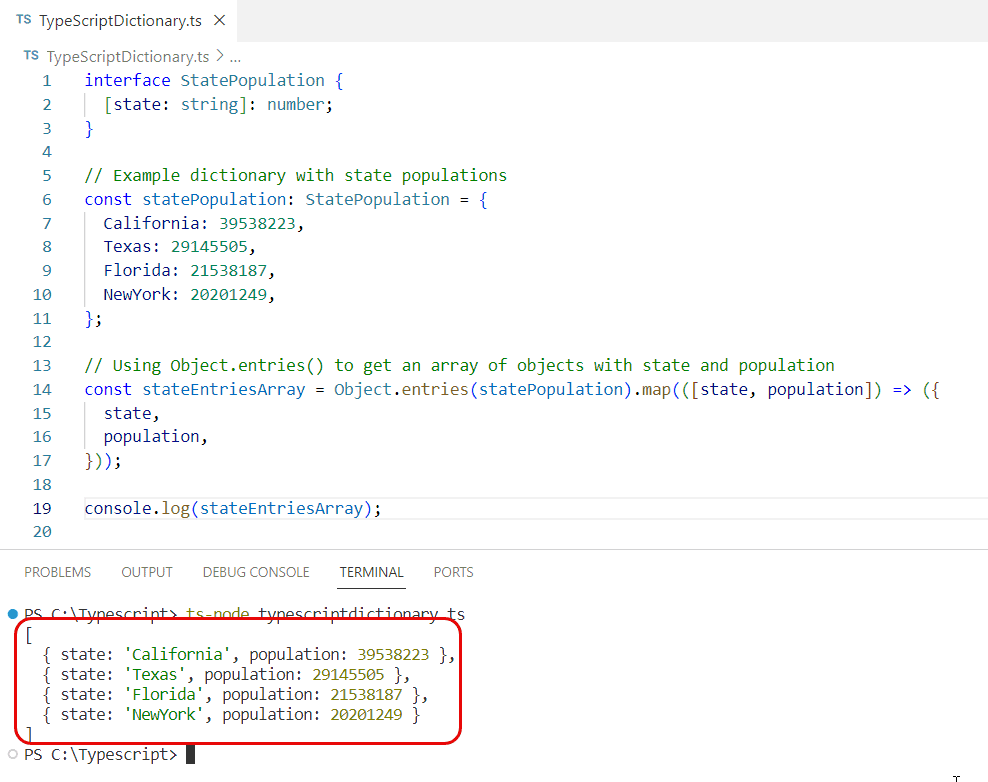
Conclusion
Typescript provides various methods to convert a dictionary to an array in Typescript, like
Object.keys(), Object.values(), or Object.entries(). In this tutorial, I have explained all of these methods with examples.
You may like the following tutorials:
- Remove Key from a Dictionary in Typescript
- How to Loop Through Dictionary in Typescript?
- How to initialize empty dictionary in Typescript?
- How to Sort a TypeScript Dictionary by Value?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com