Do you want to map an object to another in Typescript? This tutorial is everything about “Typescript map array of objects.” I will show you how to effectively use TypeScript to map through arrays of objects using mapping methods.
To map an array of objects in Typescript, you can use the map() method. By the end of the tutorial, you will get to know how to convert array of objects to another type in Typescript.
Typescript map object to another
TypeScript, you might often find the need to map an object to another object with a different shape or structure. Here’s an example where we have a Person object, and we want to map it to a Contact object in Typescript.
// Defining our starting object structure
interface Person {
firstName: string;
lastName: string;
age: number;
}
// Defining the target object structure
interface Contact {
fullName: string;
isAdult: boolean;
}
// Our function that maps a Person to a Contact
function mapPersonToContact(person: Person): Contact {
return {
fullName: `${person.firstName} ${person.lastName}`,
isAdult: person.age >= 18
};
}
// Example usage:
const person: Person = {
firstName: 'John',
lastName: 'Doe',
age: 30
};
const contact: Contact = mapPersonToContact(person);
console.log(contact);
In this Typescript code, we define two interfaces: Person and Contact. The mapPersonToContact function takes a Person object as input and produces a Contact object. The new Contact object has a fullName property, which is a concatenation of firstName and lastName from the Person object, and an isAdult property, which is a boolean indicating whether the person’s age is 18 or above.
Here you can see the output in the screenshot below:
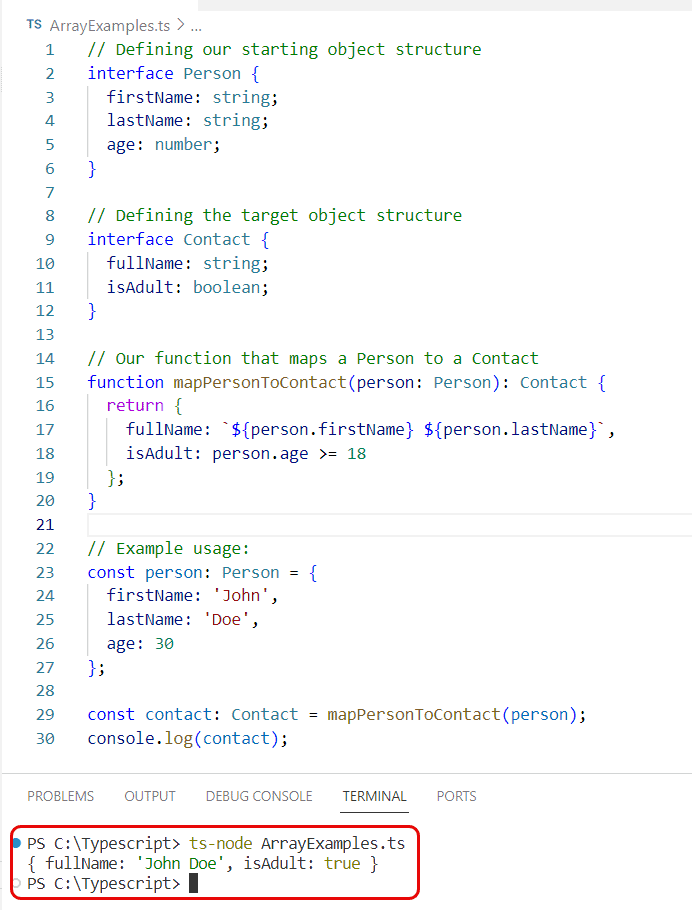
Typescript map array of objects
TypeScript’s map function is a method that you can use on arrays, which allows you to transform each item in the array and create a new array with the transformed items. When we talk about a “TypeScript map array of objects,” we’re referring to using the map method on an array where each item is an object.
Let us first understand what an array of objects in TypeScript looks like.
An array of objects is a collection where each element is an object that can hold multiple properties. TypeScript enhances these arrays by allowing us to define the type of objects they contain. Here is an example.
interface User {
id: number;
name: string;
}
const users: User[] = [
{ id: 1, name: 'Alice' },
{ id: 2, name: 'Bob' },
{ id: 3, name: 'Charlie' }
];
Typescript convert array of objects to another type using Map Through Arrays
Mapping is a method that transforms elements in an array into a new form, based on a given function. The Typescript map() method is the bread and butter for array transformations. It calls a provided function on every element in the array and returns a new array that contains the results.
