This is a very common requirement: “Remove key from a dictionary in Typescript’. In this Typescript tutorial, I have explained different methods to remove a key from a dictionary in Typescript.
To remove key from dictionary in Typescript, you can use various methods like The delete Operator, Destructuring Assignment, and TypeScript’s utility types. The delete operator allows for direct key removal while destructuring with the rest syntax offers a more functional approach. You can use utility types like Omit, which can provide type-safe key omission in Typescript.
Remove Key from a Dictionary in Typescript
A dictionary in Typescript is essentially an object with a key-value pair, where the key is a string or a symbol, and the value can be of any type.
Here are the 3 methods to remove a key from a Typescript dictionary.
1. Using The delete Operator
The delete operator is the most straightforward way to remove a key from a dictionary in Typescript.
Here is a complete example of removing a key from a Typescript dictionary using the delete operator.
interface IDictionary {
[key: string]: any;
}
let myDictionary: IDictionary = {
"firstKey": 1,
"secondKey": 2,
"thirdKey": 3
};
delete myDictionary["secondKey"];
console.log(myDictionary); // Output: { "firstKey": 1, "thirdKey": 3 }
Here, I run the code using Visual Studio Code and the output in the screenshot below.
{ firstKey: 1, thirdKey: 3 }
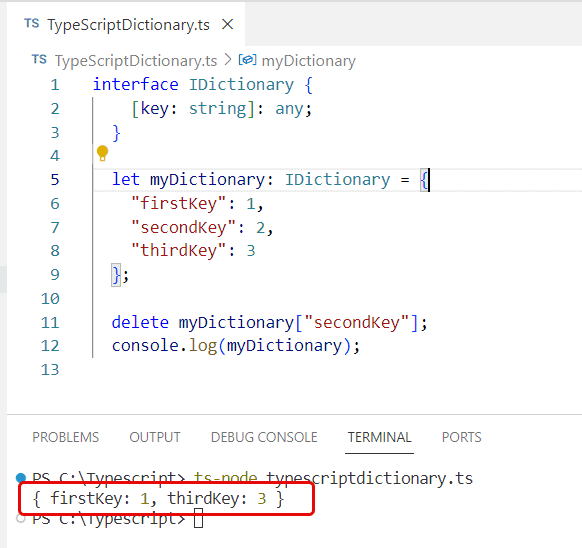
2. Using Destructuring Assignment
You can also use the destructuring assignment to remove keys from a Typescript dictionary.
Below is a complete example of using a destructuring assignment to remove a key from a dictionary in TypeScript.
// Define the dictionary interface
interface IDictionary {
[key: string]: any;
}
// Initialize the dictionary with some key-value pairs
let myDictionary: IDictionary = {
firstKey: 1,
secondKey: 'value2',
thirdKey: true
};
// The key you want to remove
const keyToRemove = "secondKey";
// Use destructuring to exclude the keyToRemove and gather the rest of the properties
const { [keyToRemove]: omitted, ...rest } = myDictionary;
// rest now holds the new dictionary without the keyToRemove
myDictionary = rest;
// Log the updated dictionary to the console
console.log(myDictionary);
You can check out the screenshot below after I ran the code using the Visual Studio Code editor.
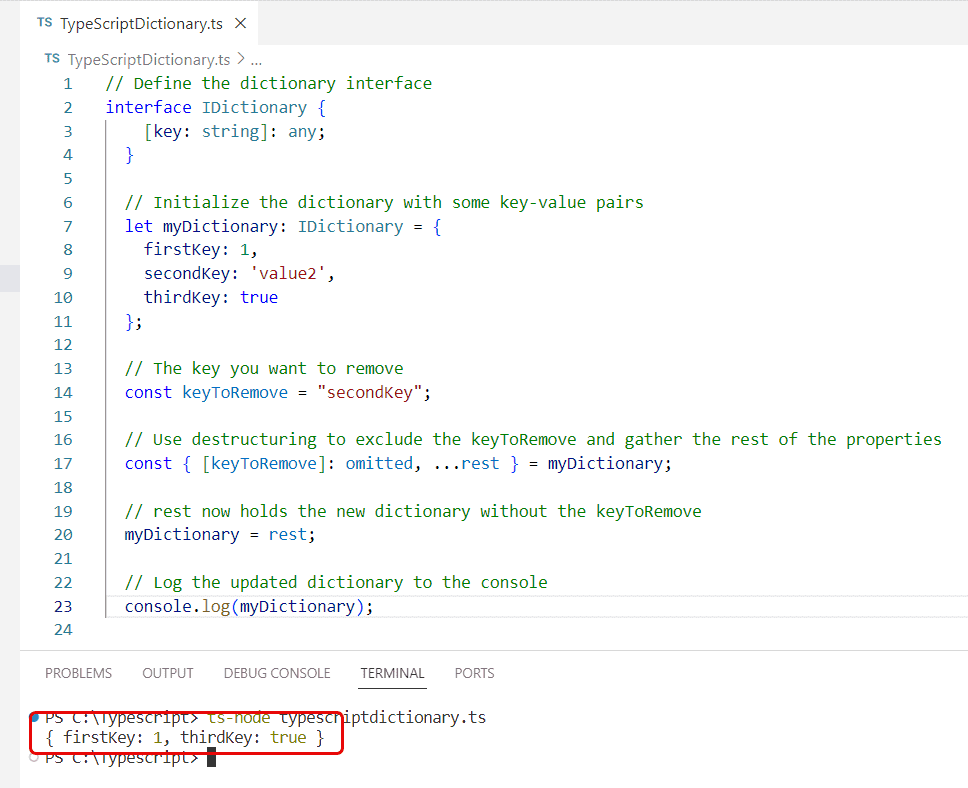
3. Using TypeScript’s utility types
For a type-safe way to remove keys, consider using TypeScript’s utility types. Here is a complete example of removing a Key from a Dictionary in Typescript.
type OmitKey<T, K extends keyof any> = Pick<T, Exclude<keyof T, K>>;
function removeKey<T, K extends keyof T>(obj: T, ...keys: K[]): OmitKey<T, K> {
let modifiedObj = { ...obj };
keys.forEach(key => {
delete modifiedObj[key];
});
return modifiedObj as OmitKey<T, K>;
}
myDictionary = removeKey(myDictionary, "thirdKey");
console.log(myDictionary); // Output: { "firstKey": 1 }
This function removeKey() takes a dictionary and the keys to remove, and returns a new dictionary without the specified keys in Typescript
Conclusion
In this Typescript tutorial, I have explained how to remove a key from a dictionary in Typescript using various methods with examples.
- Using The delete Operator
- Using Destructuring Assignment
- Using TypeScript’s utility types
You may also like:
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com