Do you want to sort a dictionary in Typescript? In this tutorial, I will explain various methods to sort a Typescript dictionary by value.
To sort a Typescript dictionary by value, you can use various methods like Using Array’s sort Function, using custom comparator function. The easiest method is to use the array’s sort function like items.sort((a, b) => a[1] – b[1]);.
Sort a TypeScript Dictionary by Value
Let us check a few Typescript sort dictionary examples. We will see 3 methods to sort a dictionary by value in Typescript.
1. Using Array’s sort Function
The first and most straightforward method involves converting the dictionary into an array of tuples, sorting the array, and then, if needed, converting it back to an object.
Here is a complete code:
// Define our dictionary with USA city names as keys and population numbers as values
const cityPopulation: Record<string, number> = {
Chicago: 2716000,
NewYork: 8419000,
LosAngeles: 3971000
};
// Convert the dictionary into an array of [city, population] tuples
const cityItems = Object.keys(cityPopulation).map(city => [city, cityPopulation[city]] as [string, number]);
// Sort the array by the population, which is the second element of the tuple
cityItems.sort((a, b) => a[1] - b[1]);
// If you need the sorted object back
const sortedCityPopulation: Record<string, number> = {};
cityItems.forEach(([city, population]) => {
sortedCityPopulation[city] = population;
});
console.log(sortedCityPopulation);
Here, you can see the output below in the screenshot after it sorts the Typescript dictionary by value.
{ Chicago: 2716000, LosAngeles: 3971000, NewYork: 8419000 }
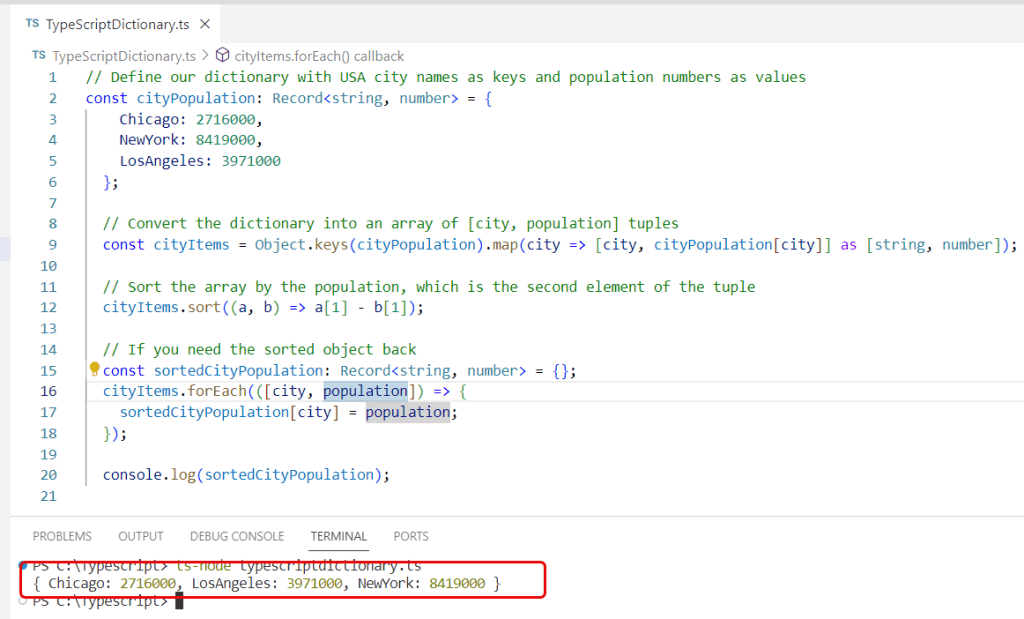
2. Using Custom Comparator Function
For more complex sorting logic, such as when values are objects themselves or when you want to sort by multiple criteria, you can use a custom comparator function in Typescript to sort a dictionary.
Here is a complete code:
interface CityInfo {
name: string;
population: number;
}
// Dictionary with complex structure
const cityDetails: Record<string, CityInfo> = {
Chicago: { name: "Chicago", population: 2716000 },
NewYork: { name: "NewYork", population: 8419000 },
LosAngeles: { name: "LosAngeles", population: 3971000 }
};
// Custom comparator function for sorting by population
function compareByPopulation(a: [string, CityInfo], b: [string, CityInfo]) {
return a[1].population - b[1].population;
}
const cityEntries = Object.entries(cityDetails);
cityEntries.sort(compareByPopulation);
const sortedCityDetails: Record<string, CityInfo> = {};
cityEntries.forEach(([city, info]) => {
sortedCityDetails[city] = info;
});
console.log(sortedCityDetails);
Output:
{
Chicago: { name: 'Chicago', population: 2716000 },
LosAngeles: { name: 'LosAngeles', population: 3971000 },
NewYork: { name: 'NewYork', population: 8419000 }
}
You can see the screenshot also for the output after I executed the code using VS code.