Here is the code:
// Define what our "card" looks like with a name and age
interface Person {
name: string;
age: number;
}
// Create a list of these "cards"
const people: Person[] = [
{ name: 'Alice', age: 25 },
{ name: 'Bob', age: 30 },
{ name: 'Carol', age: 35 },
];
// Use map to create a new list with just the names
const names = people.map((person) => person.name);
console.log(names);
In this example, people is an array of objects where each object has a name and an age. We use map to go through that array, and for each person, we take just their name and make a new array of names. So, the map() function helps us to transform an array of objects into a new array of just the values we want.
If you run the code using Visual Studio Code, you can see the output like the screenshot below.
[ 'Alice', 'Bob', 'Carol' ]
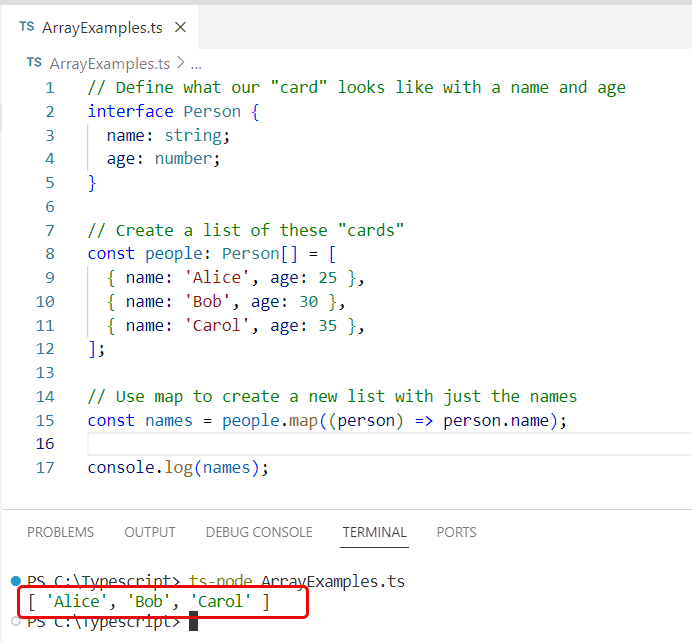
Typescript map array to object using map() with TypeScript Generics
TypeScript generics add a layer of type safety to the map() method. With generics, you can ensure that the mapping function adheres to a specific contract, which can be beneficial when dealing with complex data structures.
Using the map() function with TypeScript generics allows you to create a more flexible and reusable mapping function that can work with arrays of any type. Let me provide you with a complete example of how to use map() with TypeScript generics.
First, let’s define a generic function called mapArray that will take an array of any type T, and a transformation function that will convert any element of type T to another type K. The function will return a new array of the transformed elements of type K.
function mapArray<T, K>(array: T[], transform: (item: T) => K): K[] {
return array.map(transform);
}
// Example usage:
// Let's define a simple User interface
interface User {
id: number;
name: string;
}
// And an array of users
const users: User[] = [
{ id: 1, name: 'Alice' },
{ id: 2, name: 'Bob' },
{ id: 3, name: 'Charlie' },
];
// Now we can use our generic mapArray function to get an array of user names
const userNames = mapArray(users, user => user.name);
console.log(userNames); // This will output: ['Alice', 'Bob', 'Charlie']
// We can also use it to transform the array of users into an array of lengths of names
const nameLengths = mapArray(users, user => user.name.length);
console.log(nameLengths);
In this code, mapArray is a generic function where T is the type of the elements in the input array, and K is the type of elements in the output array. The transform parameter is a function that dictates how each element of the input array should be transformed into an element of the output array.
We’ve used mapArray in two ways:
- To transform our array of User objects into an array of string values, which are the names of the users.
- To transform the same array of User objects into an array of number values, representing the length of each user’s name.
Here is the output in the below screenshot.
