Do you want to learn about the Typescript dictionary? Check out this tutorial; I will explain here how to work with a dictionary in typescript.
In TypeScript, a dictionary is a collection of key-value pairs, where each unique key maps to a value. It is similar to an object or a map in JavaScript, providing a way to store data that can be efficiently looked up by keys. TypeScript allows for strict typing of these keys and values, ensuring a more predictable and error-resistant codebase.
Create a Dictionary in TypeScript
Now, let us see how to create a dictionary in Typescript.
To define a dictionary in TypeScript, you can use the Record<Keys, Type> utility type or an index signature. Here’s how you can define a dictionary with string keys and string values:
type StringDictionary = Record<string, string>;
// Or using an index signature
interface IDictionary {
[key: string]: string;
}
Now, let us see how to initialize a dictionary directly using object literals in Typescript:
let capitals: StringDictionary = {
"USA": "Washington, D.C.",
"Canada": "Ottawa",
"Mexico": "Mexico City"
};
Add Items to a Dictionary
To add an item to a TypeScript dictionary, simply assign a value to a new key:
capitals["Germany"] = "Berlin";
Get Items from a Dictionary
You can access the value associated with a specific key using the indexing syntax:
let capitalOfUSA = capitals["USA"];
Checking for Existence of a Key
Use the in operator to check if a key exists in a Typescript dictionary:
let hasCanada = "Canada" in capitals; // true
Iterate Over a Dictionary in Typescript
To iterate over the keys and values of a dictionary in Typescript, you can use Object.keys, Object.values, or Object.entries:
// Iterating over keys
for (let country of Object.keys(capitals)) {
console.log(country);
}
// Iterating over values
for (let capital of Object.values(capitals)) {
console.log(capital);
}
// Iterating over entries
for (let [country, capital] of Object.entries(capitals)) {
console.log(`The capital of ${country} is ${capital}`);
}
Remove Items from a Dictionary
To remove an item from a Typescript dictionary, delete the key-value pair using the delete operator:
delete capitals["Mexico"];
Here is a complete example of a Typescript dictionary.
// Define the dictionary interface
interface IDictionary {
[index: string]: number;
}
// Create a dictionary to store the ages of people
let ageDictionary: IDictionary = {};
// Populate the dictionary
ageDictionary["Alice"] = 25;
ageDictionary["Bob"] = 30;
ageDictionary["Charlie"] = 28;
// Retrieve a value
let aliceAge = ageDictionary["Alice"]; // 25
// Check if a key exists
let hasBob = "Bob" in ageDictionary; // true
// Iterate over the dictionary
for (let name in ageDictionary) {
console.log(`${name}: ${ageDictionary[name]}`);
}
// Remove an item
delete ageDictionary["Charlie"];
// The updated dictionary no longer has Charlie
console.log(ageDictionary);
You can see in the screenshot below I have executed the complete code in Visual Studio Code.
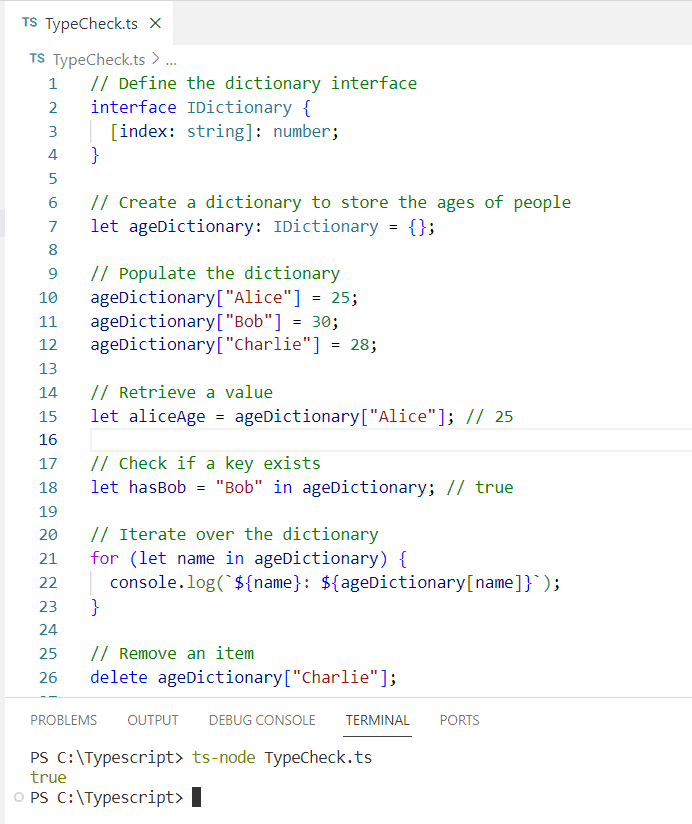
Conclusion
In this tutorial, we’ve learned about TypeScript dictionaries and their use cases, which include defining, initializing, adding, retrieving, checking, iterating, and removing dictionary items in Typescript.
- How to Loop Through Dictionary in Typescript?
- How to Declare a Dictionary in TypeScript?
- How to Remove Key from a Dictionary in Typescript?
- How to check if a key exists in a dictionary in typescript?
- How to Check if a Dictionary is Empty in TypeScript?
- How to Find Dictionary Length in TypeScript?
- How to initialize empty dictionary in Typescript?
- How to Sort a TypeScript Dictionary by Value?
- How to Convert a Dictionary to an Array in Typescript?
- How to check if value exists in dictionary in Typescript?
- How to get value from a dictionary in Typescript?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com