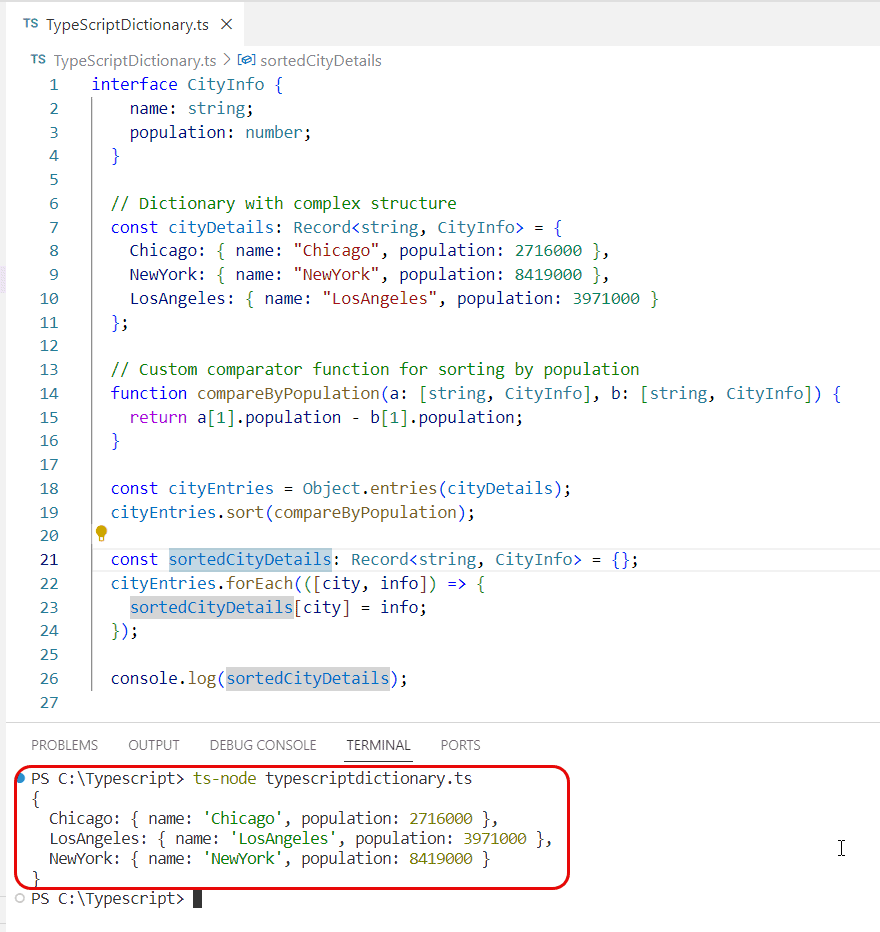
3. Sort a Typescript dictionary by values in descending order
If you want to sort the dictionary by values in descending order, you simply modify the comparator function to reverse the comparison. Here is a complete code:
const cityPopulation: Record<string, number> = {
Chicago: 2716000,
NewYork: 8419000,
LosAngeles: 3971000
};
const cityItemsDescending = Object.keys(cityPopulation).map(city => [city, cityPopulation[city]] as [string, number]);
// Sort the array by the population in descending order
cityItemsDescending.sort((a, b) => b[1] - a[1]);
const sortedCityPopulationDescending: Record<string, number> = {};
cityItemsDescending.forEach(([city, population]) => {
sortedCityPopulationDescending[city] = population;
});
console.log(sortedCityPopulationDescending);
You can see the screenshot below and the output after executing the Typescript code.
Output:
{ NewYork: 8419000, LosAngeles: 3971000, Chicago: 2716000 }
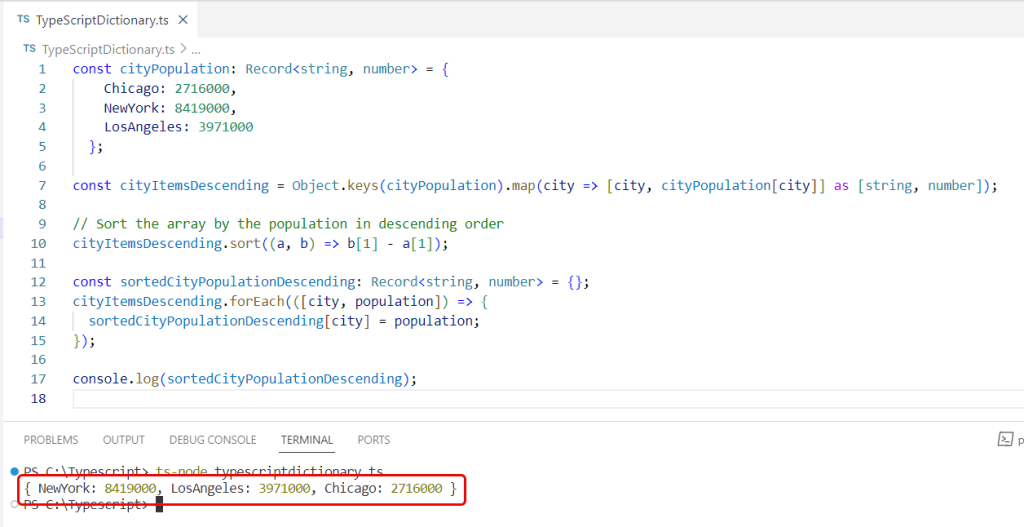
Conclusion
Sorting a dictionary by value in TypeScript can be achieved through several methods like simple key-value pairs or complex objects, TypeScript provides you with the tools to order your dictionaries as needed. Remember to leverage TypeScript’s type system to maintain the integrity of your data throughout the sorting process. By following the steps and code provided, you can implement sorting in your TypeScript projects.
You may also like:
- How to initialize empty dictionary in Typescript?
- How to Convert a Dictionary to an Array in Typescript?
- Typescript Compare Strings
- How to Check if a Dictionary is Empty in TypeScript?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com