Do you want to know how to declare and initialize a dictionary in typescript? In this tutorial, I will explain how to declare a dictionary in Typescript.
To declare a dictionary in typescript, you can use either an object with an index signature, such as { [key: string]: YourValueType }, or by leveraging the Map object, like new Map<KeyType, ValueType>(). By specifying types for both keys and values, TypeScript’s powerful type-checking ensures that your dictionary is predictable and your code is robust.
A typescript dictionary is a collection that stores elements as key-value pairs. TypeScript, being a superset of JavaScript, allows us to use plain objects as dictionaries by default, but it also provides the Map object, which offers additional benefits like better performance for frequent additions and deletions.
Declare a Dictionary in TypeScript using an Object
The simplest way to declare a dictionary in TypeScript is by using an object. Here’s how you can declare one dictionary in a Typescript example.
let dictionary: { [key: string]: any } = {};
In the above example, a dictionary is an object that will store keys of type string and values of any type. The syntax { [key: string]: any } defines an index signature, which tells TypeScript that this object can have any number of properties as long as the keys are strings.
Example with Specific Value Types
However, to ensure type safety and make the dictionary more predictable, you might want to specify the type of values that your dictionary will hold:
interface IDictionary {
[key: string]: number;
}
let salaryDictionary: IDictionary = {
"John Doe": 50000,
"Jane Smith": 75000,
"Alex Johnson": 65000,
};
console.log(salaryDictionary["John Doe"]); // Outputs: 50000
In the above code snippet, IDictionary is an interface that enforces all values in the dictionary to be of type number. This is particularly useful when dealing with structured data, like a collection of salaries.
You can see I ran the code using Visual Studio Code, and the output was given as 5000, as shown in the below screenshot.
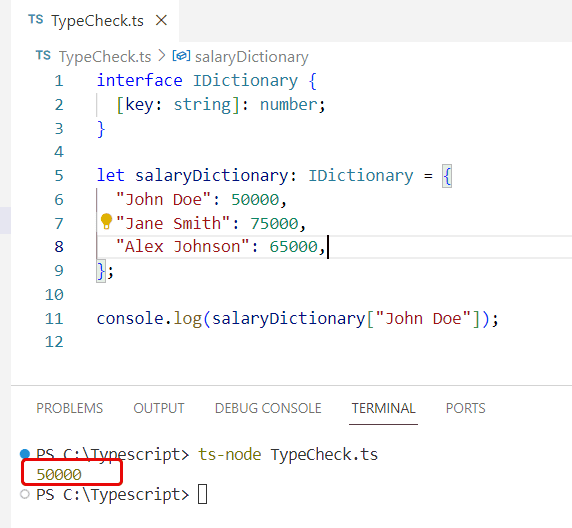
This is one way of declaring typescript using an object.
Declare a dictionary in Typescript using Map
Now, let us see how to declare a dictionary in Typescript using Map.
TypeScript also provides the Map object, which can be used as a dictionary. Here’s a simple declaration:
let dictionary: Map<string, any> = new Map();
This creates a new Map that accepts strings as keys and can have any type of values. The advantage of using Map over plain objects is that Map is an iterable, which allows for easier iteration over elements and has a better performance for large sets of data.
Example with Map
Let’s look at an example of a Map that has specific value types:
let userRoles: Map<string, string> = new Map([
["john.doe@example.com", "Admin"],
["jane.smith@example.com", "User"],
["alex.johnson@example.com", "User"]
]);
console.log(userRoles.get("john.doe@example.com"));
In this example, userRoles is a Map that connects user email addresses to their respective roles in the system. By using .get(), we can retrieve the value associated with a specific key.
I ran the code using Visual Studio Code, and you can see the output of this Typescript program in the screenshot below.
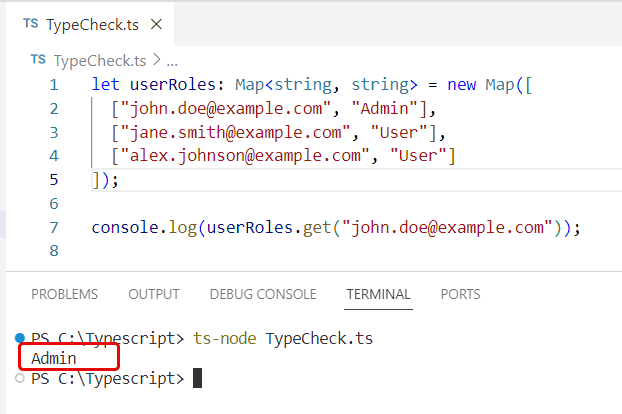
This is how to declare a dictionary in typescript using a map object.
Conclusion
By using either plain objects with index signatures or the Map object, developers can create dictionaries in Typescript. In this tutorial, I have explained to you with examples of how to declare a dictionary in typescript. It is good always to specify your types and consider the nature of your data when choosing between an object or a Map.
You may also like:
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com