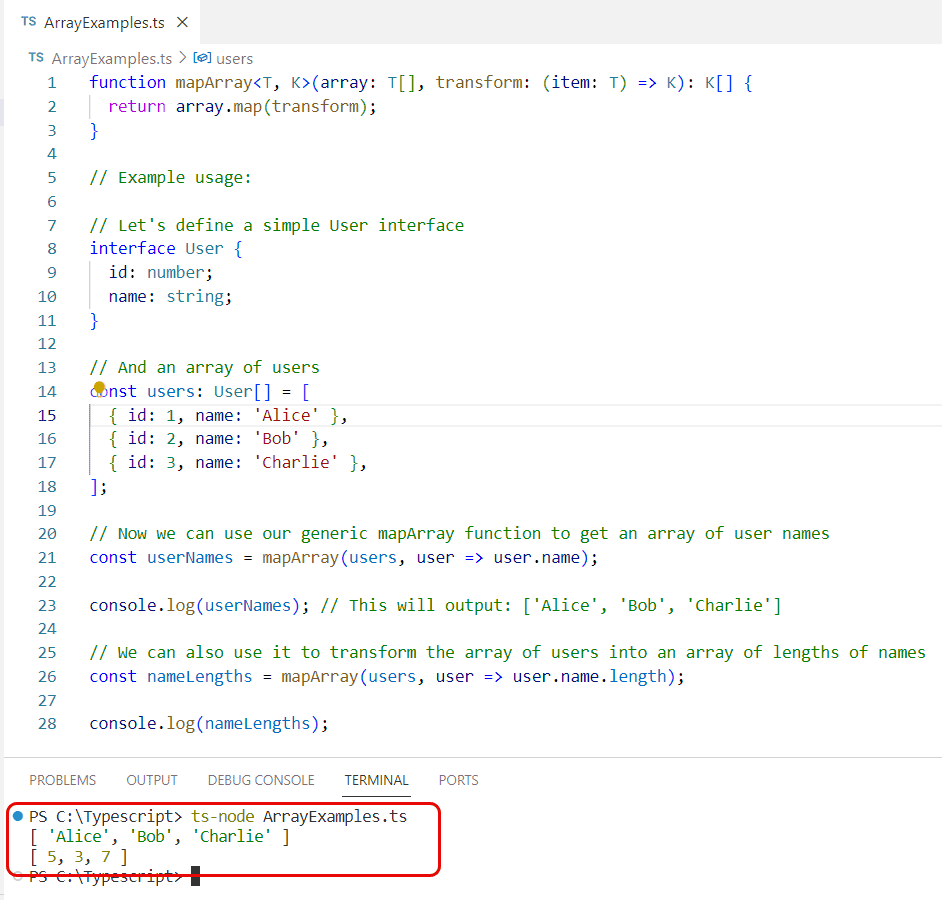
map array of objects in Typescript using custom function
For more complex mappings, you might need to write a custom function that could include additional logic, such as conditionals or error handling.
Custom mapping functions allow us to perform more complex transformations on arrays, beyond what the standard map() method offers. Below is a TypeScript code example that demonstrates how to create and use a custom mapping function to map array of objects in Typescript
// Let's start by defining an interface for our objects.
interface User {
id: number;
name: string;
isActive: boolean;
}
// Now, we create an array of User objects.
const users: User[] = [
{ id: 1, name: 'Alice', isActive: true },
{ id: 2, name: 'Bob', isActive: false },
{ id: 3, name: 'Charlie', isActive: true },
];
// This is our custom map function.
// It will iterate over each item in the array and apply a custom transformation.
function customMap<T, K>(array: T[], transform: (item: T) => K): K[] {
const result: K[] = [];
for (const item of array) {
const transformedItem = transform(item);
if (transformedItem !== null && transformedItem !== undefined) {
result.push(transformedItem);
}
}
return result;
}
// Let's use the customMap function to create an array of names from active users.
const activeUserNames = customMap(users, (user) => {
if (user.isActive) {
return user.name;
}
});
console.log(activeUserNames);
// Output should be: ['Alice', 'Charlie'], since Bob is not active.
// Here's another example, where we transform users into a different format.
const formattedUsers = customMap(users, (user) => ({
userId: `user_${user.id}`,
isActive: user.isActive ? "Yes" : "No",
}));
console.log(formattedUsers);
Conclusion
Mapping through arrays of objects in TypeScript is a very important concept in Typescript. It provides the power to transform data structures in a type-safe manner, ensuring that your applications run as expected. By using the map() method, along with TypeScript’s generics and advanced techniques, you can map array of objects in Typescript.
You may also like:
- Convert Typescript Array to String With Separator
- Get Key by Value from enum String in Typescript
- How to push an object into an array in Typescript
- Sort array of objects in typescript
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com