There will be many times you need to iterate through a dictionary in Typescript. In this Typescript tutorial, I will explain different methods to loop through dictionary in Typescript.
To loop through a dictionary in Typescript, you can use either the traditional for…in loop or the more modern Object methods like Object.keys(), Object.values(), and Object.entries(). These approaches allow for efficient traversal of keys and values, facilitating operations on dictionary data structures with precision and conciseness.
Typescript loop through dictionary
Now, let us check the various methods to iterate through a dictionary in Typescript. A few examples of “Typescript dictionary forEach”.
A dictionary is essentially an object with string keys and values of any type. TypeScript’s type system allows us to define the type of values stored in the dictionary, ensuring consistency and type safety during iteration.
Loop through a dictionary in Typescript using the for…in Loop
One of the simplest ways to iterate through dictionary objects in TypeScript is by using the for…in loop. This method traverses all enumerable properties of the Typescript dictionary.
Here is a complete example.
// Dictionary
let userScores: { [key: string]: number } = {
alice: 10,
bob: 20,
charlie: 30
};
// Typescript loop through dictionary using for...in
for (let user in userScores) {
console.log(`User: ${user}, Score: ${userScores[user]}`);
}
Once you run the code using Visual Studio Code, you can see the output in the below screenshot.
User: alice, Score: 10
User: bob, Score: 20
User: charlie, Score: 30
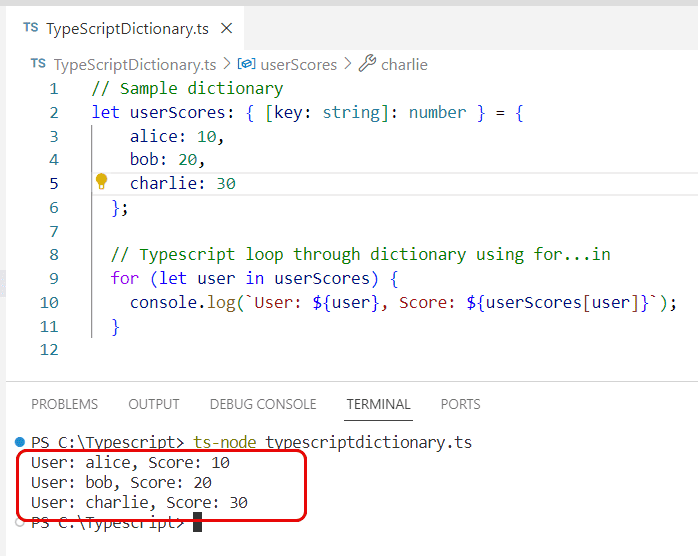
Typescript iterate over a dictionary using object methods
Now, let us see how to iterate over a dictionary using object methods in Typescript.
TypeScript provides us with Object.keys(), Object.values(), and Object.entries() methods. These functions return arrays that can be easily iterated over using Array.prototype.forEach(), for…of, or other array looping methods.
Iterate over keys with Object.keys()
The Object.keys() method returns an array of a dictionary’s own enumerable property names, iterated in the same order as that provided by a for…in loop in Typescript.
Object.keys(userScores).forEach(key => {
console.log(`User: ${key}, Score: ${userScores[key]}`);
});
Iterate over values with Object.values()
The Object.values() method returns an array of a dictionary’s own enumerable property values in Typescript.
Object.values(userScores).forEach(value => {
console.log(`Score: ${value}`);
});
Iterate over entries with Object.entries()
The Object.entries() method provides an array of a dictionary’s own enumerable property [key, value] pairs in Typescript.
Object.entries(userScores).forEach(([key, value]) => {
console.log(`User: ${key}, Score: ${value}`);
});
Iterate through a dictionary in Typescript using for…of with Object.entries()
You can also use the for…of syntax with Object.entries() to loop through a dictionary in TypeScript objects. Here is the code:
for (let [key, value] of Object.entries(userScores)) {
console.log(`User: ${key}, Score: ${value}`);
}
Here is the complete example:
// Define a dictionary interface with string keys and any type of values
interface IDictionary {
[key: string]: any;
}
// Create a dictionary with some data
const myDictionary: IDictionary = {
key1: 'value1',
key2: 100,
key3: true,
key4: [1, 2, 3]
};
// Use for...of with Object.entries() to iterate over dictionary entries
for (const [key, value] of Object.entries(myDictionary)) {
console.log(`Key: ${key}, Value: ${value}`);
}
Here, you can see in the screenshot below the output after I executed the code using Visual Studio Code.
Key: key1, Value: value1
Key: key2, Value: 100
Key: key3, Value: true
Key: key4, Value: 1,2,3
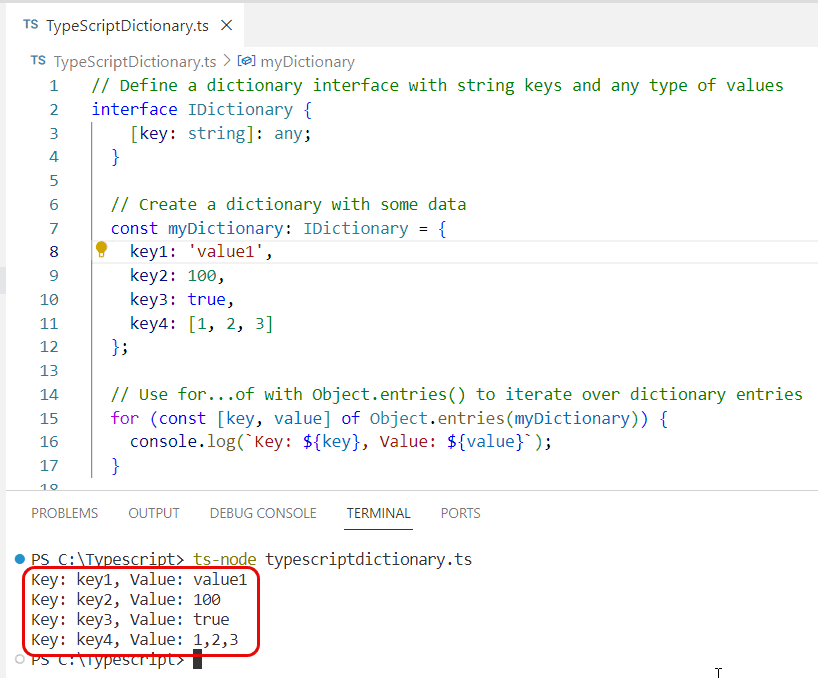
Conclusion
To efficiently loop through a dictionary in TypeScript, developers can use various methods ranging from traditional for…in loops to more modern methods provided by Object.keys(), Object.values(), and Object.entries(). In this tutorial, I explained all these methods with complete code with an example of iterating through a dictionary in Typescript.
You may also like:
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com