In this typescript tutorial, we will see how to get key by value from enum string in Typescript by using different methods. These methods are:
- Using Object.value, indexOf(), and Object.key
- Using a for… in loop
- Using Object.Keys
- Using a type assertation
Read: How to push an object into an array in Typescript
Using Object.value, indexOf(), and Object.key
Here we will see how to use Object.value(), indexOf(), and Objeck.key() to enum gets key by string value in typescript.
The built-in function Object.values() returns an array of values from an object’s properties in TypeScript and JavaScript.
The built-in TypeScript function Object.keys() provides an array containing a given object’s own enumerable property names, in an identical chronological sequence as a for…in loop.
The built-in function indexOf() in TypeScript returns the position of the first instance of a given element within an array. It returns -1 if the element cannot be located.
For example, we will use the Object. value, to retrieve an array of enum values, then use the indexOf() to get the index of the enum value from an array. After that, we will use the Object.key, to retrieve the enum key of an array.
In the code editor, create a file named as ‘enumGetsKeyByStringValue.ts’ file, and write the below code:
enum Country{
USA= "usa",
UK="uk",
Australia= "australia"
}
const indexOfUK = Object.values(Country).indexOf('uk' as unknown as Country);
const key = Object.keys(Country)[indexOfUK];
console.log(key); // 👉️output: "UK"
To compile the code, run the below command and you can see the result in the console.
ts-node enumGetsKeyByStringValue.ts
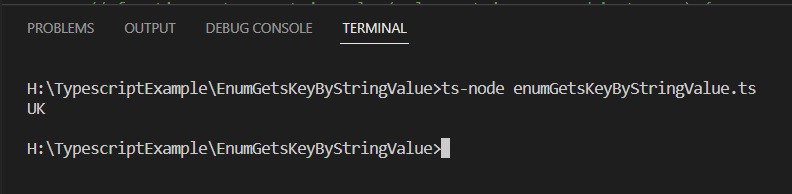
This is how we can enum gets by string value in typescript by using the object.key, object. value and indexOf().
Check out: How to get string between 2 characters in Typescript
Using a for… in loop
Here we will see how to enum gets key by string value in typescript by using for…in loop.
The for..in loop in typescript is used to loop through the properties of an object. Also, this is used to iterate over the key of an object and do some action on each key
Syntax:
for (let key in object) {
// Code to be executed for each key
}
For example, we will create an enum Country with three properties (USA, UK, and Australia).Then create a function ‘getKeyByStringValue’, inside this create a ‘for..in’ loop that iterates over the key i.e. Property names of the enum object, and checks whether the current property value matches the input value.
If the key is found, then the function returns the key, else returns null.
In the ‘enumGetsKeyByStringValue.ts’ file write the below code.
enum Country{
USA= "usa",
UK="uk",
Australia= "australia"
}
function getKeyByStringValue(value: string, enumObject: any) {
for (const key in enumObject) {
if (enumObject[key] === value) {
return key;
}
}
return null;
}
console.log(getKeyByStringValue("uk", Country));
To compile the code, run the below command and you can see the result in the console.
ts-node enumGetsKeyByStringValue.ts
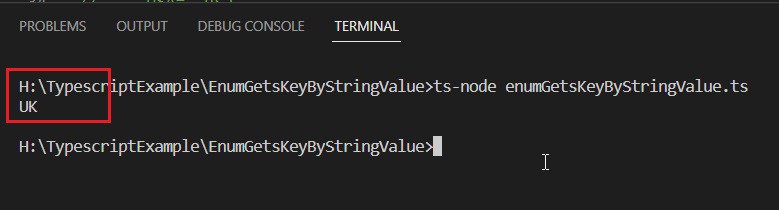
This is an example of an enum gets a key by string value in typescript by using for…in a loop.
Using Object.Keys
Here we will see how to enum gets a key by string value in typescript by using Object.key.
The built-in TypeScript function Object.keys() provides an array containing a given object’s own enumerable property names, in an identical chronological sequence as a for…in a loop.
For example, we will create an enum Country with three properties (USA, UK, and Australia). Then we will define a function ‘getKeyByStringValue’ using the object.key returns an array of keys i.e. property name from the enum objects, and then we will filter the array to get the key whose value matches the input string.
If the key is found, then the function returns the key else returns null.
In the ‘enumGetsKeyByStringValue.ts’ file write the below code.
enum Country{
USA= "usa",
UK="uk",
Australia= "australia"
}
function getKeyByStringValue(value: string, enumObject: any) {
return Object.keys(enumObject).find(key => enumObject[key] === value) || null;
}
console.log(getKeyByStringValue("usa", Country)); // Output: USA
To compile the code, run the below command and you can see the result in the console.
ts-node enumGetsKeyByStringValue.ts
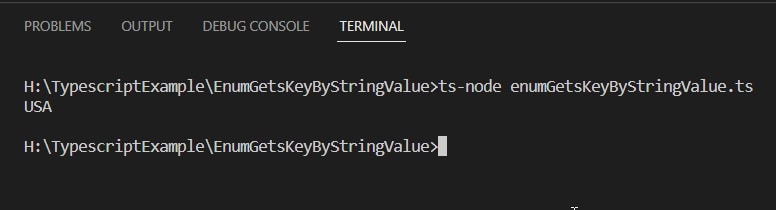
This is an example of an enum that gets key by string value in typescript by using Object.keys
Read: How to convert string to double in typescript
Using a type assertation
Here we will see how an enum gets by string value in typescript by using the object.key, object. value and indexOf().
With typescript, type assertation is a way to convey to the compiler that we know the type of a value more precisely than the compiler assigns automatically. Also, it enables us to override the type of variable or expression and provide a type that we know it will be.
For example create an enum Country with the three values USA, UK, and Australia. Then create the function getKeyByStringValue, which accepts an enum object and a string value as input and returns the key (property name) of the enum whose value matches the input string.
In the ‘enumGetsKeyByStringValue.ts’ file write the below code.
enum Country{
USA= "usa",
UK="uk",
Australia= "australia"
}
function getKeyByStringValue(value: string, enumObject: any) {
return (Object.entries(enumObject) as Array<[string, string]>)
.find(([key, enumValue]) => enumValue === value)?.[0] || null;
}
console.log(getKeyByStringValue("usa", Country));
To compile the code run the below command and you can see the result in the console:
ts-node enumGetsKeyByStringValue.ts
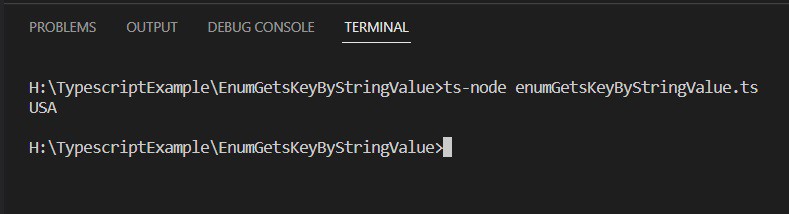
This is an example of an enum gets key by string value in typescript by using type assertation.
Additionally, you may like some more typescript tutorials:
- How to get string after character in Typescript
- Typescript string enum reverse mapping
- Sort array of objects in typescript [With 10 examples]
- How to convert a string to boolean in typescript [using 4 different ways]
- Typescript Array to String With Separator
Conclusion
In this typescript tutorial, we saw how to enum gets key by string value in typescript by using different methods. These are the methods we covered:
- Using Object.value, indexOf(), and Object.key
- Using a for… in loop
- Using Object.Keys
- Using a type assertation
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com